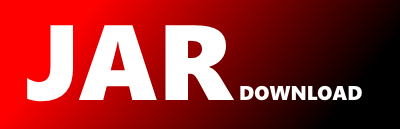
net.finmath.smartcontract.product.xml.Fra Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import javax.xml.datatype.XMLGregorianCalendar;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* A type defining a Forward Rate Agreement (FRA) product.
*
* Java class for Fra complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Fra">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}Product">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BuyerSeller.model"/>
* <sequence>
* <element name="adjustedEffectiveDate" type="{http://www.fpml.org/FpML-5/confirmation}RequiredIdentifierDate"/>
* <element name="adjustedTerminationDate" type="{http://www.w3.org/2001/XMLSchema}date"/>
* </sequence>
* <element name="paymentDate" type="{http://www.fpml.org/FpML-5/confirmation}AdjustableDate"/>
* <element name="fixingDateOffset" type="{http://www.fpml.org/FpML-5/confirmation}RelativeDateOffset"/>
* <element name="dayCountFraction" type="{http://www.fpml.org/FpML-5/confirmation}DayCountFraction"/>
* <element name="calculationPeriodNumberOfDays" type="{http://www.w3.org/2001/XMLSchema}positiveInteger"/>
* <element name="notional" type="{http://www.fpml.org/FpML-5/confirmation}Money"/>
* <element name="fixedRate" type="{http://www.fpml.org/FpML-5/confirmation}IdentifiedRate"/>
* <element name="floatingRateIndex" type="{http://www.fpml.org/FpML-5/confirmation}FloatingRateIndex"/>
* <element name="indexTenor" type="{http://www.fpml.org/FpML-5/confirmation}Period" maxOccurs="unbounded"/>
* <element name="fraDiscounting" type="{http://www.fpml.org/FpML-5/confirmation}FraDiscountingEnum"/>
* <element name="additionalPayment" type="{http://www.fpml.org/FpML-5/confirmation}Payment" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Fra", propOrder = {
"buyerPartyReference",
"buyerAccountReference",
"sellerPartyReference",
"sellerAccountReference",
"adjustedEffectiveDate",
"adjustedTerminationDate",
"paymentDate",
"fixingDateOffset",
"dayCountFraction",
"calculationPeriodNumberOfDays",
"notional",
"fixedRate",
"floatingRateIndex",
"indexTenor",
"fraDiscounting",
"additionalPayment"
})
public class Fra
extends Product
{
@XmlElement(required = true)
protected PartyReference buyerPartyReference;
protected AccountReference buyerAccountReference;
@XmlElement(required = true)
protected PartyReference sellerPartyReference;
protected AccountReference sellerAccountReference;
@XmlElement(required = true)
protected RequiredIdentifierDate adjustedEffectiveDate;
@XmlElement(required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar adjustedTerminationDate;
@XmlElement(required = true)
protected AdjustableDate paymentDate;
@XmlElement(required = true)
protected RelativeDateOffset fixingDateOffset;
@XmlElement(required = true)
protected DayCountFraction dayCountFraction;
@XmlElement(required = true)
@XmlSchemaType(name = "positiveInteger")
protected BigInteger calculationPeriodNumberOfDays;
@XmlElement(required = true)
protected Money notional;
@XmlElement(required = true)
protected IdentifiedRate fixedRate;
@XmlElement(required = true)
protected FloatingRateIndex floatingRateIndex;
@XmlElement(required = true)
protected List indexTenor;
@XmlElement(required = true)
@XmlSchemaType(name = "token")
protected FraDiscountingEnum fraDiscounting;
protected List additionalPayment;
/**
* Gets the value of the buyerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getBuyerPartyReference() {
return buyerPartyReference;
}
/**
* Sets the value of the buyerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setBuyerPartyReference(PartyReference value) {
this.buyerPartyReference = value;
}
/**
* Gets the value of the buyerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getBuyerAccountReference() {
return buyerAccountReference;
}
/**
* Sets the value of the buyerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setBuyerAccountReference(AccountReference value) {
this.buyerAccountReference = value;
}
/**
* Gets the value of the sellerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getSellerPartyReference() {
return sellerPartyReference;
}
/**
* Sets the value of the sellerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setSellerPartyReference(PartyReference value) {
this.sellerPartyReference = value;
}
/**
* Gets the value of the sellerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getSellerAccountReference() {
return sellerAccountReference;
}
/**
* Sets the value of the sellerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setSellerAccountReference(AccountReference value) {
this.sellerAccountReference = value;
}
/**
* Gets the value of the adjustedEffectiveDate property.
*
* @return
* possible object is
* {@link RequiredIdentifierDate }
*
*/
public RequiredIdentifierDate getAdjustedEffectiveDate() {
return adjustedEffectiveDate;
}
/**
* Sets the value of the adjustedEffectiveDate property.
*
* @param value
* allowed object is
* {@link RequiredIdentifierDate }
*
*/
public void setAdjustedEffectiveDate(RequiredIdentifierDate value) {
this.adjustedEffectiveDate = value;
}
/**
* Gets the value of the adjustedTerminationDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getAdjustedTerminationDate() {
return adjustedTerminationDate;
}
/**
* Sets the value of the adjustedTerminationDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setAdjustedTerminationDate(XMLGregorianCalendar value) {
this.adjustedTerminationDate = value;
}
/**
* Gets the value of the paymentDate property.
*
* @return
* possible object is
* {@link AdjustableDate }
*
*/
public AdjustableDate getPaymentDate() {
return paymentDate;
}
/**
* Sets the value of the paymentDate property.
*
* @param value
* allowed object is
* {@link AdjustableDate }
*
*/
public void setPaymentDate(AdjustableDate value) {
this.paymentDate = value;
}
/**
* Gets the value of the fixingDateOffset property.
*
* @return
* possible object is
* {@link RelativeDateOffset }
*
*/
public RelativeDateOffset getFixingDateOffset() {
return fixingDateOffset;
}
/**
* Sets the value of the fixingDateOffset property.
*
* @param value
* allowed object is
* {@link RelativeDateOffset }
*
*/
public void setFixingDateOffset(RelativeDateOffset value) {
this.fixingDateOffset = value;
}
/**
* Gets the value of the dayCountFraction property.
*
* @return
* possible object is
* {@link DayCountFraction }
*
*/
public DayCountFraction getDayCountFraction() {
return dayCountFraction;
}
/**
* Sets the value of the dayCountFraction property.
*
* @param value
* allowed object is
* {@link DayCountFraction }
*
*/
public void setDayCountFraction(DayCountFraction value) {
this.dayCountFraction = value;
}
/**
* Gets the value of the calculationPeriodNumberOfDays property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getCalculationPeriodNumberOfDays() {
return calculationPeriodNumberOfDays;
}
/**
* Sets the value of the calculationPeriodNumberOfDays property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setCalculationPeriodNumberOfDays(BigInteger value) {
this.calculationPeriodNumberOfDays = value;
}
/**
* Gets the value of the notional property.
*
* @return
* possible object is
* {@link Money }
*
*/
public Money getNotional() {
return notional;
}
/**
* Sets the value of the notional property.
*
* @param value
* allowed object is
* {@link Money }
*
*/
public void setNotional(Money value) {
this.notional = value;
}
/**
* Gets the value of the fixedRate property.
*
* @return
* possible object is
* {@link IdentifiedRate }
*
*/
public IdentifiedRate getFixedRate() {
return fixedRate;
}
/**
* Sets the value of the fixedRate property.
*
* @param value
* allowed object is
* {@link IdentifiedRate }
*
*/
public void setFixedRate(IdentifiedRate value) {
this.fixedRate = value;
}
/**
* Gets the value of the floatingRateIndex property.
*
* @return
* possible object is
* {@link FloatingRateIndex }
*
*/
public FloatingRateIndex getFloatingRateIndex() {
return floatingRateIndex;
}
/**
* Sets the value of the floatingRateIndex property.
*
* @param value
* allowed object is
* {@link FloatingRateIndex }
*
*/
public void setFloatingRateIndex(FloatingRateIndex value) {
this.floatingRateIndex = value;
}
/**
* Gets the value of the indexTenor property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the indexTenor property.
*
*
* For example, to add a new item, do as follows:
*
* getIndexTenor().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Period }
*
*
*/
public List getIndexTenor() {
if (indexTenor == null) {
indexTenor = new ArrayList();
}
return this.indexTenor;
}
/**
* Gets the value of the fraDiscounting property.
*
* @return
* possible object is
* {@link FraDiscountingEnum }
*
*/
public FraDiscountingEnum getFraDiscounting() {
return fraDiscounting;
}
/**
* Sets the value of the fraDiscounting property.
*
* @param value
* allowed object is
* {@link FraDiscountingEnum }
*
*/
public void setFraDiscounting(FraDiscountingEnum value) {
this.fraDiscounting = value;
}
/**
* Gets the value of the additionalPayment property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the additionalPayment property.
*
*
* For example, to add a new item, do as follows:
*
* getAdditionalPayment().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Payment }
*
*
*/
public List getAdditionalPayment() {
if (additionalPayment == null) {
additionalPayment = new ArrayList();
}
return this.additionalPayment;
}
}