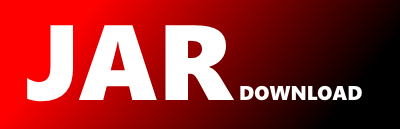
net.finmath.smartcontract.product.xml.PackageInformation Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlType;
/**
* A type defining additional information that may be recorded against a
* package of trades.
*
*
* Java class for PackageInformation complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PackageInformation">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="relatedParty" type="{http://www.fpml.org/FpML-5/confirmation}RelatedParty" maxOccurs="unbounded" minOccurs="0"/>
* <element name="category" type="{http://www.fpml.org/FpML-5/confirmation}TradeCategory" maxOccurs="unbounded" minOccurs="0"/>
* <element name="executionDateTime" type="{http://www.fpml.org/FpML-5/confirmation}ExecutionDateTime" minOccurs="0"/>
* <element name="timestamps" type="{http://www.fpml.org/FpML-5/confirmation}TradeProcessingTimestamps" minOccurs="0"/>
* <element name="intentToAllocate" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="allocationStatus" type="{http://www.fpml.org/FpML-5/confirmation}AllocationReportingStatus" minOccurs="0"/>
* <element name="intentToClear" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="clearingStatus" type="{http://www.fpml.org/FpML-5/confirmation}ClearingStatusValue" minOccurs="0"/>
* <element name="executionVenueType" type="{http://www.fpml.org/FpML-5/confirmation}ExecutionVenueType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PackageInformation", propOrder = {
"relatedParty",
"category",
"executionDateTime",
"timestamps",
"intentToAllocate",
"allocationStatus",
"intentToClear",
"clearingStatus",
"executionVenueType"
})
public class PackageInformation {
protected List relatedParty;
protected List category;
protected ExecutionDateTime executionDateTime;
protected TradeProcessingTimestamps timestamps;
protected Boolean intentToAllocate;
protected AllocationReportingStatus allocationStatus;
protected Boolean intentToClear;
protected ClearingStatusValue clearingStatus;
protected ExecutionVenueType executionVenueType;
/**
* Gets the value of the relatedParty property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the relatedParty property.
*
*
* For example, to add a new item, do as follows:
*
* getRelatedParty().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelatedParty }
*
*
*/
public List getRelatedParty() {
if (relatedParty == null) {
relatedParty = new ArrayList();
}
return this.relatedParty;
}
/**
* Gets the value of the category property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the category property.
*
*
* For example, to add a new item, do as follows:
*
* getCategory().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link TradeCategory }
*
*
*/
public List getCategory() {
if (category == null) {
category = new ArrayList();
}
return this.category;
}
/**
* Gets the value of the executionDateTime property.
*
* @return
* possible object is
* {@link ExecutionDateTime }
*
*/
public ExecutionDateTime getExecutionDateTime() {
return executionDateTime;
}
/**
* Sets the value of the executionDateTime property.
*
* @param value
* allowed object is
* {@link ExecutionDateTime }
*
*/
public void setExecutionDateTime(ExecutionDateTime value) {
this.executionDateTime = value;
}
/**
* Gets the value of the timestamps property.
*
* @return
* possible object is
* {@link TradeProcessingTimestamps }
*
*/
public TradeProcessingTimestamps getTimestamps() {
return timestamps;
}
/**
* Sets the value of the timestamps property.
*
* @param value
* allowed object is
* {@link TradeProcessingTimestamps }
*
*/
public void setTimestamps(TradeProcessingTimestamps value) {
this.timestamps = value;
}
/**
* Gets the value of the intentToAllocate property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIntentToAllocate() {
return intentToAllocate;
}
/**
* Sets the value of the intentToAllocate property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIntentToAllocate(Boolean value) {
this.intentToAllocate = value;
}
/**
* Gets the value of the allocationStatus property.
*
* @return
* possible object is
* {@link AllocationReportingStatus }
*
*/
public AllocationReportingStatus getAllocationStatus() {
return allocationStatus;
}
/**
* Sets the value of the allocationStatus property.
*
* @param value
* allowed object is
* {@link AllocationReportingStatus }
*
*/
public void setAllocationStatus(AllocationReportingStatus value) {
this.allocationStatus = value;
}
/**
* Gets the value of the intentToClear property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIntentToClear() {
return intentToClear;
}
/**
* Sets the value of the intentToClear property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIntentToClear(Boolean value) {
this.intentToClear = value;
}
/**
* Gets the value of the clearingStatus property.
*
* @return
* possible object is
* {@link ClearingStatusValue }
*
*/
public ClearingStatusValue getClearingStatus() {
return clearingStatus;
}
/**
* Sets the value of the clearingStatus property.
*
* @param value
* allowed object is
* {@link ClearingStatusValue }
*
*/
public void setClearingStatus(ClearingStatusValue value) {
this.clearingStatus = value;
}
/**
* Gets the value of the executionVenueType property.
*
* @return
* possible object is
* {@link ExecutionVenueType }
*
*/
public ExecutionVenueType getExecutionVenueType() {
return executionVenueType;
}
/**
* Sets the value of the executionVenueType property.
*
* @param value
* allowed object is
* {@link ExecutionVenueType }
*
*/
public void setExecutionVenueType(ExecutionVenueType value) {
this.executionVenueType = value;
}
}