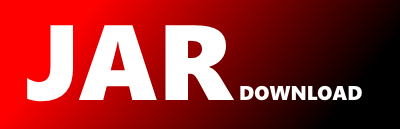
net.hamnaberg.json.codec.FieldDecoder Maven / Gradle / Ivy
package net.hamnaberg.json.codec;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.Callable;
import java.util.function.Function;
public final class FieldDecoder {
public final String name;
public final DecodeJson decoder;
private FieldDecoder(String name, DecodeJson decoder) {
this.name = name;
this.decoder = decoder;
}
@Override
public String toString() {
return String.format("FieldDecoder(%s)", name);
}
public FieldDecoder map(Function f) {
return typedFieldOf(name, decoder.map(f), Optional.empty());
}
public FieldDecoder flatMap(Function> f) {
DecodeJson bdecoder = json -> decoder.flatMap(a -> f.apply(a).decoder).fromJson(json);
return typedFieldOf(name, bdecoder, Optional.empty());
}
public FieldDecoder narrow(Function> f) {
return typedFieldOf(
name,
json -> decoder.tryMap(f).fromJson(json).fold(
err -> DecodeResult.fail(String.format("Decode for '%s' failed with %s", name, err)),
DecodeResult::ok
),
Optional.empty()
);
}
public FieldDecoder tryNarrow(Function f) {
return narrow(a -> () -> f.apply(a));
}
public FieldDecoder withDefaultValue(A defaultValue) {
return typedFieldOf(this.name, this.decoder, Optional.of(defaultValue));
}
public static FieldDecoder TString(String name) {
return typedFieldOf(name, Decoders.DString, Optional.empty());
}
public static FieldDecoder TInt(String name) {
return typedFieldOf(name, Decoders.DInt, Optional.empty());
}
public static FieldDecoder TDouble(String name) {
return typedFieldOf(name, Decoders.DDouble, Optional.empty());
}
public static FieldDecoder TLong(String name) {
return typedFieldOf(name, Decoders.DLong, Optional.empty());
}
public static FieldDecoder TBoolean(String name) {
return typedFieldOf(name, Decoders.DBoolean, Optional.empty());
}
public static FieldDecoder> TOptional(String name, DecodeJson decoder) {
return typedFieldOf(name, Decoders.optionalDecoder(decoder), Optional.of(Optional.empty()));
}
public static FieldDecoder> TList(String name, DecodeJson decoder) {
return typedFieldOf(name, Decoders.listDecoder(decoder));
}
public static FieldDecoder typedFieldOf(String name, DecodeJson decoder) {
return new FieldDecoder(name, decoder);
}
public static FieldDecoder typedFieldOf(String name, DecodeJson decoder, Optional defaultValue) {
return new FieldDecoder(name, defaultValue.map(decoder::withDefaultValue).orElse(decoder));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy