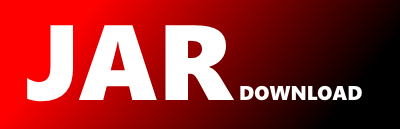
net.guerlab.smart.notify.web.controller.user.SmsSendConfigController Maven / Gradle / Ivy
/*
* Copyright 2018-2021 guerlab.net and other contributors.
*
* Licensed under the GNU LESSER GENERAL PUBLIC LICENSE, Version 3 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.guerlab.smart.notify.web.controller.user;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.security.SecurityRequirement;
import io.swagger.v3.oas.annotations.tags.Tag;
import net.guerlab.smart.notify.core.PermissionConstants;
import net.guerlab.smart.notify.core.domain.SmsSendConfigDTO;
import net.guerlab.smart.notify.core.exceptions.SendConfigInvalidException;
import net.guerlab.smart.notify.core.searchparams.SmsSendConfigSearchParams;
import net.guerlab.smart.notify.service.entity.SmsSendConfig;
import net.guerlab.smart.notify.service.service.SmsSendConfigService;
import net.guerlab.smart.platform.commons.Constants;
import net.guerlab.smart.platform.commons.annotation.HasPermission;
import net.guerlab.smart.platform.commons.util.BeanConvertUtils;
import net.guerlab.smart.user.api.OperationLogApi;
import net.guerlab.smart.user.auth.UserContextHandler;
import net.guerlab.web.result.ListObject;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
/**
* 短信发送配置
*
* @author guer
*/
@Tag(name = "短信发送配置")
@RestController("/user/smsSendConfig")
@RequestMapping("/user/smsSendConfig")
public class SmsSendConfigController {
private SmsSendConfigService service;
private OperationLogApi operationLogApi;
@Operation(description = "查询单个配置", security = @SecurityRequirement(name = Constants.TOKEN))
@GetMapping("/{templateKey}/{manufacturerId}")
public SmsSendConfigDTO findOne(@Parameter(name = "模板ID", required = true) @PathVariable String templateKey, @Parameter(name = "厂商ID", required = true) @PathVariable String manufacturerId) {
return findOne0(templateKey, manufacturerId).convert();
}
@Operation(description = "查询列表", security = @SecurityRequirement(name = Constants.TOKEN))
@GetMapping
public ListObject findList(SmsSendConfigSearchParams searchParams) {
return BeanConvertUtils.toListObject(service.selectPage(searchParams));
}
@Operation(description = "查询全部", security = @SecurityRequirement(name = Constants.TOKEN))
@GetMapping("/all")
public List findAll(SmsSendConfigSearchParams searchParams) {
return BeanConvertUtils.toList(service.selectAll(searchParams));
}
@HasPermission("hasKey(" + PermissionConstants.SEND_CONFIG_MANAGER + ")")
@Operation(description = "添加", security = @SecurityRequirement(name = Constants.TOKEN))
@PostMapping
public SmsSendConfigDTO save(@Parameter(name = "对象数据", required = true) @RequestBody SmsSendConfigDTO dto) {
SmsSendConfig entity = new SmsSendConfig();
BeanUtils.copyProperties(dto, entity);
service.insert(entity);
operationLogApi.add("添加短信发送配置", UserContextHandler.getUserId(), entity);
return entity.convert();
}
@HasPermission("hasKey(" + PermissionConstants.SEND_CONFIG_MANAGER + ")")
@Operation(description = "编辑", security = @SecurityRequirement(name = Constants.TOKEN))
@PutMapping("/{templateKey}/{manufacturerId}")
public SmsSendConfigDTO update(@Parameter(name = "模板ID", required = true) @PathVariable String templateKey, @Parameter(name = "厂商ID", required = true) @PathVariable String manufacturerId,
@Parameter(name = "对象数据", required = true) @RequestBody SmsSendConfigDTO dto) {
SmsSendConfig entity = findOne0(templateKey, manufacturerId);
BeanUtils.copyProperties(dto, entity);
entity.setTemplateKey(templateKey);
entity.setManufacturerId(manufacturerId);
service.updateById(entity);
operationLogApi.add("编辑短信发送配置", UserContextHandler.getUserId(), entity);
return findOne0(templateKey, manufacturerId).convert();
}
@HasPermission("hasKey(" + PermissionConstants.SEND_CONFIG_MANAGER + ")")
@Operation(description = "删除", security = @SecurityRequirement(name = Constants.TOKEN))
@DeleteMapping("/{templateKey}/{manufacturerId}")
public void update(@Parameter(name = "模板ID", required = true) @PathVariable String templateKey, @Parameter(name = "厂商ID", required = true) @PathVariable String manufacturerId) {
findOne0(templateKey, manufacturerId);
service.deleteById(templateKey, manufacturerId);
operationLogApi.add("删除短信发送配置", UserContextHandler.getUserId(), templateKey, manufacturerId);
}
private SmsSendConfig findOne0(String templateKey, String manufacturerId) {
return service.selectByIdOptional(templateKey, manufacturerId).orElseThrow(SendConfigInvalidException::new);
}
@Autowired
public void setService(SmsSendConfigService service) {
this.service = service;
}
@SuppressWarnings("SpringJavaInjectionPointsAutowiringInspection")
@Autowired
public void setOperationLogApi(OperationLogApi operationLogApi) {
this.operationLogApi = operationLogApi;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy