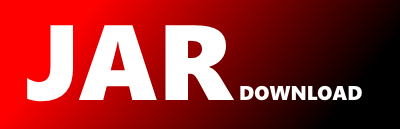
net.flexmojos.oss.plugin.compiler.AsdocMojo Maven / Gradle / Ivy
/**
* Flexmojos is a set of maven goals to allow maven users to compile, optimize and test Flex SWF, Flex SWC, Air SWF and Air SWC.
* Copyright (C) 2008-2012 Marvin Froeder
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package net.flexmojos.oss.plugin.compiler;
import static ch.lambdaj.Lambda.filter;
import static org.hamcrest.CoreMatchers.allOf;
import static org.hamcrest.CoreMatchers.not;
import static net.flexmojos.oss.matcher.artifact.ArtifactMatcher.scope;
import static net.flexmojos.oss.matcher.artifact.ArtifactMatcher.type;
import static net.flexmojos.oss.plugin.common.FlexExtension.AIR;
import static net.flexmojos.oss.plugin.common.FlexExtension.SWC;
import static net.flexmojos.oss.plugin.common.FlexExtension.SWF;
import static net.flexmojos.oss.plugin.common.FlexScopes.TEST;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.Mojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.DefaultProjectBuildingRequest;
import org.apache.maven.project.MavenProject;
import org.apache.maven.project.ProjectBuilder;
import org.apache.maven.project.ProjectBuildingException;
import org.apache.maven.project.ProjectBuildingRequest;
import org.codehaus.plexus.archiver.Archiver;
import org.codehaus.plexus.archiver.UnArchiver;
import org.hamcrest.Matcher;
import net.flexmojos.oss.compatibilitykit.FlexCompatibility;
import net.flexmojos.oss.compiler.IASDocConfiguration;
import net.flexmojos.oss.compiler.IPackagesConfiguration;
import net.flexmojos.oss.compiler.IRuntimeSharedLibraryPath;
import net.flexmojos.oss.compiler.command.Result;
import net.flexmojos.oss.plugin.compiler.attributes.MavenRuntimeException;
import net.flexmojos.oss.plugin.compiler.attributes.SimplifiablePattern;
import net.flexmojos.oss.plugin.utilities.MavenUtils;
import net.flexmojos.oss.util.OSUtils;
import net.flexmojos.oss.util.PathUtil;
/**
*
* Goal which generates documentation from the ActionScript sources.
*
*
* @author Marvin Herman Froeder ([email protected])
* @since 1.0
* @goal asdoc
* @requiresDependencyResolution compile
* @phase process-sources
* @threadSafe
*/
public class AsdocMojo
extends AbstractFlexCompilerMojo
implements IASDocConfiguration, IPackagesConfiguration, Mojo
{
/**
* If true, will treat multi-modules projects as only one project otherwise will generate Asdoc per project
*
* @parameter default-value="false" expression="${flex.asdoc.aggregate}"
*/
private boolean aggregate;
/**
* @parameter expression="${project.build.directory}/asdoc"
* @readonly
* @required
*/
private File asdocOutputDirectory;
/**
* If true, bundles the asdoc documentation for main code into a zip using the standard Asdoc Tool.
*
* @parameter default-value="true" expression="${flex.asdoc.attach}"
*/
private boolean attach;
/**
* Specifies whether to include date with footer
*
* Equivalent to -date-in-footer
*
*
* @parameter expression="${flex.dateInFooter}"
*/
private Boolean dateInFooter;
/**
* Automatically document all declared namespaces
*
* @parameter default-value="false" expression="${flex.docAllNamespaces}"
*/
private boolean docAllNamespaces;
/**
* List of classes to include in the documentation
*
* Equivalent to -doc-classes
*
* Usage:
*
*
* <docClasses>
* <includes>
* <include>com/mycompany/*</include>
* </includes>
* <excludes>
* <exclude>com/mycompany/ui/*</exclude>
* </excludes>
* </docClasses>
*
*
* @parameter
*/
private SimplifiablePattern docClasses;
/**
* List of namespaces to include in the documentation
*
* Equivalent to -doc-namespaces
*
* Usage:
*
*
* <docNamespaces>
* <namespace>http://mynamespace.com</namespace>
* </docNamespaces>
*
*
* @parameter
*/
private List docNamespaces;
/**
* List of source file to include in the documentation
*
* Equivalent to -doc-sources
*
* Usage:
*
*
* <docSources>
* <docSource>${project.build.sourceDirectory}</docSource>
* </docSources>
*
*
* @parameter
*/
private File[] docSources;
/**
* Path to look for the example files
*
* Equivalent to -examples-path
*
*
* @parameter expression="${flex.examplesPath}"
*/
private File examplesPath;
/**
* Boolean specifying whether to exclude dependencies
*
* Equivalent to -exclude-dependencies
*
*
* @parameter expression="${flex.excludeDependencies}"
*/
private Boolean excludeDependencies;
/**
* Footer string to be displayed in the documentation
*
* Equivalent to -footer
*
*
* @parameter default-value="Generated by Flexmojos" expression="${flex.footer}"
*/
private String footer;
/**
* DOCME undocumented by adobe
*
* Equivalent to -include-all-for-asdoc
*
* include-all-only is only for internal use
*
* @parameter expression="${flex.includeAllForAsdoc}"
*/
private Boolean includeAllForAsdoc;
/**
* If true, manifest entries with lookupOnly=true are included in SWC catalog
*
* Equivalent to -include-lookup-only
*
*
* @parameter expression="${flex.includeLookupOnly}"
*/
private Boolean includeLookupOnly;
/**
* DOCME undocumented by adobe
*
* Equivalent to -keep-xml
*
*
* @parameter expression="${flex.keepXml}"
*/
private Boolean keepXml;
/**
* Width of the left frame
*
* Equivalent to -left-frameset-width
*
*
* @parameter expression="${flex.leftFramesetWidth}"
*/
private Integer leftFramesetWidth;
/**
* Report well-formed HTML errors as warnings
*
* Equivalent to -lenient
*
*
* @parameter expression="${flex.lenient}"
*/
private Boolean lenient;
/**
* Title to be displayed in the title bar
*
* Equivalent to -main-title
*
*
* @parameter default-value="${project.name} Documentation" expression="${flex.mainTitle}"
*/
private String mainTitle;
/**
* The filename of bundled asdoc
*
* @parameter default-value="${project.build.directory}/${project.build.finalName}-asdoc.zip"
*/
private File output;
/**
* @component
*/
private ProjectBuilder projectBuilder;
/**
* @parameter expression="${reactorProjects}"
* @required
* @readonly
*/
private List reactorProjects;
/**
* DOCME undocumented by adobe
*
* Equivalent to -restore-builtin-classes
*
* Restore-builtin-classes is only for internal use
*
* @parameter expression="${flex.restoreBuiltinClasses}"
*/
private Boolean restoreBuiltinClasses;
/**
* DOCME undocumented by adobe
*
* Equivalent to -skip-xsl
*
*
* @parameter expression="${flex.skipXsl}"
*/
private Boolean skipXsl;
/**
* TODO
*
* @parameter
*/
private File templatePath;
/**
* Title to be displayed in the browser window
*
* Equivalent to -window-title
*
*
* @parameter default-value="${project.name} Documentation" expression="${flex.windowTitle}"
*/
private String windowTitle;
private void attachAsdoc()
throws Exception
{
Archiver archiver = archiverManager.getArchiver( output );
archiver.addDirectory( new File( getOutput() ) );
archiver.setDestFile( output );
archiver.createArchive();
projectHelper.attachArtifact( project, PathUtil.fileExtention( output ), "asdoc", output );
}
@Override
public Result doCompile( IASDocConfiguration cfg, boolean synchronize )
throws Exception
{
return compiler.asdoc( cfg, synchronize, compilerName );
}
public void fmExecute()
throws MojoExecutionException, MojoFailureException
{
if ( aggregate && !project.isExecutionRoot() )
{
getLog().info( "Skipping asdoc execution, aggregate mode active." );
return;
}
if ( !PathUtil.existAny( getSourcePath() ) )
{
getLog().warn( "Skipping asdoc, source path doesn't exist." );
return;
}
wait( executeCompiler( this, true ) );
if ( attach )
{
try
{
attachAsdoc();
}
catch ( Exception e )
{
throw new MojoExecutionException( "Failed to create asdoc bundle", e );
}
}
}
public Boolean getDateInFooter()
{
return dateInFooter;
}
public List getDocClasses()
{
if ( docClasses == null )
{
return null;
}
List classes = new ArrayList();
classes.addAll( docClasses.getIncludes() );
classes.addAll( filterClasses( docClasses.getPatterns(), getSourcePath() ) );
return classes;
}
public List getDocNamespaces()
{
if ( docNamespaces != null )
{
return docNamespaces;
}
if ( docAllNamespaces )
{
return getNamespacesUri();
}
return null;
}
public File[] getDocSources()
{
if ( docSources == null && getDocNamespaces() == null && docClasses == null )
{
return getSourcePath();
}
return docSources;
}
@Override
public String getDumpConfig()
{
return null;
}
public String getExamplesPath()
{
return PathUtil.path( examplesPath );
}
public List getExcludeClasses()
{
// TODO Auto-generated method stub
return null;
}
public Boolean getExcludeDependencies()
{
return excludeDependencies;
}
public File[] getExcludeSources()
{
// TODO Auto-generated method stub
return null;
}
@SuppressWarnings( "unchecked" )
@Override
public File[] getExternalLibraryPath()
{
return MavenUtils.getFiles( getGlobalArtifactCollection() );
}
public String getFooter()
{
return footer;
}
public Boolean getIncludeAllForAsdoc()
{
return includeAllForAsdoc;
}
@Override
public File[] getIncludeLibraries()
{
return null;
}
public Boolean getIncludeLookupOnly()
{
return includeLookupOnly;
}
public Boolean getKeepXml()
{
return keepXml;
}
public Integer getLeftFramesetWidth()
{
return leftFramesetWidth;
}
public Boolean getLenient()
{
return lenient;
}
@SuppressWarnings( "unchecked" )
@Override
public File[] getLibraryPath()
{
Matcher extends Artifact>[] filter =
new Matcher[] { type( SWC ), not( scope( TEST ) ), not( GLOBAL_MATCHER ) };
if ( aggregate )
{
Set deps = new LinkedHashSet();
for ( MavenProject p : reactorProjects )
{
if ( !( SWC.equals( p.getPackaging() ) || SWF.equals( p.getPackaging() ) || AIR.equals( p.getPackaging() ) ) )
{
continue;
}
ProjectBuildingRequest request = new DefaultProjectBuildingRequest();
request.setLocalRepository( localRepository );
request.setRemoteRepositories( remoteRepositories );
request.setResolveDependencies( true );
request.setRepositorySession( session.getRepositorySession() );
try
{
p = projectBuilder.build( p.getArtifact(), request ).getProject();
}
catch ( ProjectBuildingException e )
{
throw new MavenRuntimeException( e.getMessage(), e );
}
deps.addAll( MavenUtils.getFilesSet( filter( allOf( filter ), p.getArtifacts() ) ) );
}
deps.addAll( MavenUtils.getFilesSet( getCompiledResouceBundles() ) );
return deps.toArray( new File[0] );
}
else
{
return MavenUtils.getFiles( getDependencies( filter ), getCompiledResouceBundles() );
}
}
@Override
public String getLinkReport()
{
return null;
}
public String getMainTitle()
{
return mainTitle;
}
public String getOutput()
{
asdocOutputDirectory.mkdirs();
return PathUtil.path( asdocOutputDirectory );
}
public String[] getPackage()
{
// TODO Auto-generated method stub
return null;
}
public String getPackageDescriptionFile()
{
// TODO Auto-generated method stub
return null;
}
public IPackagesConfiguration getPackagesConfiguration()
{
return this;
}
public Boolean getRestoreBuiltinClasses()
{
return restoreBuiltinClasses;
}
@Override
public IRuntimeSharedLibraryPath[] getRuntimeSharedLibraryPath()
{
return null;
}
@Override
public String getSizeReport()
{
return null;
}
public Boolean getSkipXsl()
{
return skipXsl;
}
@Override
public File[] getSourcePath()
{
if ( aggregate )
{
List files = new ArrayList();
for ( MavenProject p : reactorProjects )
{
files.addAll( PathUtil.existingFilesList( p.getCompileSourceRoots() ) );
}
return files.toArray( new File[0] );
}
else
{
return PathUtil.existingFiles( super.getSourcePath() );
}
}
public String getTemplatesPath()
{
if ( templatePath != null )
{
return PathUtil.path( templatePath );
}
File templateOutput = new File( project.getBuild().getDirectory(), "templates" );
templateOutput.mkdirs();
Artifact template = resolve( "org.apache.flex.compiler", "asdoc", getCompilerVersion(), "template", "zip" );
try
{
UnArchiver unarchiver = archiverManager.getUnArchiver( "zip" );
unarchiver.setDestDirectory( templateOutput );
unarchiver.setSourceFile( template.getFile() );
unarchiver.extract();
}
catch ( Exception e )
{
throw new MavenRuntimeException( "Unable to unpack asdoc template", e );
}
return PathUtil.path( templateOutput );
}
public String getWindowTitle()
{
return windowTitle;
}
}