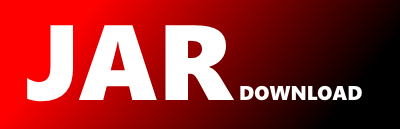
net.finmath.smartcontract.product.xml.FxAccrualForward Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
/**
* The product defines a schedule of expiry and delivery dates which specify
* settlement periods. The product further defines a schedule of fixing (or observation) dates and defines
* regions of spot where the product settlement amounts will accrue. There are n total fixings. One
* accumulates a fixed proportion of Notional (1/n) for every observation date that spot fixes within the
* pre-defined limits of the accrual regions. If spot breaks the limits, the Notional stops accumulating
* during the fixings outside the limits, but continues accruing once spot comes back to the accruing
* region. At expiry, one buys the accrued Notional at the pre-agreed hedge rate. Payout can be cash or
* physical. The variation of this product include: Accrual Forward (European and American), Double Accrual
* Forward (DAF), Boosted Accrual Forward, Fading Forward, Leveraged Accrual Forward, Accrual Forward with
* Collars, etc.
*
*
* Java class for FxAccrualForward complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FxAccrualForward">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}Product">
* <sequence>
* <element name="notionalAmount" type="{http://www.fpml.org/FpML-5/confirmation}NonNegativeAmountSchedule"/>
* <element name="accrual" type="{http://www.fpml.org/FpML-5/confirmation}FxAccrual"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}FxExpiryDateOrSchedule.model"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}FxSettlementDateOrSchedule.model"/>
* <element name="spotRate" type="{http://www.fpml.org/FpML-5/confirmation}PositiveDecimal" minOccurs="0"/>
* <element name="linearPayoffRegion" type="{http://www.fpml.org/FpML-5/confirmation}FxAccrualLinearPayoffRegion" maxOccurs="unbounded"/>
* <element name="averageRate" type="{http://www.fpml.org/FpML-5/confirmation}FxAverageRate" minOccurs="0"/>
* <element name="barrier" type="{http://www.fpml.org/FpML-5/confirmation}FxAccrualBarrier" maxOccurs="unbounded" minOccurs="0"/>
* <element name="additionalPayment" type="{http://www.fpml.org/FpML-5/confirmation}SimplePayment" maxOccurs="unbounded" minOccurs="0"/>
* <element name="cashSettlement" type="{http://www.fpml.org/FpML-5/confirmation}FxCashSettlementSimple" minOccurs="0"/>
* <element name="settlementPeriodSchedule" type="{http://www.fpml.org/FpML-5/confirmation}FxAccrualSettlementPeriodSchedule" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FxAccrualForward", propOrder = {
"notionalAmount",
"accrual",
"expiryDate",
"expirySchedule",
"settlementDate",
"settlementSchedule",
"spotRate",
"linearPayoffRegion",
"averageRate",
"barrier",
"additionalPayment",
"cashSettlement",
"settlementPeriodSchedule"
})
public class FxAccrualForward
extends Product
{
@XmlElement(required = true)
protected NonNegativeAmountSchedule notionalAmount;
@XmlElement(required = true)
protected FxAccrual accrual;
protected FxExpiryDate expiryDate;
protected FxExpirySchedule expirySchedule;
protected FxAdjustedDateAndDateAdjustments settlementDate;
protected FxSettlementSchedule settlementSchedule;
protected BigDecimal spotRate;
@XmlElement(required = true)
protected List linearPayoffRegion;
protected FxAverageRate averageRate;
protected List barrier;
protected List additionalPayment;
protected FxCashSettlementSimple cashSettlement;
protected FxAccrualSettlementPeriodSchedule settlementPeriodSchedule;
/**
* Gets the value of the notionalAmount property.
*
* @return
* possible object is
* {@link NonNegativeAmountSchedule }
*
*/
public NonNegativeAmountSchedule getNotionalAmount() {
return notionalAmount;
}
/**
* Sets the value of the notionalAmount property.
*
* @param value
* allowed object is
* {@link NonNegativeAmountSchedule }
*
*/
public void setNotionalAmount(NonNegativeAmountSchedule value) {
this.notionalAmount = value;
}
/**
* Gets the value of the accrual property.
*
* @return
* possible object is
* {@link FxAccrual }
*
*/
public FxAccrual getAccrual() {
return accrual;
}
/**
* Sets the value of the accrual property.
*
* @param value
* allowed object is
* {@link FxAccrual }
*
*/
public void setAccrual(FxAccrual value) {
this.accrual = value;
}
/**
* Gets the value of the expiryDate property.
*
* @return
* possible object is
* {@link FxExpiryDate }
*
*/
public FxExpiryDate getExpiryDate() {
return expiryDate;
}
/**
* Sets the value of the expiryDate property.
*
* @param value
* allowed object is
* {@link FxExpiryDate }
*
*/
public void setExpiryDate(FxExpiryDate value) {
this.expiryDate = value;
}
/**
* Gets the value of the expirySchedule property.
*
* @return
* possible object is
* {@link FxExpirySchedule }
*
*/
public FxExpirySchedule getExpirySchedule() {
return expirySchedule;
}
/**
* Sets the value of the expirySchedule property.
*
* @param value
* allowed object is
* {@link FxExpirySchedule }
*
*/
public void setExpirySchedule(FxExpirySchedule value) {
this.expirySchedule = value;
}
/**
* Gets the value of the settlementDate property.
*
* @return
* possible object is
* {@link FxAdjustedDateAndDateAdjustments }
*
*/
public FxAdjustedDateAndDateAdjustments getSettlementDate() {
return settlementDate;
}
/**
* Sets the value of the settlementDate property.
*
* @param value
* allowed object is
* {@link FxAdjustedDateAndDateAdjustments }
*
*/
public void setSettlementDate(FxAdjustedDateAndDateAdjustments value) {
this.settlementDate = value;
}
/**
* Gets the value of the settlementSchedule property.
*
* @return
* possible object is
* {@link FxSettlementSchedule }
*
*/
public FxSettlementSchedule getSettlementSchedule() {
return settlementSchedule;
}
/**
* Sets the value of the settlementSchedule property.
*
* @param value
* allowed object is
* {@link FxSettlementSchedule }
*
*/
public void setSettlementSchedule(FxSettlementSchedule value) {
this.settlementSchedule = value;
}
/**
* Gets the value of the spotRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSpotRate() {
return spotRate;
}
/**
* Sets the value of the spotRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSpotRate(BigDecimal value) {
this.spotRate = value;
}
/**
* Gets the value of the linearPayoffRegion property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the linearPayoffRegion property.
*
*
* For example, to add a new item, do as follows:
*
* getLinearPayoffRegion().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FxAccrualLinearPayoffRegion }
*
*
*/
public List getLinearPayoffRegion() {
if (linearPayoffRegion == null) {
linearPayoffRegion = new ArrayList();
}
return this.linearPayoffRegion;
}
/**
* Gets the value of the averageRate property.
*
* @return
* possible object is
* {@link FxAverageRate }
*
*/
public FxAverageRate getAverageRate() {
return averageRate;
}
/**
* Sets the value of the averageRate property.
*
* @param value
* allowed object is
* {@link FxAverageRate }
*
*/
public void setAverageRate(FxAverageRate value) {
this.averageRate = value;
}
/**
* Gets the value of the barrier property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the barrier property.
*
*
* For example, to add a new item, do as follows:
*
* getBarrier().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FxAccrualBarrier }
*
*
*/
public List getBarrier() {
if (barrier == null) {
barrier = new ArrayList();
}
return this.barrier;
}
/**
* Gets the value of the additionalPayment property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the additionalPayment property.
*
*
* For example, to add a new item, do as follows:
*
* getAdditionalPayment().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link SimplePayment }
*
*
*/
public List getAdditionalPayment() {
if (additionalPayment == null) {
additionalPayment = new ArrayList();
}
return this.additionalPayment;
}
/**
* Gets the value of the cashSettlement property.
*
* @return
* possible object is
* {@link FxCashSettlementSimple }
*
*/
public FxCashSettlementSimple getCashSettlement() {
return cashSettlement;
}
/**
* Sets the value of the cashSettlement property.
*
* @param value
* allowed object is
* {@link FxCashSettlementSimple }
*
*/
public void setCashSettlement(FxCashSettlementSimple value) {
this.cashSettlement = value;
}
/**
* Gets the value of the settlementPeriodSchedule property.
*
* @return
* possible object is
* {@link FxAccrualSettlementPeriodSchedule }
*
*/
public FxAccrualSettlementPeriodSchedule getSettlementPeriodSchedule() {
return settlementPeriodSchedule;
}
/**
* Sets the value of the settlementPeriodSchedule property.
*
* @param value
* allowed object is
* {@link FxAccrualSettlementPeriodSchedule }
*
*/
public void setSettlementPeriodSchedule(FxAccrualSettlementPeriodSchedule value) {
this.settlementPeriodSchedule = value;
}
}