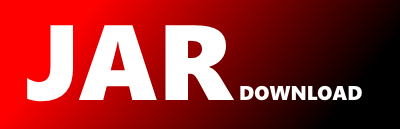
org.eigenbase.resgen.CppGenerator Maven / Gradle / Ivy
/*
// Licensed to Julian Hyde under one or more contributor license
// agreements. See the NOTICE file distributed with this work for
// additional information regarding copyright ownership.
//
// Julian Hyde licenses this file to you under the Apache License,
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at:
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
*/
package org.eigenbase.resgen;
import java.io.File;
import java.io.PrintWriter;
/**
* Generates a C++ class containing resource definitions.
*
* @author jhyde
*/
class CppGenerator extends AbstractGenerator
{
private final String className;
private final String defaultExceptionClassName;
private final String headerFilename;
private final String baseClassName;
private static final String CPP_STRING = "const std::string &";
private static final String CPP_NUMBER = "int";
private static final String CPP_DATE_TIME = "time_t";
private static final String[] CPP_TYPE_NAMES =
{CPP_STRING, CPP_NUMBER, CPP_DATE_TIME, CPP_DATE_TIME};
/**
* Creates a C++ header generator.
*
* @param srcFile Source file
* @param file File
* @param className Class name
* @param baseClassName Name of base class, must not be null, typically
* @param defaultExceptionClassName Default exception class name
*/
CppGenerator(
File srcFile,
File file,
String className,
String baseClassName,
String defaultExceptionClassName,
String headerFilename)
{
super(srcFile, file);
assert className.indexOf('.') < 0 :
"C++ class name must not contain '.': " + className;
this.className = className;
this.defaultExceptionClassName = defaultExceptionClassName;
this.headerFilename = headerFilename;
assert baseClassName != null;
this.baseClassName = baseClassName;
}
protected String getClassName()
{
return className;
}
protected String getBaseClassName()
{
return baseClassName;
}
protected String[] getArgTypes(String message) {
return ResourceDefinition.getArgTypes(message, CPP_TYPE_NAMES);
}
public void generateModule(ResourceGen generator, ResourceDef.ResourceBundle resourceList, PrintWriter pw)
{
generateDoNotModifyHeader(pw);
generateGeneratedByBlock(pw);
String className = getClassName();
String bundleCacheClassName = className + "BundleCache";
String baseClass = getBaseClassName();
if (resourceList.cppCommonInclude != null) {
pw.println(
"// begin common include specified by "
+ getSrcFileForComment());
pw.println("#include \"" + resourceList.cppCommonInclude + "\"");
pw.println(
"// end common include specified by "
+ getSrcFileForComment());
}
pw.println("#include \"" + headerFilename + "\"");
pw.println("#include \"ResourceBundle.h\"");
pw.println("#include \"Locale.h\"");
pw.println();
pw.println("#include
© 2015 - 2025 Weber Informatics LLC | Privacy Policy