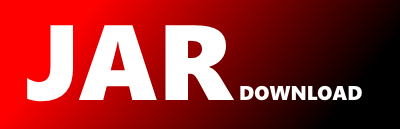
scala.googleapis.bigquery.Job.scala Maven / Gradle / Ivy
package googleapis.bigquery
import io.circe._
import io.circe.syntax._
final case class Job(
/** Output only. A hash of this resource.
*/
etag: Option[String] = None,
/** Output only. Information about the job, including starting time and ending time of the job.
*/
statistics: Option[JobStatistics] = None,
/** Output only. A URL that can be used to access the resource again.
*/
selfLink: Option[String] = None,
/** Output only. The reason why a Job was created. [Preview](https://cloud.google.com/products/#product-launch-stages)
*/
jobCreationReason: Option[JobCreationReason] = None,
/** Output only. Email address of the user who ran the job.
*/
user_email: Option[String] = None,
/** Output only. [Full-projection-only] String representation of identity of requesting party. Populated for both first- and third-party identities. Only present for APIs that support third-party identities.
*/
principal_subject: Option[String] = None,
/** Output only. Opaque ID field of the job.
*/
id: Option[String] = None,
/** Output only. The status of this job. Examine this value when polling an asynchronous job to see if the job is complete.
*/
status: Option[JobStatus] = None,
/** Required. Describes the job configuration.
*/
configuration: Option[JobConfiguration] = None,
/** Output only. The type of the resource.
*/
kind: Option[String] = None,
/** Optional. Reference describing the unique-per-user name of the job.
*/
jobReference: Option[JobReference] = None,
)
object Job {
implicit val encoder: Encoder[Job] = Encoder.instance { x =>
Json.obj(
"etag" := x.etag,
"statistics" := x.statistics,
"selfLink" := x.selfLink,
"jobCreationReason" := x.jobCreationReason,
"user_email" := x.user_email,
"principal_subject" := x.principal_subject,
"id" := x.id,
"status" := x.status,
"configuration" := x.configuration,
"kind" := x.kind,
"jobReference" := x.jobReference,
)
}
implicit val decoder: Decoder[Job] = Decoder.instance { c =>
for {
v0 <- c.get[Option[String]]("etag")
v1 <- c.get[Option[JobStatistics]]("statistics")
v2 <- c.get[Option[String]]("selfLink")
v3 <- c.get[Option[JobCreationReason]]("jobCreationReason")
v4 <- c.get[Option[String]]("user_email")
v5 <- c.get[Option[String]]("principal_subject")
v6 <- c.get[Option[String]]("id")
v7 <- c.get[Option[JobStatus]]("status")
v8 <- c.get[Option[JobConfiguration]]("configuration")
v9 <- c.get[Option[String]]("kind")
v10 <- c.get[Option[JobReference]]("jobReference")
} yield Job(v0, v1, v2, v3, v4, v5, v6, v7, v8, v9, v10)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy