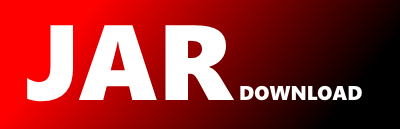
hydra.tier1.Tier1 Maven / Gradle / Ivy
package hydra.tier1;
import hydra.compute.Flow;
import hydra.compute.FlowState;
import hydra.compute.Trace;
import hydra.mantle.Either;
import java.util.function.Function;
/**
* A module for miscellaneous tier-1 functions and constants.
*/
public interface Tier1 {
static Double floatValueToBigfloat(hydra.core.FloatValue v1) {
return ((v1)).accept(new hydra.core.FloatValue.Visitor() {
@Override
public Double visit(hydra.core.FloatValue.Bigfloat instance) {
return hydra.lib.equality.Identity.apply((instance.value));
}
@Override
public Double visit(hydra.core.FloatValue.Float32 instance) {
return hydra.lib.literals.Float32ToBigfloat.apply((instance.value));
}
@Override
public Double visit(hydra.core.FloatValue.Float64 instance) {
return hydra.lib.literals.Float64ToBigfloat.apply((instance.value));
}
});
}
static java.math.BigInteger integerValueToBigint(hydra.core.IntegerValue v1) {
return ((v1)).accept(new hydra.core.IntegerValue.Visitor() {
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Bigint instance) {
return hydra.lib.equality.Identity.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Int8 instance) {
return hydra.lib.literals.Int8ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Int16 instance) {
return hydra.lib.literals.Int16ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Int32 instance) {
return hydra.lib.literals.Int32ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Int64 instance) {
return hydra.lib.literals.Int64ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Uint8 instance) {
return hydra.lib.literals.Uint8ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Uint16 instance) {
return hydra.lib.literals.Uint16ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Uint32 instance) {
return hydra.lib.literals.Uint32ToBigint.apply((instance.value));
}
@Override
public java.math.BigInteger visit(hydra.core.IntegerValue.Uint64 instance) {
return hydra.lib.literals.Uint64ToBigint.apply((instance.value));
}
});
}
static Boolean isLambda(hydra.core.Term term) {
return (hydra.strip.Strip.stripTerm((term))).accept(new hydra.core.Term.PartialVisitor() {
@Override
public Boolean otherwise(hydra.core.Term instance) {
return false;
}
@Override
public Boolean visit(hydra.core.Term.Function instance) {
return ((instance.value)).accept(new hydra.core.Function.PartialVisitor() {
@Override
public Boolean otherwise(hydra.core.Function instance) {
return false;
}
@Override
public Boolean visit(hydra.core.Function.Lambda instance) {
return true;
}
});
}
@Override
public Boolean visit(hydra.core.Term.Let instance) {
return hydra.tier1.Tier1.isLambda(((instance.value)).environment);
}
});
}
static hydra.core.Name unqualifyName(hydra.module.QualifiedName qname) {
String prefix = ((((qname)).namespace).map((java.util.function.Function) (n -> hydra.lib.strings.Cat.apply(java.util.Arrays.asList(
((n)).value,
"."))))).orElse("");
return new hydra.core.Name(hydra.lib.strings.Cat.apply(java.util.Arrays.asList(
(prefix),
((qname)).local)));
}
hydra.compute.Trace emptyTrace = new hydra.compute.Trace(java.util.Arrays.asList(), java.util.Arrays.asList(), hydra.lib.maps.Empty.apply());
static java.util.function.Function, Boolean> flowSucceeds(S cx) {
return (java.util.function.Function, Boolean>) (f -> hydra.lib.optionals.IsJust.apply((((((f)).value).apply((cx))).apply((hydra.tier1.Tier1.emptyTrace))).value));
}
static java.util.function.Function, A>> fromFlow(A def) {
return (java.util.function.Function, A>>) (cx -> (java.util.function.Function, A>) (f -> (((((((f)).value).apply((cx))).apply((hydra.tier1.Tier1.emptyTrace))).value).map((java.util.function.Function) (x -> (x)))).orElse((def))));
}
static java.util.function.Function>, java.util.function.Function, hydra.compute.Flow>> mutateTrace(java.util.function.Function> mutate) {
return (java.util.function.Function>, java.util.function.Function, hydra.compute.Flow>>) (restore -> (java.util.function.Function, hydra.compute.Flow>) (f -> new hydra.compute.Flow((java.util.function.Function>>) (s0 -> (java.util.function.Function>) (t0 -> {
java.util.function.Function> forRight = t1 -> {
hydra.compute.FlowState f2 = ((((f)).value).apply((s0))).apply((t1));
return new hydra.compute.FlowState<>(((f2)).value, ((f2)).state, (((restore)).apply((t0))).apply(((f2)).trace));
};
java.util.function.Function> forLeft = msg -> new hydra.compute.FlowState<>(java.util.Optional.empty(), (s0), (Tier1.pushError((msg))).apply((t0)));
return mutate.apply(t0).accept(new Either.Visitor>() {
@Override
public FlowState visit(Either.Left instance) {
return ((forLeft)).apply((instance.value));
}
@Override
public FlowState visit(Either.Right instance) {
return ((forRight)).apply((instance.value));
}
});
})))));
}
static java.util.function.Function pushError(String msg) {
return (java.util.function.Function) (t -> {
String errorMsg = hydra.lib.strings.Cat.apply(java.util.Arrays.asList(
"Error: ",
(msg),
" (",
hydra.lib.strings.Intercalate.apply(
" > ",
hydra.lib.lists.Reverse.apply(((t)).stack)),
")"));
return new hydra.compute.Trace(((t)).stack, hydra.lib.lists.Cons.apply(
(errorMsg),
((t)).messages), ((t)).other);
});
}
static java.util.function.Function, hydra.compute.Flow> warn(String msg) {
return (java.util.function.Function, hydra.compute.Flow>) (b -> new hydra.compute.Flow((java.util.function.Function>>) (s0 -> (java.util.function.Function>) (t0 -> {
hydra.compute.FlowState f1 = ((((b)).value).apply((s0))).apply((t0));
java.util.function.Function addMessage = (java.util.function.Function) (t -> new hydra.compute.Trace(((t)).stack, hydra.lib.lists.Cons.apply(
hydra.lib.strings.Cat.apply(java.util.Arrays.asList(
"Warning: ",
(msg))),
((t)).messages), ((t)).other));
return new hydra.compute.FlowState(((f1)).value, ((f1)).state, ((addMessage)).apply(((f1)).trace));
}))));
}
static java.util.function.Function, hydra.compute.Flow> withFlag(String a1) {
java.util.function.Function> restore = (java.util.function.Function>) (ignored -> (java.util.function.Function) (t1 -> new hydra.compute.Trace(((t1)).stack, ((t1)).messages, hydra.lib.maps.Remove.apply(
(a1),
((t1)).other))));
java.util.function.Function> mutate = (t -> new hydra.mantle.Either.Right<>(new hydra.compute.Trace(((t)).stack, ((t)).messages, hydra.lib.maps.Insert.apply(
(a1),
new hydra.core.Term.Literal(new hydra.core.Literal.Boolean_(true)),
((t)).other))));
Function>, Function, Flow>> r = mutateTrace(mutate);
return r.apply(restore);
}
static java.util.function.Function, hydra.compute.Flow> withState(S1 cx0) {
return (f -> new hydra.compute.Flow<>((cx1 -> (t1 -> {
hydra.compute.FlowState f1 = ((((f)).value).apply((cx0))).apply((t1));
return new hydra.compute.FlowState(((f1)).value, (cx1), ((f1)).trace);
}))));
}
static java.util.function.Function, hydra.compute.Flow> withTrace(String a1) {
java.util.function.Function> restore = (java.util.function.Function>) (t0 -> (java.util.function.Function) (t1 -> new hydra.compute.Trace(((t0)).stack, ((t1)).messages, ((t1)).other)));
java.util.function.Function> mutate = (java.util.function.Function>) (t -> hydra.lib.logic.IfElse.apply(
new hydra.mantle.Either.Left<>("maximum trace depth exceeded. This may indicate an infinite loop"),
new hydra.mantle.Either.Right<>(new hydra.compute.Trace(hydra.lib.lists.Cons.apply(
(a1),
((t)).stack), ((t)).messages, ((t)).other)),
hydra.lib.equality.GteInt32.apply(
hydra.lib.lists.Length.apply(((t)).stack),
(hydra.constants.Constants.maxTraceDepth))));
Function>, Function, Flow>> r = mutateTrace(mutate);
return r.apply(restore);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy