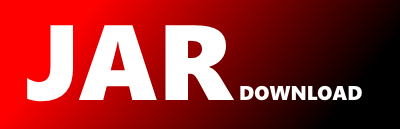
net.finmath.smartcontract.product.xml.Resource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finmath-smart-derivative-contract Show documentation
Show all versions of finmath-smart-derivative-contract Show documentation
Project to support the implementation a of smart derivative contract.
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.math.BigDecimal;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.HexBinaryAdapter;
import jakarta.xml.bind.annotation.adapters.NormalizedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Describes the resource that contains the media representation of a business
* event (i.e used for stating the Publicly Available Information). For example, can describe a file or a
* URL that represents the event. This type is an extended version of a type defined by RIXML
* (www.rixml.org).
*
*
* Java class for Resource complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Resource">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="resourceId" type="{http://www.fpml.org/FpML-5/confirmation}ResourceId"/>
* <element name="resourceType" type="{http://www.fpml.org/FpML-5/confirmation}ResourceType" minOccurs="0"/>
* <element name="language" type="{http://www.fpml.org/FpML-5/confirmation}Language" minOccurs="0"/>
* <element name="sizeInBytes" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="length" type="{http://www.fpml.org/FpML-5/confirmation}ResourceLength" minOccurs="0"/>
* <element name="mimeType" type="{http://www.fpml.org/FpML-5/confirmation}MimeType"/>
* <element name="name" type="{http://www.fpml.org/FpML-5/confirmation}NormalizedString" minOccurs="0"/>
* <element name="comments" type="{http://www.fpml.org/FpML-5/confirmation}String" minOccurs="0"/>
* <choice minOccurs="0">
* <element name="string" type="{http://www.fpml.org/FpML-5/confirmation}String"/>
* <element name="hexadecimalBinary" type="{http://www.w3.org/2001/XMLSchema}hexBinary"/>
* <element name="base64Binary" type="{http://www.w3.org/2001/XMLSchema}base64Binary"/>
* <element name="url" type="{http://www.fpml.org/FpML-5/confirmation}NonEmptyURI"/>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Resource", propOrder = {
"resourceId",
"resourceType",
"language",
"sizeInBytes",
"length",
"mimeType",
"name",
"comments",
"string",
"hexadecimalBinary",
"base64Binary",
"url"
})
public class Resource {
@XmlElement(required = true)
protected ResourceId resourceId;
protected ResourceType resourceType;
protected Language language;
protected BigDecimal sizeInBytes;
protected ResourceLength length;
@XmlElement(required = true)
protected MimeType mimeType;
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String name;
protected String comments;
protected String string;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(HexBinaryAdapter.class)
@XmlSchemaType(name = "hexBinary")
protected byte[] hexadecimalBinary;
protected byte[] base64Binary;
@XmlSchemaType(name = "anyURI")
protected String url;
/**
* Gets the value of the resourceId property.
*
* @return
* possible object is
* {@link ResourceId }
*
*/
public ResourceId getResourceId() {
return resourceId;
}
/**
* Sets the value of the resourceId property.
*
* @param value
* allowed object is
* {@link ResourceId }
*
*/
public void setResourceId(ResourceId value) {
this.resourceId = value;
}
/**
* Gets the value of the resourceType property.
*
* @return
* possible object is
* {@link ResourceType }
*
*/
public ResourceType getResourceType() {
return resourceType;
}
/**
* Sets the value of the resourceType property.
*
* @param value
* allowed object is
* {@link ResourceType }
*
*/
public void setResourceType(ResourceType value) {
this.resourceType = value;
}
/**
* Gets the value of the language property.
*
* @return
* possible object is
* {@link Language }
*
*/
public Language getLanguage() {
return language;
}
/**
* Sets the value of the language property.
*
* @param value
* allowed object is
* {@link Language }
*
*/
public void setLanguage(Language value) {
this.language = value;
}
/**
* Gets the value of the sizeInBytes property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSizeInBytes() {
return sizeInBytes;
}
/**
* Sets the value of the sizeInBytes property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSizeInBytes(BigDecimal value) {
this.sizeInBytes = value;
}
/**
* Gets the value of the length property.
*
* @return
* possible object is
* {@link ResourceLength }
*
*/
public ResourceLength getLength() {
return length;
}
/**
* Sets the value of the length property.
*
* @param value
* allowed object is
* {@link ResourceLength }
*
*/
public void setLength(ResourceLength value) {
this.length = value;
}
/**
* Gets the value of the mimeType property.
*
* @return
* possible object is
* {@link MimeType }
*
*/
public MimeType getMimeType() {
return mimeType;
}
/**
* Sets the value of the mimeType property.
*
* @param value
* allowed object is
* {@link MimeType }
*
*/
public void setMimeType(MimeType value) {
this.mimeType = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the comments property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComments() {
return comments;
}
/**
* Sets the value of the comments property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComments(String value) {
this.comments = value;
}
/**
* Gets the value of the string property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getString() {
return string;
}
/**
* Sets the value of the string property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setString(String value) {
this.string = value;
}
/**
* Gets the value of the hexadecimalBinary property.
*
* @return
* possible object is
* {@link String }
*
*/
public byte[] getHexadecimalBinary() {
return hexadecimalBinary;
}
/**
* Sets the value of the hexadecimalBinary property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHexadecimalBinary(byte[] value) {
this.hexadecimalBinary = value;
}
/**
* Gets the value of the base64Binary property.
*
* @return
* possible object is
* byte[]
*/
public byte[] getBase64Binary() {
return base64Binary;
}
/**
* Sets the value of the base64Binary property.
*
* @param value
* allowed object is
* byte[]
*/
public void setBase64Binary(byte[] value) {
this.base64Binary = value;
}
/**
* Gets the value of the url property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUrl() {
return url;
}
/**
* Sets the value of the url property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUrl(String value) {
this.url = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy