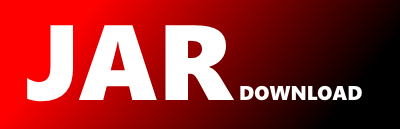
scala.googleapis.bigquery.IndexUnusedReasonCode.scala Maven / Gradle / Ivy
package googleapis.bigquery
import io.circe._
sealed abstract class IndexUnusedReasonCode(val value: String) extends Product with Serializable
object IndexUnusedReasonCode {
/** Code not specified.
*/
case object CODE_UNSPECIFIED extends IndexUnusedReasonCode("CODE_UNSPECIFIED")
/** Indicates the search index configuration has not been created.
*/
case object INDEX_CONFIG_NOT_AVAILABLE extends IndexUnusedReasonCode("INDEX_CONFIG_NOT_AVAILABLE")
/** Indicates the search index creation has not been completed.
*/
case object PENDING_INDEX_CREATION extends IndexUnusedReasonCode("PENDING_INDEX_CREATION")
/** Indicates the base table has been truncated (rows have been removed from table with TRUNCATE TABLE statement) since the last time the search index was refreshed.
*/
case object BASE_TABLE_TRUNCATED extends IndexUnusedReasonCode("BASE_TABLE_TRUNCATED")
/** Indicates the search index configuration has been changed since the last time the search index was refreshed.
*/
case object INDEX_CONFIG_MODIFIED extends IndexUnusedReasonCode("INDEX_CONFIG_MODIFIED")
/** Indicates the search query accesses data at a timestamp before the last time the search index was refreshed.
*/
case object TIME_TRAVEL_QUERY extends IndexUnusedReasonCode("TIME_TRAVEL_QUERY")
/** Indicates the usage of search index will not contribute to any pruning improvement for the search function, e.g. when the search predicate is in a disjunction with other non-search predicates.
*/
case object NO_PRUNING_POWER extends IndexUnusedReasonCode("NO_PRUNING_POWER")
/** Indicates the search index does not cover all fields in the search function.
*/
case object UNINDEXED_SEARCH_FIELDS extends IndexUnusedReasonCode("UNINDEXED_SEARCH_FIELDS")
/** Indicates the search index does not support the given search query pattern.
*/
case object UNSUPPORTED_SEARCH_PATTERN extends IndexUnusedReasonCode("UNSUPPORTED_SEARCH_PATTERN")
/** Indicates the query has been optimized by using a materialized view.
*/
case object OPTIMIZED_WITH_MATERIALIZED_VIEW
extends IndexUnusedReasonCode("OPTIMIZED_WITH_MATERIALIZED_VIEW")
/** Indicates the query has been secured by data masking, and thus search indexes are not applicable.
*/
case object SECURED_BY_DATA_MASKING extends IndexUnusedReasonCode("SECURED_BY_DATA_MASKING")
/** Indicates that the search index and the search function call do not have the same text analyzer.
*/
case object MISMATCHED_TEXT_ANALYZER extends IndexUnusedReasonCode("MISMATCHED_TEXT_ANALYZER")
/** Indicates the base table is too small (below a certain threshold). The index does not provide noticeable search performance gains when the base table is too small.
*/
case object BASE_TABLE_TOO_SMALL extends IndexUnusedReasonCode("BASE_TABLE_TOO_SMALL")
/** Indicates that the total size of indexed base tables in your organization exceeds your region's limit and the index is not used in the query. To index larger base tables, you can use your own reservation for index-management jobs.
*/
case object BASE_TABLE_TOO_LARGE extends IndexUnusedReasonCode("BASE_TABLE_TOO_LARGE")
/** Indicates that the estimated performance gain from using the search index is too low for the given search query.
*/
case object ESTIMATED_PERFORMANCE_GAIN_TOO_LOW
extends IndexUnusedReasonCode("ESTIMATED_PERFORMANCE_GAIN_TOO_LOW")
/** Indicates that search indexes can not be used for search query with STANDARD edition.
*/
case object NOT_SUPPORTED_IN_STANDARD_EDITION
extends IndexUnusedReasonCode("NOT_SUPPORTED_IN_STANDARD_EDITION")
/** Indicates that an option in the search function that cannot make use of the index has been selected.
*/
case object INDEX_SUPPRESSED_BY_FUNCTION_OPTION
extends IndexUnusedReasonCode("INDEX_SUPPRESSED_BY_FUNCTION_OPTION")
/** Indicates that the query was cached, and thus the search index was not used.
*/
case object QUERY_CACHE_HIT extends IndexUnusedReasonCode("QUERY_CACHE_HIT")
/** The index cannot be used in the search query because it is stale.
*/
case object STALE_INDEX extends IndexUnusedReasonCode("STALE_INDEX")
/** Indicates an internal error that causes the search index to be unused.
*/
case object INTERNAL_ERROR extends IndexUnusedReasonCode("INTERNAL_ERROR")
/** Indicates that the reason search indexes cannot be used in the query is not covered by any of the other IndexUnusedReason options.
*/
case object OTHER_REASON extends IndexUnusedReasonCode("OTHER_REASON")
val values = List(
CODE_UNSPECIFIED,
INDEX_CONFIG_NOT_AVAILABLE,
PENDING_INDEX_CREATION,
BASE_TABLE_TRUNCATED,
INDEX_CONFIG_MODIFIED,
TIME_TRAVEL_QUERY,
NO_PRUNING_POWER,
UNINDEXED_SEARCH_FIELDS,
UNSUPPORTED_SEARCH_PATTERN,
OPTIMIZED_WITH_MATERIALIZED_VIEW,
SECURED_BY_DATA_MASKING,
MISMATCHED_TEXT_ANALYZER,
BASE_TABLE_TOO_SMALL,
BASE_TABLE_TOO_LARGE,
ESTIMATED_PERFORMANCE_GAIN_TOO_LOW,
NOT_SUPPORTED_IN_STANDARD_EDITION,
INDEX_SUPPRESSED_BY_FUNCTION_OPTION,
QUERY_CACHE_HIT,
STALE_INDEX,
INTERNAL_ERROR,
OTHER_REASON,
)
def fromString(input: String): Either[String, IndexUnusedReasonCode] = values
.find(_.value == input)
.toRight(s"'$input' was not a valid value for IndexUnusedReasonCode")
implicit val decoder: Decoder[IndexUnusedReasonCode] = Decoder[String].emap(s => fromString(s))
implicit val encoder: Encoder[IndexUnusedReasonCode] = Encoder[String].contramap(_.value)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy