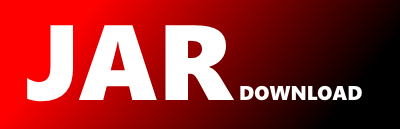
hydra.module.Module Maven / Gradle / Ivy
package hydra.module;
import java.io.Serializable;
/**
* A logical collection of elements in the same namespace, having dependencies on zero or more other modules
*/
public class Module implements Serializable {
public static final hydra.core.Name NAME = new hydra.core.Name("hydra/module.Module");
/**
* A common prefix for all element names in the module
*/
public final hydra.module.Namespace namespace;
/**
* The elements defined in this module
*/
public final java.util.List> elements;
/**
* Any modules which the term expressions of this module directly depend upon
*/
public final java.util.List> termDependencies;
/**
* Any modules which the type expressions of this module directly depend upon
*/
public final java.util.List> typeDependencies;
/**
* An optional human-readable description of the module
*/
public final java.util.Optional description;
public Module (hydra.module.Namespace namespace, java.util.List> elements, java.util.List> termDependencies, java.util.List> typeDependencies, java.util.Optional description) {
this.namespace = namespace;
this.elements = elements;
this.termDependencies = termDependencies;
this.typeDependencies = typeDependencies;
this.description = description;
}
@Override
public boolean equals(Object other) {
if (!(other instanceof Module)) {
return false;
}
Module o = (Module) (other);
return namespace.equals(o.namespace) && elements.equals(o.elements) && termDependencies.equals(o.termDependencies) && typeDependencies.equals(o.typeDependencies) && description.equals(o.description);
}
@Override
public int hashCode() {
return 2 * namespace.hashCode() + 3 * elements.hashCode() + 5 * termDependencies.hashCode() + 7 * typeDependencies.hashCode() + 11 * description.hashCode();
}
public Module withNamespace(hydra.module.Namespace namespace) {
return new Module(namespace, elements, termDependencies, typeDependencies, description);
}
public Module withElements(java.util.List> elements) {
return new Module(namespace, elements, termDependencies, typeDependencies, description);
}
public Module withTermDependencies(java.util.List> termDependencies) {
return new Module(namespace, elements, termDependencies, typeDependencies, description);
}
public Module withTypeDependencies(java.util.List> typeDependencies) {
return new Module(namespace, elements, termDependencies, typeDependencies, description);
}
public Module withDescription(java.util.Optional description) {
return new Module(namespace, elements, termDependencies, typeDependencies, description);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy