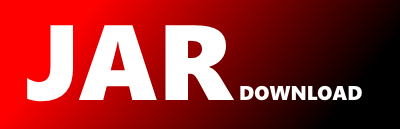
net.guerlab.cloud.searchparams.mybatisplus.CollectionHandler Maven / Gradle / Ivy
/*
* Copyright 2018-2025 guerlab.net and other contributors.
*
* Licensed under the GNU LESSER GENERAL PUBLIC LICENSE, Version 3 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.gnu.org/licenses/lgpl-3.0.html
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.guerlab.cloud.searchparams.mybatisplus;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import jakarta.annotation.Nullable;
import net.guerlab.cloud.searchparams.JsonField;
import net.guerlab.cloud.searchparams.SearchModelType;
/**
* 集合元素处理.
*/
public class CollectionHandler extends AbstractMyBatisPlusSearchParamsHandler {
private static String buildReplacement(int size) {
StringBuilder replacementBuilder = new StringBuilder();
replacementBuilder.append("(");
for (int i = 0; i < size; i++) {
if (i != 0) {
replacementBuilder.append(", ");
}
replacementBuilder.append("{");
replacementBuilder.append(i);
replacementBuilder.append("}");
}
replacementBuilder.append(")");
return replacementBuilder.toString();
}
@Override
public Class> acceptClass() {
return Collection.class;
}
@SuppressWarnings("unchecked")
@Override
public void setValue(Object object, String fieldName, String columnName, Object value,
SearchModelType searchModelType, @Nullable String customSql, @Nullable JsonField jsonField) {
Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy