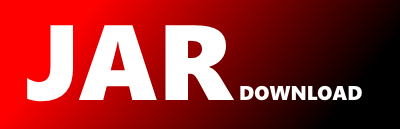
scala.googleapis.bigquery.RoutinesClient.scala Maven / Gradle / Ivy
package googleapis.bigquery
import cats.effect.Concurrent
import org.http4s._
import org.http4s.implicits._
import org.http4s.client.Client
class RoutinesClient[F[_]: Concurrent](client: Client[F]) extends AbstractClient[F](client) {
val baseUri = uri"https://bigquery.googleapis.com/bigquery/v2"
def insert(
/** Required. Project ID of the new routine
*/
projectId: String,
/** Required. Dataset ID of the new routine
*/
datasetId: String,
)(input: Routine): F[Routine] = expectJson[Routine](
requestWithBody(
method = Method.POST,
uri = baseUri / "projects" / s"${projectId}" / "datasets" / s"${datasetId}" / "routines",
)(input)
)
def setIamPolicy(
/** REQUIRED: The resource for which the policy is being specified. See [Resource names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
resource: String
)(input: SetIamPolicyRequest): F[Policy] =
expectJson[Policy](requestWithBody(method = Method.POST, uri = baseUri / s"${resource}")(input))
def getIamPolicy(
/** REQUIRED: The resource for which the policy is being requested. See [Resource names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this field.
*/
resource: String
)(input: GetIamPolicyRequest): F[Policy] =
expectJson[Policy](requestWithBody(method = Method.POST, uri = baseUri / s"${resource}")(input))
def delete(
/** Required. Project ID of the routine to delete
*/
projectId: String,
/** Required. Dataset ID of the routine to delete
*/
datasetId: String,
/** Required. Routine ID of the routine to delete
*/
routineId: String,
): F[Status] = client.status(
request(
method = Method.DELETE,
uri =
baseUri / "projects" / s"${projectId}" / "datasets" / s"${datasetId}" / "routines" / s"${routineId}",
)
)
def get(
/** Required. Project ID of the requested routine
*/
projectId: String,
/** Required. Dataset ID of the requested routine
*/
datasetId: String,
/** Required. Routine ID of the requested routine
*/
routineId: String,
query: RoutinesClient.GetParams = RoutinesClient.GetParams(),
): F[Routine] = expectJson[Routine](
request(
method = Method.GET,
uri =
(baseUri / "projects" / s"${projectId}" / "datasets" / s"${datasetId}" / "routines" / s"${routineId}")
.copy(query = Query.fromVector(Vector(List("readMask" -> query.readMask).flatMap {
case (k, v) => v.map(vv => k -> Option(vv))
}).flatten)),
)
)
def update(
/** Required. Project ID of the routine to update
*/
projectId: String,
/** Required. Dataset ID of the routine to update
*/
datasetId: String,
/** Required. Routine ID of the routine to update
*/
routineId: String,
)(input: Routine): F[Routine] = expectJson[Routine](
requestWithBody(
method = Method.PUT,
uri =
baseUri / "projects" / s"${projectId}" / "datasets" / s"${datasetId}" / "routines" / s"${routineId}",
)(input)
)
def list(
/** Required. Project ID of the routines to list
*/
projectId: String,
/** Required. Dataset ID of the routines to list
*/
datasetId: String,
query: RoutinesClient.ListParams = RoutinesClient.ListParams(),
): F[ListRoutinesResponse] = expectJson[ListRoutinesResponse](
request(
method = Method.GET,
uri = (baseUri / "projects" / s"${projectId}" / "datasets" / s"${datasetId}" / "routines")
.copy(query =
Query.fromVector(
Vector(
List("filter" -> query.filter).flatMap { case (k, v) =>
v.map(vv => k -> Option(vv))
},
List(
"maxResults" -> query.maxResults.map(s => QueryParamEncoder[Int].encode(s).value)
).flatMap { case (k, v) => v.map(vv => k -> Option(vv)) },
List("pageToken" -> query.pageToken).flatMap { case (k, v) =>
v.map(vv => k -> Option(vv))
},
List("readMask" -> query.readMask).flatMap { case (k, v) =>
v.map(vv => k -> Option(vv))
},
).flatten
)
),
)
)
}
object RoutinesClient {
final case class GetParams(
/** If set, only the Routine fields in the field mask are returned in the response. If unset, all Routine fields are returned.
*/
readMask: Option[String] = None
)
final case class ListParams(
/** If set, then only the Routines matching this filter are returned. The supported format is `routineType:{RoutineType}`, where `{RoutineType}` is a RoutineType enum. For example: `routineType:SCALAR_FUNCTION`.
*/
filter: Option[String] = None,
/** The maximum number of results to return in a single response page. Leverage the page tokens to iterate through the entire collection.
*/
maxResults: Option[Int] = None,
/** Page token, returned by a previous call, to request the next page of results
*/
pageToken: Option[String] = None,
/** If set, then only the Routine fields in the field mask, as well as project_id, dataset_id and routine_id, are returned in the response. If unset, then the following Routine fields are returned: etag, project_id, dataset_id, routine_id, routine_type, creation_time, last_modified_time, and language.
*/
readMask: Option[String] = None,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy