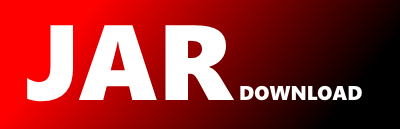
scala.googleapis.bigquery.JobStatistics2.scala Maven / Gradle / Ivy
package googleapis.bigquery
import JsonInstances._
import io.circe._
import io.circe.syntax._
import scala.concurrent.duration.FiniteDuration
final case class JobStatistics2(
/** Output only. The DDL target dataset. Present only for CREATE/ALTER/DROP SCHEMA(dataset) queries.
*/
ddlTargetDataset: Option[DatasetReference] = None,
/** Output only. [Beta] The DDL target routine. Present only for CREATE/DROP FUNCTION/PROCEDURE queries.
*/
ddlTargetRoutine: Option[RoutineReference] = None,
/** Output only. Statistics of metadata cache usage in a query for BigLake tables.
*/
metadataCacheStatistics: Option[MetadataCacheStatistics] = None,
/** Output only. Statistics of a Spark procedure job.
*/
sparkStatistics: Option[SparkStatistics] = None,
/** Output only. Performance insights.
*/
performanceInsights: Option[PerformanceInsights] = None,
/** Output only. Total bytes processed for the job.
*/
totalBytesProcessed: Option[Long] = None,
/** Output only. Statistics of materialized views of a query job.
*/
materializedViewStatistics: Option[MaterializedViewStatistics] = None,
/** Output only. The number of row access policies affected by a DDL statement. Present only for DROP ALL ROW ACCESS POLICIES queries.
*/
ddlAffectedRowAccessPolicyCount: Option[Long] = None,
/** Output only. The type of query statement, if valid. Possible values:
* `SELECT`: [`SELECT`](https://cloud.google.com/bigquery/docs/reference/standard-sql/query-syntax#select_list) statement.
* `ASSERT`: [`ASSERT`](https://cloud.google.com/bigquery/docs/reference/standard-sql/debugging-statements#assert) statement.
* `INSERT`: [`INSERT`](https://cloud.google.com/bigquery/docs/reference/standard-sql/dml-syntax#insert_statement) statement.
* `UPDATE`: [`UPDATE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/query-syntax#update_statement) statement.
* `DELETE`: [`DELETE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language) statement.
* `MERGE`: [`MERGE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-manipulation-language) statement.
* `CREATE_TABLE`: [`CREATE TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_table_statement) statement, without `AS SELECT`.
* `CREATE_TABLE_AS_SELECT`: [`CREATE TABLE AS SELECT`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#query_statement) statement.
* `CREATE_VIEW`: [`CREATE VIEW`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_view_statement) statement.
* `CREATE_MODEL`: [`CREATE MODEL`](https://cloud.google.com/bigquery-ml/docs/reference/standard-sql/bigqueryml-syntax-create#create_model_statement) statement.
* `CREATE_MATERIALIZED_VIEW`: [`CREATE MATERIALIZED VIEW`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_materialized_view_statement) statement.
* `CREATE_FUNCTION`: [`CREATE FUNCTION`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_function_statement) statement.
* `CREATE_TABLE_FUNCTION`: [`CREATE TABLE FUNCTION`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_table_function_statement) statement.
* `CREATE_PROCEDURE`: [`CREATE PROCEDURE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_procedure) statement.
* `CREATE_ROW_ACCESS_POLICY`: [`CREATE ROW ACCESS POLICY`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_row_access_policy_statement) statement.
* `CREATE_SCHEMA`: [`CREATE SCHEMA`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_schema_statement) statement.
* `CREATE_SNAPSHOT_TABLE`: [`CREATE SNAPSHOT TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_snapshot_table_statement) statement.
* `CREATE_SEARCH_INDEX`: [`CREATE SEARCH INDEX`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_search_index_statement) statement.
* `DROP_TABLE`: [`DROP TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_table_statement) statement.
* `DROP_EXTERNAL_TABLE`: [`DROP EXTERNAL TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_external_table_statement) statement.
* `DROP_VIEW`: [`DROP VIEW`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_view_statement) statement.
* `DROP_MODEL`: [`DROP MODEL`](https://cloud.google.com/bigquery-ml/docs/reference/standard-sql/bigqueryml-syntax-drop-model) statement.
* `DROP_MATERIALIZED_VIEW`: [`DROP MATERIALIZED VIEW`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_materialized_view_statement) statement.
* `DROP_FUNCTION` : [`DROP FUNCTION`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_function_statement) statement.
* `DROP_TABLE_FUNCTION` : [`DROP TABLE FUNCTION`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_table_function) statement.
* `DROP_PROCEDURE`: [`DROP PROCEDURE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_procedure_statement) statement.
* `DROP_SEARCH_INDEX`: [`DROP SEARCH INDEX`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_search_index) statement.
* `DROP_SCHEMA`: [`DROP SCHEMA`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_schema_statement) statement.
* `DROP_SNAPSHOT_TABLE`: [`DROP SNAPSHOT TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_snapshot_table_statement) statement.
* `DROP_ROW_ACCESS_POLICY`: [`DROP [ALL] ROW ACCESS POLICY|POLICIES`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#drop_row_access_policy_statement) statement.
* `ALTER_TABLE`: [`ALTER TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#alter_table_set_options_statement) statement.
* `ALTER_VIEW`: [`ALTER VIEW`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#alter_view_set_options_statement) statement.
* `ALTER_MATERIALIZED_VIEW`: [`ALTER MATERIALIZED VIEW`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#alter_materialized_view_set_options_statement) statement.
* `ALTER_SCHEMA`: [`ALTER SCHEMA`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#aalter_schema_set_options_statement) statement.
* `SCRIPT`: [`SCRIPT`](https://cloud.google.com/bigquery/docs/reference/standard-sql/procedural-language).
* `TRUNCATE_TABLE`: [`TRUNCATE TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/dml-syntax#truncate_table_statement) statement.
* `CREATE_EXTERNAL_TABLE`: [`CREATE EXTERNAL TABLE`](https://cloud.google.com/bigquery/docs/reference/standard-sql/data-definition-language#create_external_table_statement) statement.
* `EXPORT_DATA`: [`EXPORT DATA`](https://cloud.google.com/bigquery/docs/reference/standard-sql/other-statements#export_data_statement) statement.
* `EXPORT_MODEL`: [`EXPORT MODEL`](https://cloud.google.com/bigquery-ml/docs/reference/standard-sql/bigqueryml-syntax-export-model) statement.
* `LOAD_DATA`: [`LOAD DATA`](https://cloud.google.com/bigquery/docs/reference/standard-sql/other-statements#load_data_statement) statement.
* `CALL`: [`CALL`](https://cloud.google.com/bigquery/docs/reference/standard-sql/procedural-language#call) statement.
*/
statementType: Option[String] = None,
/** Output only. Whether the query result was fetched from the query cache.
*/
cacheHit: Option[Boolean] = None,
/** Output only. Describes execution plan for the query.
*/
queryPlan: Option[List[ExplainQueryStage]] = None,
/** Output only. Referenced routines for the job.
*/
referencedRoutines: Option[List[RoutineReference]] = None,
/** Output only. The DDL target row access policy. Present only for CREATE/DROP ROW ACCESS POLICY queries.
*/
ddlTargetRowAccessPolicy: Option[RowAccessPolicyReference] = None,
/** Deprecated.
*/
modelTrainingCurrentIteration: Option[Int] = None,
/** Output only. Billing tier for the job. This is a BigQuery-specific concept which is not related to the Google Cloud notion of "free tier". The value here is a measure of the query's resource consumption relative to the amount of data scanned. For on-demand queries, the limit is 100, and all queries within this limit are billed at the standard on-demand rates. On-demand queries that exceed this limit will fail with a billingTierLimitExceeded error.
*/
billingTier: Option[Int] = None,
/** Output only. Statistics for a LOAD query.
*/
loadQueryStatistics: Option[LoadQueryStatistics] = None,
/** Output only. BI Engine specific Statistics.
*/
biEngineStatistics: Option[BiEngineStatistics] = None,
/** Output only. Vector Search query specific statistics.
*/
vectorSearchStatistics: Option[VectorSearchStatistics] = None,
/** Output only. Job resource usage breakdown by reservation. This field reported misleading information and will no longer be populated.
*/
reservationUsage: Option[List[JobStatistics2ReservationUsage]] = None,
/** Output only. The schema of the results. Present only for successful dry run of non-legacy SQL queries.
*/
schema: Option[TableSchema] = None,
/** Output only. Referenced tables for the job. Queries that reference more than 50 tables will not have a complete list.
*/
referencedTables: Option[List[TableReference]] = None,
/** Output only. Detailed statistics for DML statements INSERT, UPDATE, DELETE, MERGE or TRUNCATE.
*/
dmlStats: Option[DmlStatistics] = None,
/** Output only. The number of rows affected by a DML statement. Present only for DML statements INSERT, UPDATE or DELETE.
*/
numDmlAffectedRows: Option[Long] = None,
/** Output only. If the project is configured to use on-demand pricing, then this field contains the total bytes billed for the job. If the project is configured to use flat-rate pricing, then you are not billed for bytes and this field is informational only.
*/
totalBytesBilled: Option[Long] = None,
/** Output only. Referenced view for DCL statement.
*/
dclTargetView: Option[TableReference] = None,
/** Output only. Slot-milliseconds for the job.
*/
totalSlotMs: Option[FiniteDuration] = None,
/** Output only. The original estimate of bytes processed for the job.
*/
estimatedBytesProcessed: Option[Long] = None,
/** Output only. Referenced table for DCL statement.
*/
dclTargetTable: Option[TableReference] = None,
/** Output only. Describes a timeline of job execution.
*/
timeline: Option[List[QueryTimelineSample]] = None,
/** Output only. Statistics of a BigQuery ML training job.
*/
mlStatistics: Option[MlStatistics] = None,
/** Deprecated.
*/
modelTraining: Option[BigQueryModelTraining] = None,
/** Output only. Job cost breakdown as bigquery internal cost and external service costs.
*/
externalServiceCosts: Option[List[ExternalServiceCost]] = None,
/** Output only. Total bytes transferred for cross-cloud queries such as Cross Cloud Transfer and CREATE TABLE AS SELECT (CTAS).
*/
transferredBytes: Option[Long] = None,
/** Output only. For dry-run jobs, totalBytesProcessed is an estimate and this field specifies the accuracy of the estimate. Possible values can be: UNKNOWN: accuracy of the estimate is unknown. PRECISE: estimate is precise. LOWER_BOUND: estimate is lower bound of what the query would cost. UPPER_BOUND: estimate is upper bound of what the query would cost.
*/
totalBytesProcessedAccuracy: Option[String] = None,
/** Output only. Referenced dataset for DCL statement.
*/
dclTargetDataset: Option[DatasetReference] = None,
/** Output only. Query optimization information for a QUERY job.
*/
queryInfo: Option[QueryInfo] = None,
/** Deprecated.
*/
modelTrainingExpectedTotalIteration: Option[Long] = None,
/** Output only. The DDL operation performed, possibly dependent on the pre-existence of the DDL target.
*/
ddlOperationPerformed: Option[String] = None,
/** Output only. Total number of partitions processed from all partitioned tables referenced in the job.
*/
totalPartitionsProcessed: Option[Long] = None,
/** Output only. Stats for EXPORT DATA statement.
*/
exportDataStatistics: Option[ExportDataStatistics] = None,
/** Output only. Search query specific statistics.
*/
searchStatistics: Option[SearchStatistics] = None,
/** Output only. GoogleSQL only: list of undeclared query parameters detected during a dry run validation.
*/
undeclaredQueryParameters: Option[List[QueryParameter]] = None,
/** Output only. The DDL target table. Present only for CREATE/DROP TABLE/VIEW and DROP ALL ROW ACCESS POLICIES queries.
*/
ddlTargetTable: Option[TableReference] = None,
/** Output only. The table after rename. Present only for ALTER TABLE RENAME TO query.
*/
ddlDestinationTable: Option[TableReference] = None,
)
object JobStatistics2 {
implicit val encoder: Encoder[JobStatistics2] = Encoder.instance { x =>
Json.obj(
"ddlTargetDataset" := x.ddlTargetDataset,
"ddlTargetRoutine" := x.ddlTargetRoutine,
"metadataCacheStatistics" := x.metadataCacheStatistics,
"sparkStatistics" := x.sparkStatistics,
"performanceInsights" := x.performanceInsights,
"totalBytesProcessed" := x.totalBytesProcessed,
"materializedViewStatistics" := x.materializedViewStatistics,
"ddlAffectedRowAccessPolicyCount" := x.ddlAffectedRowAccessPolicyCount,
"statementType" := x.statementType,
"cacheHit" := x.cacheHit,
"queryPlan"
:= x.queryPlan,
"referencedRoutines" := x.referencedRoutines,
"ddlTargetRowAccessPolicy" := x.ddlTargetRowAccessPolicy,
"modelTrainingCurrentIteration" := x.modelTrainingCurrentIteration,
"billingTier" := x.billingTier,
"loadQueryStatistics" := x.loadQueryStatistics,
"biEngineStatistics" := x.biEngineStatistics,
"vectorSearchStatistics" := x.vectorSearchStatistics,
"reservationUsage"
:= x.reservationUsage,
"schema" := x.schema,
"referencedTables" := x.referencedTables,
"dmlStats" := x.dmlStats,
"numDmlAffectedRows" := x.numDmlAffectedRows,
"totalBytesBilled" := x.totalBytesBilled,
"dclTargetView" := x.dclTargetView,
"totalSlotMs" := x.totalSlotMs,
"estimatedBytesProcessed" := x.estimatedBytesProcessed,
"dclTargetTable"
:= x.dclTargetTable,
"timeline" := x.timeline,
"mlStatistics" := x.mlStatistics,
"modelTraining" := x.modelTraining,
"externalServiceCosts"
:= x.externalServiceCosts,
"transferredBytes" := x.transferredBytes,
"totalBytesProcessedAccuracy" := x.totalBytesProcessedAccuracy,
"dclTargetDataset" := x.dclTargetDataset,
"queryInfo" := x.queryInfo,
"modelTrainingExpectedTotalIteration" := x.modelTrainingExpectedTotalIteration,
"ddlOperationPerformed" := x.ddlOperationPerformed,
"totalPartitionsProcessed" := x.totalPartitionsProcessed,
"exportDataStatistics" := x.exportDataStatistics,
"searchStatistics" := x.searchStatistics,
"undeclaredQueryParameters" := x.undeclaredQueryParameters,
"ddlTargetTable" := x.ddlTargetTable,
"ddlDestinationTable" := x.ddlDestinationTable,
)
}
implicit val decoder: Decoder[JobStatistics2] = Decoder.instance { c =>
for {
v0 <- c.get[Option[DatasetReference]]("ddlTargetDataset")
v1 <- c.get[Option[RoutineReference]]("ddlTargetRoutine")
v2 <- c.get[Option[MetadataCacheStatistics]]("metadataCacheStatistics")
v3 <- c.get[Option[SparkStatistics]]("sparkStatistics")
v4 <- c.get[Option[PerformanceInsights]]("performanceInsights")
v5 <- c.get[Option[Long]]("totalBytesProcessed")
v6 <- c.get[Option[MaterializedViewStatistics]]("materializedViewStatistics")
v7 <- c.get[Option[Long]]("ddlAffectedRowAccessPolicyCount")
v8 <- c.get[Option[String]]("statementType")
v9 <- c.get[Option[Boolean]]("cacheHit")
v10 <- c.get[Option[List[ExplainQueryStage]]]("queryPlan")
v11 <- c.get[Option[List[RoutineReference]]]("referencedRoutines")
v12 <- c.get[Option[RowAccessPolicyReference]]("ddlTargetRowAccessPolicy")
v13 <- c.get[Option[Int]]("modelTrainingCurrentIteration")
v14 <- c.get[Option[Int]]("billingTier")
v15 <- c.get[Option[LoadQueryStatistics]]("loadQueryStatistics")
v16 <- c.get[Option[BiEngineStatistics]]("biEngineStatistics")
v17 <- c.get[Option[VectorSearchStatistics]]("vectorSearchStatistics")
v18 <- c.get[Option[List[JobStatistics2ReservationUsage]]]("reservationUsage")
v19 <- c.get[Option[TableSchema]]("schema")
v20 <- c.get[Option[List[TableReference]]]("referencedTables")
v21 <- c.get[Option[DmlStatistics]]("dmlStats")
v22 <- c.get[Option[Long]]("numDmlAffectedRows")
v23 <- c.get[Option[Long]]("totalBytesBilled")
v24 <- c.get[Option[TableReference]]("dclTargetView")
v25 <- c.get[Option[FiniteDuration]]("totalSlotMs")
v26 <- c.get[Option[Long]]("estimatedBytesProcessed")
v27 <- c.get[Option[TableReference]]("dclTargetTable")
v28 <- c.get[Option[List[QueryTimelineSample]]]("timeline")
v29 <- c.get[Option[MlStatistics]]("mlStatistics")
v30 <- c.get[Option[BigQueryModelTraining]]("modelTraining")
v31 <- c.get[Option[List[ExternalServiceCost]]]("externalServiceCosts")
v32 <- c.get[Option[Long]]("transferredBytes")
v33 <- c.get[Option[String]]("totalBytesProcessedAccuracy")
v34 <- c.get[Option[DatasetReference]]("dclTargetDataset")
v35 <- c.get[Option[QueryInfo]]("queryInfo")
v36 <- c.get[Option[Long]]("modelTrainingExpectedTotalIteration")
v37 <- c.get[Option[String]]("ddlOperationPerformed")
v38 <- c.get[Option[Long]]("totalPartitionsProcessed")
v39 <- c.get[Option[ExportDataStatistics]]("exportDataStatistics")
v40 <- c.get[Option[SearchStatistics]]("searchStatistics")
v41 <- c.get[Option[List[QueryParameter]]]("undeclaredQueryParameters")
v42 <- c.get[Option[TableReference]]("ddlTargetTable")
v43 <- c.get[Option[TableReference]]("ddlDestinationTable")
} yield JobStatistics2(
v0,
v1,
v2,
v3,
v4,
v5,
v6,
v7,
v8,
v9,
v10,
v11,
v12,
v13,
v14,
v15,
v16,
v17,
v18,
v19,
v20,
v21,
v22,
v23,
v24,
v25,
v26,
v27,
v28,
v29,
v30,
v31,
v32,
v33,
v34,
v35,
v36,
v37,
v38,
v39,
v40,
v41,
v42,
v43,
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy