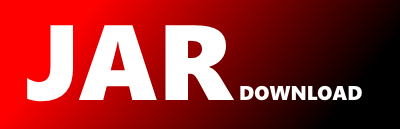
org.eigenbase.xom.XOMGenTask Maven / Gradle / Ivy
The newest version!
/*
// Licensed to Julian Hyde under one or more contributor license
// agreements. See the NOTICE file distributed with this work for
// additional information regarding copyright ownership.
//
// Julian Hyde licenses this file to you under the Apache License,
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at:
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
*/
package org.eigenbase.xom;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Task;
import java.io.File;
import java.io.IOException;
/**
* XOMGenTask
is an ANT task with which to invoke {@link
* MetaGenerator}.
*
* @author jhyde
*
*
*
* XOMGen
* Description
*
* Invokes the {@link MetaGenerator}.
*
*
* This task only invokes XOMGen if the grammar file is newer than the
* generated Java files.
*
*
* Parameters
*
*
* Attribute
* Description
* Required
*
*
* model
* The name of the XML file which holds the XOM
* model.
* Yes
*
*
* destdir
* The name of the output directory. Default is the
* current directory.
* No
*
*
* classname
* The full name of the class to generate.
* Yes
*
*
* dtdname
* The name of the DTD file to generate. The path may be
* either absolute, or relative to destdir
.
* Yes
*
*
*
* Example
* <xomgen
* model="src/org/eigenbase/xom/Meta.xml"
* destdir="src"
* classname="org.eigenbase.xom.MetaDef"/>
*
* This invokes XOMGen on the model file
* src/org/eigenbase/xom/Meta.xml
, and generates
* src/org/eigenbase/xom/MetaDef.java
and
* src/org/eigenbase/xom/meta.dtd
.
*
*
*
*/
public class XOMGenTask extends Task {
String modelFileName;
String destDir;
String dtdFileName;
String className;
public XOMGenTask()
{}
public void execute() throws BuildException {
try {
if (modelFileName == null) {
throw new BuildException("You must specify model.");
}
if (className == null) {
throw new BuildException("You must specify className.");
}
File projectBase = getProject().getBaseDir();
File destinationDirectory;
if (destDir == null) {
destinationDirectory = projectBase;
} else {
destinationDirectory = new File(projectBase, destDir);
}
if (!destinationDirectory.exists()) {
throw new BuildException(
"Destination directory doesn't exist: " +
destinationDirectory.toString());
}
File modelFile = new File(projectBase, modelFileName);
File classFile = classNameToFile(destinationDirectory, className);
File outputDir = classFile.getParentFile();
File dtdFile = new File(outputDir, dtdFileName);
if (modelFile.exists() &&
classFile.exists() &&
dtdFile.exists()) {
long modelStamp = modelFile.lastModified(),
classStamp = classFile.lastModified(),
dtdStamp = dtdFile.lastModified();
if (classStamp > modelStamp &&
dtdStamp > modelStamp) {
// files are up to date
return;
}
}
final boolean testMode = false;
MetaGenerator generator = new MetaGenerator(
modelFile.toString(), testMode, className);
generator.writeFiles(destinationDirectory.toString(), dtdFileName);
generator.writeOutputs();
} catch (XOMException e) {
throw new BuildException("Generation of model failed: " + e);
} catch (IOException e) {
throw new BuildException("Generation of model failed: " + e);
}
}
// ------------------------------------------------------------------------
// ANT attribute methods
/** See parameter model
. */
public void setModel(String model) {
this.modelFileName = model;
}
/** See parameter destdir
. */
public void setDestdir(String destdir) {
this.destDir = destdir;
}
/** See parameter classname
. */
public void setClassname(String classname) {
this.className = classname;
}
/** See parameter dtdname
. */
public void setDtdname(String dtdname) {
this.dtdFileName = dtdname;
}
// ------------------------------------------------------------------------
/**
* Creates the File that a java class will live in. For example,
* makeJavaFileName("com.myproj", "MyClass")
returns
* "com/myproj/MyClass.java".
*/
static File classNameToFile(File dir, String className) {
char fileSep = System.getProperty("file.separator").charAt(0); // e.g. '/'
String relativePath = className.replace('.', fileSep) + ".java";
if (dir == null) {
return new File(relativePath);
} else {
return new File(dir, relativePath);
}
}
}
// End XOMGenTask.java
© 2015 - 2025 Weber Informatics LLC | Privacy Policy