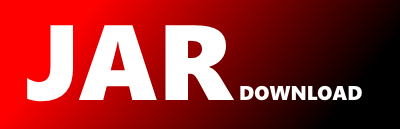
net.guerlab.cloud.commons.util.BeanConvertUtils Maven / Gradle / Ivy
/*
* Copyright 2018-2024 guerlab.net and other contributors.
*
* Licensed under the GNU LESSER GENERAL PUBLIC LICENSE, Version 3 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.gnu.org/licenses/lgpl-3.0.html
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.guerlab.cloud.commons.util;
import java.util.Collection;
import java.util.List;
import java.util.function.Function;
import org.springframework.beans.BeanUtils;
import org.springframework.lang.Nullable;
import net.guerlab.cloud.core.dto.Convert;
import net.guerlab.cloud.core.result.Pageable;
import net.guerlab.commons.collection.CollectionUtil;
import net.guerlab.commons.exception.ApplicationException;
/**
* Bean转换工具类.
*
* @author guer
*/
@SuppressWarnings({"unused"})
public final class BeanConvertUtils {
private BeanConvertUtils() {
}
/**
* 转换为目标类型.
*
* @param 目标类型
* @param 实体类型
* @param entity 实体
* @return 目标类型
*/
@Nullable
public static > T toObject(@Nullable E entity) {
return entity == null ? null : entity.convert();
}
/**
* 转换为目标类型.
*
* @param 目标类型
* @param 实体类型
* @param entity 实体
* @param targetClass 目标类型
* @return 目标类型
*/
@Nullable
public static T toObject(@Nullable E entity, Class targetClass) {
if (entity == null) {
return null;
}
T target;
try {
target = targetClass.getDeclaredConstructor().newInstance();
}
catch (Exception e) {
throw new ApplicationException(e.getLocalizedMessage(), e);
}
BeanUtils.copyProperties(entity, target);
return target;
}
/**
* 转换为目标列表.
*
* @param 目标类型
* @param 实体类型
* @param entityList 实体列表
* @return 目标列表
*/
public static > List toList(Collection entityList) {
return CollectionUtil.toList(entityList, Convert::convert);
}
/**
* 转换为目标列表.
*
* @param 目标类型
* @param 实体类型
* @param entityList 实体列表
* @param targetClass 目标类型
* @return 目标列表
*/
public static List toList(Collection entityList, Class targetClass) {
return CollectionUtil.toList(entityList, e -> toObject(e, targetClass));
}
/**
* 转换为目标列表对象.
*
* @param 目标类型
* @param 实体类型
* @param list 实体列表对象
* @return 目标列表对象
*/
public static > Pageable toPageable(@Nullable Pageable list) {
if (list == null) {
return Pageable.empty();
}
Pageable result = copyPageable(list);
result.setList(toList(list.getList()));
return result;
}
/**
* 转换为目标列表对象.
*
* @param 目标类型
* @param 实体类型
* @param list 实体列表对象
* @param dtoClass 目标类型
* @return 目标列表对象
*/
public static Pageable toPageable(@Nullable Pageable list, Class dtoClass) {
if (list == null) {
return Pageable.empty();
}
Pageable result = copyPageable(list);
result.setList(toList(list.getList(), dtoClass));
return result;
}
/**
* 转换为目标列表对象.
*
* @param 目标类型
* @param 实体类型
* @param list 实体列表对象
* @param convertFunction 对象转换方法
* @return 目标列表对象
*/
public static Pageable toPageable(@Nullable Pageable list, Function convertFunction) {
if (list == null) {
return Pageable.empty();
}
Pageable result = copyPageable(list);
result.setList(CollectionUtil.toList(list.getList(), convertFunction));
return result;
}
private static Pageable copyPageable(Pageable> origin) {
Pageable result = new Pageable<>();
result.setPageSize(origin.getPageSize());
result.setCount(origin.getCount());
result.setCurrentPageId(origin.getCurrentPageId());
result.setMaxPageId(origin.getMaxPageId());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy