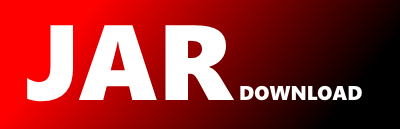
hydra.langs.json.JsonIoCoder Maven / Gradle / Ivy
package hydra.langs.json;
import com.cedarsoftware.util.io.JsonObject;
import hydra.Flows;
import hydra.compute.Coder;
import hydra.compute.Flow;
import hydra.json.Value;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* A bidirectional coder between Hydra's native JSON values and the JSON objects supported by json-io.
*/
public class JsonIoCoder extends Coder {
public JsonIoCoder() {
super(JsonIoCoder::encode, JsonIoCoder::decode);
}
/**
* Encode a JSON value as a json-io object.
*/
public static Flow encode(Value value) {
return value.accept(new Value.Visitor>() {
@Override
public Flow visit(Value.Array instance) {
return Flows.map(Flows.mapM(instance.value, JsonIoCoder::encode), List::toArray);
}
@Override
public Flow visit(Value.Boolean_ instance) {
return Flows.pure(instance.value);
}
@Override
public Flow visit(Value.Null instance) {
// Note: we return an object here rather than a null, as Hydra flows cannot accept nulls as values.
// This is acceptable so long as we are only using the generated java-io objects internally,
// for serialization. See normalizeEncoded().
return Flows.pure(instance);
}
@Override
public Flow visit(Value.Number_ instance) {
return Flows.pure(instance.value);
}
@Override
public Flow visit(Value.Object_ instance) {
return Flows.map(Flows.mapM(instance.value, Flows::pure, JsonIoCoder::encode), m -> {
JsonObject obj = new JsonObject();
obj.putAll(m);
return obj;
});
}
@Override
public Flow visit(Value.String_ instance) {
return Flows.pure(instance.value);
}
});
}
/**
* Decode a json-io object as a JSON value.
*/
public static Flow decode(Object value) {
if (value == null) {
return Flows.pure(new Value.Null());
} else if (value.getClass().isArray()) {
Object[] array = (Object[]) value;
return Flows.map(Flows.mapM(array, JsonIoCoder::decode), Value.Array::new);
} else if (value instanceof JsonObject) {
return Flows.map(Flows.mapM((Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy