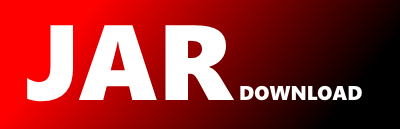
net.freeutils.util.db.ResultsHandlers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jelementary Show documentation
Show all versions of jelementary Show documentation
The Java Elementary Utilities package
The newest version!
/*
* Copyright © 2003-2024 Amichai Rothman
*
* This file is part of JElementary - the Java Elementary Utilities package.
*
* JElementary is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 2 of the License, or
* (at your option) any later version.
*
* JElementary is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with JElementary. If not, see .
*
* For additional info see https://www.freeutils.net/source/jelementary/
*/
package net.freeutils.util.db;
import net.freeutils.util.Reflect;
import net.freeutils.util.Strings;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* A utility class containing commonly used ResultsHandler implementations.
*/
public class ResultsHandlers {
// private constructor to prevent instantiation
private ResultsHandlers() {}
/**
* A ResultsHandler that returns true if there are any results
* (the result set is not empty), or false if there are no results
* (the result set is empty).
*/
public static final ResultsHandler EXISTS_HANDLER = new ResultsHandler() {
@Override
public Boolean handle(ResultSet rs) throws SQLException {
return rs.next();
}
};
/**
* A ResultsHandler that returns the object at the first column of the first row,
* or null if the result set is empty.
*/
public static final ResultsHandler
© 2015 - 2024 Weber Informatics LLC | Privacy Policy