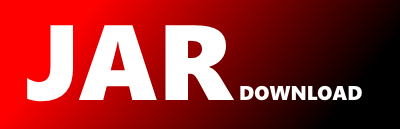
net.fortytwo.sesametools.CompoundCloseableIteration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Common utilities for Sesametools
The newest version!
package net.fortytwo.sesametools;
import info.aduna.iteration.CloseableIteration;
import java.util.Collection;
import java.util.Iterator;
/**
* A CloseableIteration which wraps an ordered collection of other CloseableIterations.
*
* @author Joshua Shinavier (http://fortytwo.net)
*/
public class CompoundCloseableIteration implements CloseableIteration {
private Iterator> iterations;
private CloseableIteration currentIteration;
public CompoundCloseableIteration(final Collection> childIterations) {
iterations = childIterations.iterator();
if (iterations.hasNext()) {
currentIteration = iterations.next();
} else {
currentIteration = null;
}
}
public void close() throws E {
if (null != currentIteration) {
currentIteration.close();
currentIteration = null;
}
while (iterations.hasNext()) {
iterations.next().close();
}
}
public boolean hasNext() throws E {
if (null == currentIteration) {
return false;
} else if (currentIteration.hasNext()) {
return true;
} else {
currentIteration.close();
if (iterations.hasNext()) {
currentIteration = iterations.next();
// Recurse until a non-empty iteration is found.
return hasNext();
} else {
currentIteration = null;
return false;
}
}
}
public T next() throws E {
return currentIteration.next();
}
public void remove() throws E {
currentIteration.remove();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy