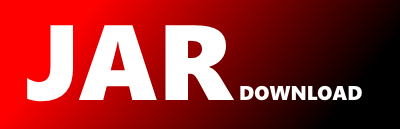
net.finmath.smartcontract.valuation.oracle.SmartDerivativeContractSettlementOracle Maven / Gradle / Ivy
Show all versions of finmath-smart-derivative-contract Show documentation
/*
* (c) Copyright Christian P. Fries, Germany. Contact: [email protected].
*
* Created on 7 Oct 2018
*/
package net.finmath.smartcontract.valuation.oracle;
import java.math.BigDecimal;
import java.time.LocalDateTime;
import java.util.Map;
import java.util.stream.Collectors;
/**
* The margin agreement of a smart derivative contract.
* The agreement consists of
*
* - a valuation oracle, implementing
net.finmath.smartcontract.oracle.ValuationOracle
*
*
* The accrual of the collateral is assumed to e consistent with the valuation, hence, the accrued collateral
* can be determined from calling getValue(marginPeriodEnd, marginPeriodStart)
.
*
* @author Christian Fries
* @see ValuationOracle
*/
public class SmartDerivativeContractSettlementOracle {
private final ValuationOracle derivativeValuationOracle;
public SmartDerivativeContractSettlementOracle(final ValuationOracle derivativeValuationOracle) {
super();
this.derivativeValuationOracle = derivativeValuationOracle;
}
/**
* Get the margin of the contract based on the valuation oracles.
*
* @param marginPeriodStart Period start time of the margin period.
* @param marginPeriodEnd Period end time of the margin period.
* @return The margin.
*/
public Map getMargin(final LocalDateTime marginPeriodStart, final LocalDateTime marginPeriodEnd) {
final Map valueDerivativeCurrent = derivativeValuationOracle.getValues(marginPeriodEnd, marginPeriodEnd);
final Map valueDerivativePrevious = derivativeValuationOracle.getValues(marginPeriodEnd, marginPeriodStart);
//
Map margin = valueDerivativeCurrent.keySet().stream().collect(Collectors.toMap(
key -> key,
key -> valueDerivativeCurrent.get(key).subtract(valueDerivativePrevious.get(key))
));
return margin;
}
}