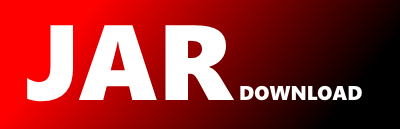
org.eigenbase.xom.package.html Maven / Gradle / Ivy
Package org.eigenbase.xom
Provides an object layer for reading and writing XML documents. The
XML-Object Mapping layer generates a class for each entity in an XML
schema, a member property for each attribute, collections for sub-objects, and
methods to serialize to and from XML.
Schema format
The schema is defined in an XML file, whose format is somewhat similar to an
XML Schema. The (self-describing) meta-schema is meta.xml
(
as you can see, an XSL style-sheet formats each schema into a javadoc-like web
page).
Other schemas include resource.xml
.
Generated Java Classes
The utilities in this package enable conversion of XML (stored in a DOM-style
model) into a typesafe Java representation. Roughly, conversion occurs as
follows:
- Each defined
Element
becomes a Java Class. The Java Class name matches the
Element name. All classes representing Elements descend from
{@link org.eigenbase.xom.ElementDef}. All classes from the same model are
implemented as static inner classes of a single enclosure class.
By convention, enclosure classes always end in "Def".
- Each
Attribute
becomes a public member of its enclosing Element class.
- Text (
PCDATA
) content becomes a single public String called cdata
.
- Element content becomes a series of public members. The members are of
the appropriate types. The members' names are defined in the schema.
ANY
content becomes a single array of {@link org.eigenbase.xom.ElementDef}
called children
.
Converting an XML Document to its XOM representation is a two-step process. First,
you parse the XML into a DOM representation using your favorite W3C DOM Level 1-compliant
parser (note: MSXML is supported as well for backward compatibility).
Then, you instantiate the XOM {@link org.eigenbase.xom.ElementDef} subclass
corresponding to the root element in the document, passing a portion of the DOM
as input to the constructor. After this, the fully-constructed root element
provides complete typesafe access to the whole document!
Specific instructions for parsing using a DOM-compliant parser (such as XERCES):
- Instantiate your parser, parse the document (validation against the DTD is optional),
and retrieve the parsed {@link org.w3c.dom.Document} object.
- Call {@link org.w3c.dom.Document#getDocumentElement()} to retrieve the toplevel Node
in the document.
- Wrap the toplevel node in an instance of
{@link org.eigenbase.xom.wrappers.W3CDOMWrapper}.
- Construct the appropriate {@link org.eigenbase.xom.ElementDef} subclass
from the wrapped node.
Specific instructions for parsing using Microsoft's XML Parser:
- Instantiate your parser, parse the document (validation against the DTD is optional),
and retrieve {@link com.ms.xml.om.Document} object representing the document.
- Call {@link com.ms.xml.om.Document#getRoot()} to retrieve the toplevel Element
in the document.
- Wrap the toplevel element in an instance of
{@link org.eigenbase.xom.wrappers.MSXMLWrapper}.
- Construct the appropriate {@link org.eigenbase.xom.ElementDef} subclass
from the wrapped node.
Generator
There is now an ANT target, XOMGen
, implemented by {@link org.eigenbase.xom.XOMGenTask}.
Tester
There is another helpful utility we use to verify that the stuff we generate
works. It is class {@link MetaTester}, and you invoke it as follows:
jview <classpath_options> org.eigenbase.xom.MetaTester [-debug]
[-msxml | -xerces] <XML model file> <output dir> [<tests>...]
All the arguments are the same as the ones for {@link org.eigenbase.xom.MetaGenerator}, except for
tests. <tests> is a list of test .xml files that should be valid
according to the generated DTD. The tester will validate the java files
against the DTD and also against the java class. It also runs some basic
checks on the java class to make sure that it works.
Generation
For all XML files except meta.xml
, we generate
corresponding DTD and NameDef.java
at build
time. {@link MetaDef} is tricky, because it depends upon itself;
use the ant all target to rebuild it.
Guidelines for writing models
- Note that within an
<Entity>
, all <Attribute>
s
must occur before all <Object>
, <Array>
or <CData>
elements.
Known Issues
Dependencies
This package is dependent upon the following other packages:
- {@link java.lang}, {@link java.io}, {@link java.util}
Class {@link XOMGenTask} only is dependent upon {@link
org.apache.tools.ant}. You therefore require ant.jar
to
build, but not to run.
Class {@link
org.eigenbase.xom.wrappers.XercesDOMParser} only is dependent
upon {@link org.xml.sax} and {@link
org.apache.xerces.parsers.DOMParser}. You therefore
require xerces.jar
to build, but not to run.