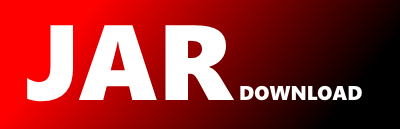
hydra.langs.json.JsonDecoding Maven / Gradle / Ivy
package hydra.langs.json;
import hydra.json.Value;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* Decoding functions for Hydra's native JSON values which are intended for use in Java.
* Decoding failures result in Java exceptions rather than failure flows.
*/
public abstract class JsonDecoding {
/**
* Decode a list from JSON.
*/
public static List decodeList(Function mapping, Value json) {
return json.accept(new Value.PartialVisitor>() {
@Override
public List otherwise(Value instance) {
throw unexpected("array", instance);
}
@Override
public List visit(Value.Array instance) {
return instance.value.stream().map(mapping).collect(Collectors.toList());
}
@Override
public List visit(Value.Null instance) {
return Collections.emptyList();
}
});
}
/**
* Decode a boolean value from JSON.
*/
public static boolean decodeBoolean(Value json) {
return json.accept(new Value.PartialVisitor() {
@Override
public Boolean otherwise(Value instance) {
throw unexpected("boolean", instance);
}
@Override
public Boolean visit(Value.Boolean_ instance) {
return instance.value;
}
});
}
/**
* Decode a double value from JSON.
*/
public static double decodeDouble(Value json) {
Number num = decodeNumber(json);
return num.doubleValue();
}
/**
* Decode a float value from JSON.
*/
public static float decodeFloat(Value json) {
Number num = decodeNumber(json);
return num.floatValue();
}
/**
* Decode an integer value from JSON.
*/
public static int decodeInteger(Value json) {
Number num = decodeNumber(json);
return num.intValue();
}
/**
* Decode a list field from JSON.
*/
public static List decodeListField(String name, Function mapping, Value json) {
// Note: this allows the field to be omitted, and also allows a null value, both resulting in an empty list
Optional> opt = decodeOptionalField(name, v -> decodeList(mapping, v), json, Collections.emptyList());
return opt.orElse(Collections.emptyList());
}
/**
* Decode a number from JSON.
*/
public static double decodeNumber(Value json) {
return json.accept(new Value.PartialVisitor() {
@Override
public Double otherwise(Value instance) {
throw unexpected("number", instance);
}
@Override
public Double visit(Value.Number_ instance) {
return instance.value;
}
});
}
/**
* Decode an object (key/value map) from JSON.
*/
public static Map decodeObject(Value json) {
return json.accept(new Value.PartialVisitor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy