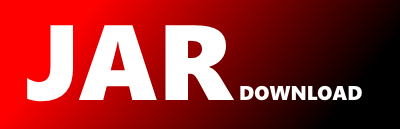
net.guerlab.smart.article.service.service.impl.ArticleServiceImpl Maven / Gradle / Ivy
package net.guerlab.smart.article.service.service.impl;
import net.guerlab.commons.collection.CollectionUtil;
import net.guerlab.commons.number.NumberHelper;
import net.guerlab.smart.article.core.eneity.Attachments;
import net.guerlab.smart.article.core.enums.AuditStatus;
import net.guerlab.smart.article.core.enums.PublishType;
import net.guerlab.smart.article.core.exception.*;
import net.guerlab.smart.article.core.searchparams.ArticleCategoryMappingSearchParams;
import net.guerlab.smart.article.core.searchparams.ArticleCategorySearchParams;
import net.guerlab.smart.article.core.searchparams.ArticleSearchParams;
import net.guerlab.smart.article.service.entity.Article;
import net.guerlab.smart.article.service.entity.ArticleCategory;
import net.guerlab.smart.article.service.entity.ArticleCategoryMapping;
import net.guerlab.smart.article.service.mapper.ArticleMapper;
import net.guerlab.smart.article.service.service.ArticleCategoryUpdateAfterHandler;
import net.guerlab.smart.article.service.service.ArticleService;
import net.guerlab.smart.platform.commons.domain.MultiId;
import net.guerlab.smart.platform.commons.util.OrderEntityUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.stereotype.Service;
import java.time.LocalDateTime;
import java.util.Collection;
import java.util.Objects;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
/**
* 文章服务实现
*
* @author guer
*/
@Service
public class ArticleServiceImpl extends AbstractArticleServiceImpl
implements ArticleService, ArticleCategoryUpdateAfterHandler {
@Override
public void articleCategoryUpdateAfterHandler(ArticleCategory articleCategory) {
Long categoryId = articleCategory.getArticleCategoryId();
String categoryName = StringUtils.trimToNull(articleCategory.getArticleCategoryName());
String categoryType = StringUtils.trimToNull(articleCategory.getArticleCategoryType());
if (refuseUpdateArticleCategory(categoryId, categoryName, categoryType)) {
return;
}
Article entity = new Article();
entity.setArticleCategoryName(categoryName);
entity.setArticleCategoryType(categoryType);
ArticleSearchParams searchParams = new ArticleSearchParams();
searchParams.setArticleCategoryId(categoryId);
mapper.updateByExampleSelective(entity, getExample(searchParams));
}
private boolean refuseUpdateArticleCategory(Long categoryId, String categoryName, String categoryType) {
return !NumberHelper.greaterZero(categoryId) || (categoryName == null && categoryType == null);
}
@Override
public void addViewNumber(Long articleId) {
if (!NumberHelper.greaterZero(articleId)) {
return;
}
mapper.addViewNumber(articleId);
}
@Override
protected void insertBefore(Article entity) {
String title = StringUtils.trimToNull(entity.getTitle());
if (title == null) {
throw new ArticleTitleInvalidException();
} else if (title.length() > TITLE_MAX_LENGTH) {
throw new ArticleTitleLengthErrorException();
}
entity.setTitle(title);
setCoverUrl(entity);
setAuthor(entity);
setSynopsis(entity);
setOriginalLink(entity);
setUniqueKey(entity);
entity.setContent(StringUtils.trimToEmpty(entity.getContent()));
ArticleCategory articleCategory = findArticleCategory(entity.getArticleCategoryId());
entity.setArticleCategoryName(articleCategory.getArticleCategoryName());
entity.setArticleCategoryType(articleCategory.getArticleCategoryType());
articleCategoryIdsFieldHandler(entity);
LocalDateTime now = LocalDateTime.now();
if (entity.getReleaseTime() == null) {
entity.setReleaseTime(now);
}
if (entity.getAlwaysRedirect() == null) {
entity.setAlwaysRedirect(false);
}
PublishType publishType = entity.getPublishType();
if (publishType == null) {
publishType = PublishType.AUTOMATIC;
}
entity.setPublishType(publishType);
Attachments attachments = entity.getAttachments();
if (attachments != null) {
attachments = attachments.stream().filter(Objects::nonNull)
.collect(Collectors.toCollection(Attachments::new));
entity.setAttachments(attachments);
entity.setHasAttachment(!attachments.isEmpty());
} else {
entity.setAttachments(new Attachments());
entity.setHasAttachment(false);
}
entity.setArticleId(sequence.nextId());
entity.setCreateTime(now);
entity.setUpdateTime(now);
if (entity.getAuditStatus() == null) {
entity.setAuditStatus(AuditStatus.WAIT);
}
OrderEntityUtils.propertiesCheck(entity);
entity.setViewNumber(0L);
setPublishType(entity, publishType, now);
}
@Override
protected void insertAfter(Article entity) {
saveArticleCategoryMappings(entity);
}
private void setUniqueKey(Article entity) {
String uniqueKey = StringUtils.trimToNull(entity.getUniqueKey());
if (uniqueKey != null) {
if (uniqueKey.length() > UNIQUE_KEY_MAX_LENGTH) {
throw new UniqueKeyLengthErrorException();
} else if (Pattern.matches(NUMBER_REG, uniqueKey)) {
throw new UniqueKeyFormatErrorException();
} else if (selectByUniqueKey(uniqueKey) != null) {
throw new UniqueKeyRepeatException();
}
} else {
uniqueKey = "";
}
entity.setUniqueKey(uniqueKey);
}
@Override
protected void updateBefore(Article entity) {
String title = StringUtils.trimToNull(entity.getTitle());
if (title != null && title.length() > TITLE_MAX_LENGTH) {
throw new ArticleTitleLengthErrorException();
}
entity.setTitle(title);
setCoverUrl(entity);
setAuthor(entity);
setSynopsis(entity);
setOriginalLink(entity);
ArticleCategory articleCategory = findArticleCategory(entity.getArticleCategoryId());
entity.setArticleCategoryName(articleCategory.getArticleCategoryName());
entity.setArticleCategoryType(articleCategory.getArticleCategoryType());
articleCategoryIdsFieldHandler(entity);
PublishType publishType = entity.getPublishType();
LocalDateTime now = LocalDateTime.now();
if (publishType != null) {
setPublishType(entity, publishType, now);
}
Attachments attachments = entity.getAttachments();
if (attachments != null) {
attachments = attachments.stream().filter(Objects::nonNull)
.collect(Collectors.toCollection(Attachments::new));
entity.setAttachments(attachments);
entity.setHasAttachment(!attachments.isEmpty());
} else {
entity.setAttachments(null);
entity.setHasAttachment(null);
}
entity.setUpdateTime(now);
}
@Override
protected void updateAfter(Article entity) {
ArticleCategoryMappingSearchParams searchParams = new ArticleCategoryMappingSearchParams();
searchParams.setArticleId(entity.getArticleId());
getMappingService().delete(searchParams);
saveArticleCategoryMappings(entity);
}
private void saveArticleCategoryMappings(Article entity) {
Long articleId = entity.getArticleId();
MultiId articleCategoryIds = entity.getArticleCategoryIds();
Collection mappings = articleCategoryIds.stream()
.map(categoryId -> new ArticleCategoryMapping(articleId, categoryId)).collect(Collectors.toList());
getMappingService().save(mappings);
}
private void articleCategoryIdsFieldHandler(Article entity) {
Long articleCategoryId = entity.getArticleCategoryId();
if (entity.getSecondaryArticleCategoryIds() != null) {
entity.getSecondaryArticleCategoryIds().remove(articleCategoryId);
}
MultiId secondaryArticleCategoryIds = findArticleCategoryIds(entity.getSecondaryArticleCategoryIds());
MultiId articleCategoryIds = new MultiId();
articleCategoryIds.add(articleCategoryId);
articleCategoryIds.addAll(secondaryArticleCategoryIds);
entity.setSecondaryArticleCategoryIds(secondaryArticleCategoryIds);
entity.setArticleCategoryIds(articleCategoryIds);
}
private void setCoverUrl(Article entity) {
String coverUrl = StringUtils.trimToEmpty(entity.getCoverUrl());
entity.setCoverUrl(coverUrl);
entity.setHasCoverUrl(!coverUrl.isEmpty());
}
private void setAuthor(Article entity) {
String author = StringUtils.trimToEmpty(entity.getAuthor());
if (author.length() > AUTHOR_MAX_LENGTH) {
throw new AuthorLengthErrorException();
}
entity.setAuthor(author);
}
private void setSynopsis(Article entity) {
String synopsis = StringUtils.trimToEmpty(entity.getSynopsis());
if (synopsis.length() > SYNOPSIS_MAX_LENGTH) {
throw new SynopsisLengthErrorException();
}
entity.setSynopsis(synopsis);
}
private void setOriginalLink(Article entity) {
String originalLink = StringUtils.trimToEmpty(entity.getOriginalLink());
if (originalLink.length() > ORIGINAL_LINK_MAX_LENGTH) {
throw new OriginalLinkLengthErrorException();
}
entity.setOriginalLink(originalLink);
}
private void setPublishType(Article entity, PublishType publishType, LocalDateTime now) {
boolean passAudit = entity.getAuditStatus() == AuditStatus.PASS;
switch (publishType) {
case AUTOMATIC:
entity.setPlanPublishTime(now);
entity.setPublishTime(now);
entity.setPublished(passAudit);
break;
case TIMING:
LocalDateTime planPublishTime = entity.getPlanPublishTime();
if (planPublishTime == null) {
throw new PlanPublishTimeInvalidException();
} else if (planPublishTime.isBefore(now)) {
entity.setPublished(passAudit);
entity.setPublishTime(now);
} else {
entity.setPublishTime(null);
entity.setPublished(false);
}
break;
case MANUAL:
if (entity.getPublished() == null) {
entity.setPlanPublishTime(null);
entity.setPublishTime(null);
} else if (entity.getPublished()) {
entity.setPlanPublishTime(now);
entity.setPublishTime(now);
} else {
entity.setPlanPublishTime(now);
entity.setPublishTime(now);
}
break;
default:
}
}
private ArticleCategory findArticleCategory(Long articleCategoryId) {
if (!NumberHelper.greaterZero(articleCategoryId)) {
throw new ArticleCategoryIdInvalidException();
}
return getCategoryService().selectByIdOptional(articleCategoryId)
.orElseThrow(ArticleCategoryInvalidException::new);
}
private MultiId findArticleCategoryIds(Collection articleCategoryIds) {
if (articleCategoryIds == null || articleCategoryIds.isEmpty()) {
return new MultiId();
}
ArticleCategorySearchParams searchParams = new ArticleCategorySearchParams();
searchParams.setArticleCategoryIds(articleCategoryIds);
MultiId multiId = new MultiId();
multiId.addAll(CollectionUtil
.toSet(getCategoryService().selectAll(searchParams), ArticleCategory::getArticleCategoryId));
return multiId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy