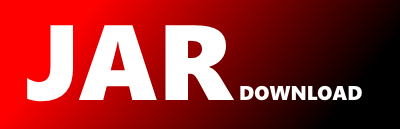
net.finmath.smartcontract.product.xml.Repo Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlElements;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* A Repo, modeled as an FpML:Product. Note: this Repo model is a candidate
* model for further industry input.
*
*
* Java class for Repo complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Repo">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}Product">
* <sequence>
* <choice>
* <element name="fixedRateSchedule" type="{http://www.fpml.org/FpML-5/confirmation}Schedule"/>
* <element name="floatingRateCalculation" type="{http://www.fpml.org/FpML-5/confirmation}FloatingRateCalculation"/>
* </choice>
* <element name="dayCountFraction" type="{http://www.fpml.org/FpML-5/confirmation}DayCountFraction"/>
* <choice>
* <element name="duration" type="{http://www.fpml.org/FpML-5/confirmation}RepoDurationEnum"/>
* <sequence>
* <element name="callingParty" type="{http://www.fpml.org/FpML-5/confirmation}CallingPartyEnum"/>
* <element name="callDate" type="{http://www.fpml.org/FpML-5/confirmation}AdjustableOrRelativeDate" minOccurs="0"/>
* <choice minOccurs="0">
* <element name="noticePeriod" type="{http://www.fpml.org/FpML-5/confirmation}AdjustableOffset"/>
* <element name="partyNoticePeriod" type="{http://www.fpml.org/FpML-5/confirmation}PartyNoticePeriod" maxOccurs="2"/>
* </choice>
* </sequence>
* </choice>
* <element name="initialMargin" type="{http://www.fpml.org/FpML-5/confirmation}InitialMargin" minOccurs="0"/>
* <element name="nearLeg" type="{http://www.fpml.org/FpML-5/confirmation}RepoNearLeg"/>
* <element name="farLeg" type="{http://www.fpml.org/FpML-5/confirmation}RepoFarLeg" minOccurs="0"/>
* <choice>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BondEquity.model" maxOccurs="unbounded"/>
* <element name="triParty" type="{http://www.fpml.org/FpML-5/confirmation}TriParty"/>
* </choice>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Repo", propOrder = {
"fixedRateSchedule",
"floatingRateCalculation",
"dayCountFraction",
"duration",
"callingParty",
"callDate",
"noticePeriod",
"partyNoticePeriod",
"initialMargin",
"nearLeg",
"farLeg",
"bondEquityModel",
"triParty"
})
public class Repo
extends Product
{
protected Schedule fixedRateSchedule;
protected FloatingRateCalculation floatingRateCalculation;
@XmlElement(required = true)
protected DayCountFraction dayCountFraction;
@XmlSchemaType(name = "token")
protected RepoDurationEnum duration;
@XmlSchemaType(name = "token")
protected CallingPartyEnum callingParty;
protected AdjustableOrRelativeDate callDate;
protected AdjustableOffset noticePeriod;
protected List partyNoticePeriod;
protected InitialMargin initialMargin;
@XmlElement(required = true)
protected RepoNearLeg nearLeg;
protected RepoFarLeg farLeg;
@XmlElements({
@XmlElement(name = "bond", type = Bond.class),
@XmlElement(name = "convertibleBond", type = ConvertibleBond.class),
@XmlElement(name = "equity", type = EquityAsset.class)
})
protected List bondEquityModel;
protected TriParty triParty;
/**
* Gets the value of the fixedRateSchedule property.
*
* @return
* possible object is
* {@link Schedule }
*
*/
public Schedule getFixedRateSchedule() {
return fixedRateSchedule;
}
/**
* Sets the value of the fixedRateSchedule property.
*
* @param value
* allowed object is
* {@link Schedule }
*
*/
public void setFixedRateSchedule(Schedule value) {
this.fixedRateSchedule = value;
}
/**
* Gets the value of the floatingRateCalculation property.
*
* @return
* possible object is
* {@link FloatingRateCalculation }
*
*/
public FloatingRateCalculation getFloatingRateCalculation() {
return floatingRateCalculation;
}
/**
* Sets the value of the floatingRateCalculation property.
*
* @param value
* allowed object is
* {@link FloatingRateCalculation }
*
*/
public void setFloatingRateCalculation(FloatingRateCalculation value) {
this.floatingRateCalculation = value;
}
/**
* Gets the value of the dayCountFraction property.
*
* @return
* possible object is
* {@link DayCountFraction }
*
*/
public DayCountFraction getDayCountFraction() {
return dayCountFraction;
}
/**
* Sets the value of the dayCountFraction property.
*
* @param value
* allowed object is
* {@link DayCountFraction }
*
*/
public void setDayCountFraction(DayCountFraction value) {
this.dayCountFraction = value;
}
/**
* Gets the value of the duration property.
*
* @return
* possible object is
* {@link RepoDurationEnum }
*
*/
public RepoDurationEnum getDuration() {
return duration;
}
/**
* Sets the value of the duration property.
*
* @param value
* allowed object is
* {@link RepoDurationEnum }
*
*/
public void setDuration(RepoDurationEnum value) {
this.duration = value;
}
/**
* Gets the value of the callingParty property.
*
* @return
* possible object is
* {@link CallingPartyEnum }
*
*/
public CallingPartyEnum getCallingParty() {
return callingParty;
}
/**
* Sets the value of the callingParty property.
*
* @param value
* allowed object is
* {@link CallingPartyEnum }
*
*/
public void setCallingParty(CallingPartyEnum value) {
this.callingParty = value;
}
/**
* Gets the value of the callDate property.
*
* @return
* possible object is
* {@link AdjustableOrRelativeDate }
*
*/
public AdjustableOrRelativeDate getCallDate() {
return callDate;
}
/**
* Sets the value of the callDate property.
*
* @param value
* allowed object is
* {@link AdjustableOrRelativeDate }
*
*/
public void setCallDate(AdjustableOrRelativeDate value) {
this.callDate = value;
}
/**
* Gets the value of the noticePeriod property.
*
* @return
* possible object is
* {@link AdjustableOffset }
*
*/
public AdjustableOffset getNoticePeriod() {
return noticePeriod;
}
/**
* Sets the value of the noticePeriod property.
*
* @param value
* allowed object is
* {@link AdjustableOffset }
*
*/
public void setNoticePeriod(AdjustableOffset value) {
this.noticePeriod = value;
}
/**
* Gets the value of the partyNoticePeriod property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the partyNoticePeriod property.
*
*
* For example, to add a new item, do as follows:
*
* getPartyNoticePeriod().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyNoticePeriod }
*
*
*/
public List getPartyNoticePeriod() {
if (partyNoticePeriod == null) {
partyNoticePeriod = new ArrayList();
}
return this.partyNoticePeriod;
}
/**
* Gets the value of the initialMargin property.
*
* @return
* possible object is
* {@link InitialMargin }
*
*/
public InitialMargin getInitialMargin() {
return initialMargin;
}
/**
* Sets the value of the initialMargin property.
*
* @param value
* allowed object is
* {@link InitialMargin }
*
*/
public void setInitialMargin(InitialMargin value) {
this.initialMargin = value;
}
/**
* Gets the value of the nearLeg property.
*
* @return
* possible object is
* {@link RepoNearLeg }
*
*/
public RepoNearLeg getNearLeg() {
return nearLeg;
}
/**
* Sets the value of the nearLeg property.
*
* @param value
* allowed object is
* {@link RepoNearLeg }
*
*/
public void setNearLeg(RepoNearLeg value) {
this.nearLeg = value;
}
/**
* Gets the value of the farLeg property.
*
* @return
* possible object is
* {@link RepoFarLeg }
*
*/
public RepoFarLeg getFarLeg() {
return farLeg;
}
/**
* Sets the value of the farLeg property.
*
* @param value
* allowed object is
* {@link RepoFarLeg }
*
*/
public void setFarLeg(RepoFarLeg value) {
this.farLeg = value;
}
/**
* A list of the financial instruments that the repo
* contract may reference.
* Gets the value of the bondEquityModel property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the bondEquityModel property.
*
*
* For example, to add a new item, do as follows:
*
* getBondEquityModel().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Bond }
* {@link ConvertibleBond }
* {@link EquityAsset }
*
*
*/
public List getBondEquityModel() {
if (bondEquityModel == null) {
bondEquityModel = new ArrayList();
}
return this.bondEquityModel;
}
/**
* Gets the value of the triParty property.
*
* @return
* possible object is
* {@link TriParty }
*
*/
public TriParty getTriParty() {
return triParty;
}
/**
* Sets the value of the triParty property.
*
* @param value
* allowed object is
* {@link TriParty }
*
*/
public void setTriParty(TriParty value) {
this.triParty = value;
}
}