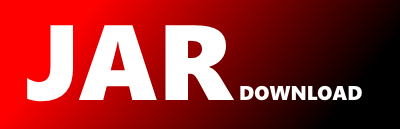
net.finmath.smartcontract.product.xml.TriggerRateObservation Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.05 at 10:53:57 AM CET
//
package net.finmath.smartcontract.product.xml;
import java.math.BigDecimal;
import javax.xml.datatype.XMLGregorianCalendar;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for TriggerRateObservation complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="TriggerRateObservation">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="observationDate" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="observationTime" type="{http://www.fpml.org/FpML-5/confirmation}BusinessCenterTime" minOccurs="0"/>
* <element name="informationSource" type="{http://www.fpml.org/FpML-5/confirmation}InformationSource" minOccurs="0"/>
* <choice>
* <sequence>
* <element name="triggerRate" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element name="quotedCurrencyPair" type="{http://www.fpml.org/FpML-5/confirmation}QuotedCurrencyPair"/>
* <element name="observedRate" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* </sequence>
* <sequence>
* <element name="triggerPrice" type="{http://www.fpml.org/FpML-5/confirmation}PositiveMoney"/>
* <element name="observedPrice" type="{http://www.fpml.org/FpML-5/confirmation}PositiveMoney" minOccurs="0"/>
* </sequence>
* </choice>
* <element name="triggerCondition" type="{http://www.fpml.org/FpML-5/confirmation}TriggerConditionEnum"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TriggerRateObservation", propOrder = {
"observationDate",
"observationTime",
"informationSource",
"triggerRate",
"quotedCurrencyPair",
"observedRate",
"triggerPrice",
"observedPrice",
"triggerCondition"
})
@XmlSeeAlso({
KnockOutRateObservation.class,
TouchRateObservation.class
})
public class TriggerRateObservation {
@XmlElement(required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar observationDate;
protected BusinessCenterTime observationTime;
protected InformationSource informationSource;
protected BigDecimal triggerRate;
protected QuotedCurrencyPair quotedCurrencyPair;
protected BigDecimal observedRate;
protected PositiveMoney triggerPrice;
protected PositiveMoney observedPrice;
@XmlElement(required = true)
@XmlSchemaType(name = "token")
protected TriggerConditionEnum triggerCondition;
/**
* Gets the value of the observationDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getObservationDate() {
return observationDate;
}
/**
* Sets the value of the observationDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setObservationDate(XMLGregorianCalendar value) {
this.observationDate = value;
}
/**
* Gets the value of the observationTime property.
*
* @return
* possible object is
* {@link BusinessCenterTime }
*
*/
public BusinessCenterTime getObservationTime() {
return observationTime;
}
/**
* Sets the value of the observationTime property.
*
* @param value
* allowed object is
* {@link BusinessCenterTime }
*
*/
public void setObservationTime(BusinessCenterTime value) {
this.observationTime = value;
}
/**
* Gets the value of the informationSource property.
*
* @return
* possible object is
* {@link InformationSource }
*
*/
public InformationSource getInformationSource() {
return informationSource;
}
/**
* Sets the value of the informationSource property.
*
* @param value
* allowed object is
* {@link InformationSource }
*
*/
public void setInformationSource(InformationSource value) {
this.informationSource = value;
}
/**
* Gets the value of the triggerRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTriggerRate() {
return triggerRate;
}
/**
* Sets the value of the triggerRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTriggerRate(BigDecimal value) {
this.triggerRate = value;
}
/**
* Gets the value of the quotedCurrencyPair property.
*
* @return
* possible object is
* {@link QuotedCurrencyPair }
*
*/
public QuotedCurrencyPair getQuotedCurrencyPair() {
return quotedCurrencyPair;
}
/**
* Sets the value of the quotedCurrencyPair property.
*
* @param value
* allowed object is
* {@link QuotedCurrencyPair }
*
*/
public void setQuotedCurrencyPair(QuotedCurrencyPair value) {
this.quotedCurrencyPair = value;
}
/**
* Gets the value of the observedRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getObservedRate() {
return observedRate;
}
/**
* Sets the value of the observedRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setObservedRate(BigDecimal value) {
this.observedRate = value;
}
/**
* Gets the value of the triggerPrice property.
*
* @return
* possible object is
* {@link PositiveMoney }
*
*/
public PositiveMoney getTriggerPrice() {
return triggerPrice;
}
/**
* Sets the value of the triggerPrice property.
*
* @param value
* allowed object is
* {@link PositiveMoney }
*
*/
public void setTriggerPrice(PositiveMoney value) {
this.triggerPrice = value;
}
/**
* Gets the value of the observedPrice property.
*
* @return
* possible object is
* {@link PositiveMoney }
*
*/
public PositiveMoney getObservedPrice() {
return observedPrice;
}
/**
* Sets the value of the observedPrice property.
*
* @param value
* allowed object is
* {@link PositiveMoney }
*
*/
public void setObservedPrice(PositiveMoney value) {
this.observedPrice = value;
}
/**
* Gets the value of the triggerCondition property.
*
* @return
* possible object is
* {@link TriggerConditionEnum }
*
*/
public TriggerConditionEnum getTriggerCondition() {
return triggerCondition;
}
/**
* Sets the value of the triggerCondition property.
*
* @param value
* allowed object is
* {@link TriggerConditionEnum }
*
*/
public void setTriggerCondition(TriggerConditionEnum value) {
this.triggerCondition = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy