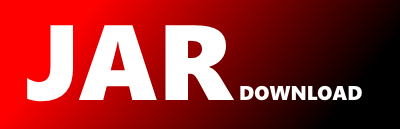
net.forthecrown.grenadier.annotations.compiler.CompileContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grenadier-annotations Show documentation
Show all versions of grenadier-annotations Show documentation
Annotation-based command support for Grenadier
package net.forthecrown.grenadier.annotations.compiler;
import com.google.common.base.Joiner;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Stack;
import java.util.function.Predicate;
import lombok.Getter;
import lombok.RequiredArgsConstructor;
import net.forthecrown.grenadier.CommandSource;
import net.forthecrown.grenadier.Grenadier;
import net.forthecrown.grenadier.GrenadierCommandNode;
import net.forthecrown.grenadier.annotations.ArgumentModifier;
import net.forthecrown.grenadier.annotations.SyntaxConsumer;
import net.forthecrown.grenadier.annotations.Token;
import net.forthecrown.grenadier.annotations.TypeRegistry;
import net.forthecrown.grenadier.annotations.tree.VariableHolder;
import net.forthecrown.grenadier.annotations.util.Result;
@Getter
@RequiredArgsConstructor
public class CompileContext {
static final boolean DEBUG_LOGGING = true;
private final Map variables;
private final ClassLoader loader;
private final TypeRegistry typeRegistry;
private final Object commandClass;
private final String defaultPermission;
final CompileErrors errors;
final SyntaxList syntaxList = new SyntaxList();
final Stack availableArguments = new Stack<>();
final Stack mappers = new Stack<>();
final Stack syntaxPrefixes = new Stack<>();
final Stack> conditions = new Stack<>();
public Result getVariable(VariableHolder variable, Class type) {
return getVariable(variable.tokenStart(), variable.variable(), type);
}
public Result getVariable(Token token, Class type) {
return getVariable(token.position(), token.value(), type);
}
/**
* Gets a variable's optional value
* @param name Name of the variable
* @param type Variable's expected type
* @return Value optional
* @param Variable's type
* @throws IllegalStateException If the variable was found, but its type did not
* match the specified {@code type}
*/
public Result getVariable(int position, String name, Class type)
throws IllegalStateException
{
Object value = variables.get(name);
if (value == null) {
return Result.fail(position, "Variable '%s' not found", name);
}
if (!type.isInstance(value)) {
return Result.fail(position,
"Variable '%s' is defined as %s, must be '%s'",
name, value.getClass().getName(), type.getName()
);
}
return Result.success((T) value);
}
/**
* Formats the default permission with a command name, if present.
*
* @param commandName Command name
* @return Formatted default permission, or {@code null}, if no default
* permission was set
*/
public String defaultedPermission(String commandName) {
if (defaultPermission == null) {
return null;
}
return defaultPermission.replace("{command}", commandName);
}
public Predicate buildConditions() {
if (conditions.isEmpty()) {
return null;
}
if (conditions.size() == 1) {
return conditions.get(0);
}
Predicate[] predicates = new Predicate[conditions.size()];
return new PredicateList(conditions.toArray(predicates));
}
public void consumeSyntax(GrenadierCommandNode node, SyntaxConsumer consumer) {
syntaxList.consume(node, consumer, getCommandClass());
}
public String syntaxPrefix() {
return Joiner.on(' ').join(syntaxPrefixes);
}
public ContextFactory createFactory() {
Map>> map = new HashMap<>();
for (MapperEntry mapper : mappers) {
var list = map.computeIfAbsent(
mapper.name(), string -> new ArrayList<>()
);
list.add(mapper.modifier());
}
if (DEBUG_LOGGING) {
Grenadier.getLogger().info("Creating context factory, path='{}', mappers.keys={}",
syntaxPrefix(), map.keySet()
);
}
return new ContextFactory(map);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy