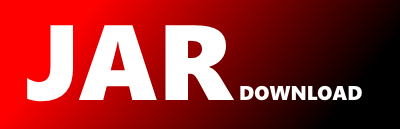
hydra.tools.LList Maven / Gradle / Ivy
package hydra.tools;
import java.util.ArrayList;
import java.util.List;
/**
* A simple tail-sharing linked list.
*/
public class LList {
public final X first;
public final LList rest;
/**
* Construct a list using a given head and tail (where the tail may be null).
*/
public LList(X first, LList rest) {
this.first = first;
this.rest = rest;
}
/**
* Find the length of a list (which may be null).
*/
public static int length(LList list) {
LList cur = list;
int len = 0;
while (cur != null) {
len++;
cur = cur.rest;
}
return len;
}
/**
* Check whether a list is empty (null).
*/
public static boolean isEmpty(LList list) {
return list == null;
}
/**
* Construct a list by logically pushing a value to the head of another list (which is not modified).
*/
public static LList push(X value, LList list) {
return new LList<>(value, list);
}
/**
* Construct a list by logically dropping n values from the head of another list (which is not modified).
*/
public static LList drop(int n, LList list) {
LList cur = list;
for (int i = 0; i < n; i++) {
cur = cur.rest;
}
return cur;
}
/**
* Construct a list by logically taking the first n values from the head of another list (which is not modified).
*/
public static List take(int n, LList list) {
List javaList = new ArrayList<>();
LList cur = list;
for (int i = 0; i < n; i++) {
javaList.add(cur.first);
cur = cur.rest;
}
return javaList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy