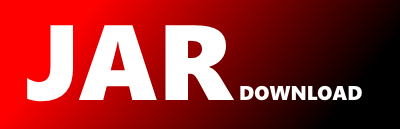
net.guerlab.smart.pay.api.autoconfig.PayOrderApiLocalServiceAutoConfigure Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2018-2021 guerlab.net and other contributors.
*
* Licensed under the GNU LESSER GENERAL PUBLIC LICENSE, Version 3 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.guerlab.smart.pay.api.autoconfig;
import lombok.AllArgsConstructor;
import net.guerlab.smart.pay.api.PayOrderApi;
import net.guerlab.smart.pay.core.domain.PayOrderDTO;
import net.guerlab.smart.pay.core.exception.PayOrderInvalidException;
import net.guerlab.smart.pay.core.searchparams.PayOrderSearchParams;
import net.guerlab.smart.pay.service.entity.PayOrder;
import net.guerlab.smart.pay.service.service.PayOrderService;
import net.guerlab.smart.platform.commons.util.BeanConvertUtils;
import net.guerlab.web.result.ListObject;
import org.springframework.beans.BeanUtils;
import org.springframework.context.annotation.*;
import org.springframework.core.type.AnnotatedTypeMetadata;
import org.springframework.lang.NonNull;
import java.util.List;
/**
* 支付订单服务接口本地实现
*
* @author guer
*/
@Configuration
@Conditional(PayOrderApiLocalServiceAutoConfigure.WrapperCondition.class)
public class PayOrderApiLocalServiceAutoConfigure {
@SuppressWarnings("SpringJavaInjectionPointsAutowiringInspection")
@Bean
public PayOrderApi payOrderApiLocalServiceWrapper(PayOrderService service) {
return new PayOrderApiLocalServiceWrapper(service);
}
public static class WrapperCondition implements Condition {
@Override
public boolean matches(@NonNull ConditionContext context, @NonNull AnnotatedTypeMetadata metadata) {
try {
return WrapperCondition.class.getClassLoader().loadClass("net.guerlab.smart.pay.service.service.PayOrderService") != null;
} catch (Exception e) {
return false;
}
}
}
@AllArgsConstructor
private static class PayOrderApiLocalServiceWrapper implements PayOrderApi {
private final PayOrderService service;
@Override
public PayOrderDTO findOne(Long payOrderId) {
return service.selectByIdOptional(payOrderId).orElseThrow(PayOrderInvalidException::new).convert();
}
@Override
public ListObject findList(PayOrderSearchParams searchParams) {
return BeanConvertUtils.toListObject(service.selectPage(searchParams));
}
@Override
public List findAll(PayOrderSearchParams searchParams) {
return BeanConvertUtils.toList(service.selectAll(searchParams));
}
@Override
public PayOrderDTO add(PayOrderDTO payOrder) {
PayOrder order = new PayOrder();
BeanUtils.copyProperties(payOrder, order);
service.insert(order);
return order.convert();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy