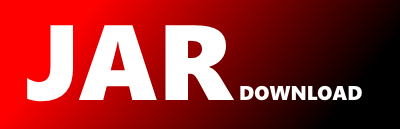
ij.io.Opener Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ij Show documentation
Show all versions of ij Show documentation
ImageJ is an open source Java image processing program inspired by NIH Image for the Macintosh.
package ij.io;
import ij.*;
import ij.gui.*;
import ij.process.*;
import ij.plugin.frame.*;
import ij.plugin.*;
import ij.text.TextWindow;
import ij.util.Java2;
import ij.measure.ResultsTable;
import ij.macro.Interpreter;
import ij.util.Tools;
import java.awt.*;
import java.awt.image.*;
import java.io.*;
import java.net.URL;
import java.net.*;
import java.util.*;
import java.util.zip.*;
import javax.swing.*;
import javax.swing.filechooser.*;
import java.awt.event.KeyEvent;
import javax.imageio.ImageIO;
import java.lang.reflect.Method;
/** Opens tiff (and tiff stacks), dicom, fits, pgm, jpeg, bmp or
gif images, and look-up tables, using a file open dialog or a path.
Calls HandleExtraFileTypes plugin if the file type is unrecognised. */
public class Opener {
public static final int UNKNOWN=0,TIFF=1,DICOM=2,FITS=3,PGM=4,JPEG=5,
GIF=6,LUT=7,BMP=8,ZIP=9,JAVA_OR_TEXT=10,ROI=11,TEXT=12,PNG=13,
TIFF_AND_DICOM=14,CUSTOM=15, AVI=16, OJJ=17, TABLE=18, RAW=19; // don't forget to also update 'types'
public static final String[] types = {"unknown","tif","dcm","fits","pgm",
"jpg","gif","lut","bmp","zip","java/txt","roi","txt","png","t&d","custom","ojj","table","raw"};
private static String defaultDirectory = null;
private int fileType;
private boolean error;
private boolean isRGB48;
private boolean silentMode;
private String omDirectory;
private File[] omFiles;
private static boolean openUsingPlugins;
private static boolean bioformats;
private String url;
static {
Hashtable commands = Menus.getCommands();
bioformats = commands!=null && commands.get("Bio-Formats Importer")!=null;
}
public Opener() {
}
/**
* Displays a file open dialog box and then opens the tiff, dicom,
* fits, pgm, jpeg, bmp, gif, lut, roi, or text file selected by
* the user. Displays an error message if the selected file is not
* in a supported format. This is the method that
* ImageJ's File/Open command uses to open files.
* @see ij.IJ#open()
* @see ij.IJ#open(String)
* @see ij.IJ#openImage()
* @see ij.IJ#openImage(String)
*/
public void open() {
OpenDialog od = new OpenDialog("Open", "");
String directory = od.getDirectory();
String name = od.getFileName();
if (name!=null) {
String path = directory+name;
error = false;
open(path);
if (!error) Menus.addOpenRecentItem(path);
}
}
/**
* Opens and displays the specified tiff, dicom, fits, pgm, jpeg,
* bmp, gif, lut, roi, or text file. Displays an error message if
* the file is not in a supported format.
* @see ij.IJ#open(String)
* @see ij.IJ#openImage(String)
*/
public void open(String path) {
boolean isURL = path.indexOf("://")>0;
if (isURL && isText(path)) {
openTextURL(path);
return;
}
if (path.endsWith(".jar") || path.endsWith(".class")) {
(new PluginInstaller()).install(path);
return;
}
boolean fullPath = path.startsWith("/") || path.startsWith("\\") || path.indexOf(":\\")==1 || path.indexOf(":/")==1 || isURL;
if (!fullPath) {
String defaultDir = OpenDialog.getDefaultDirectory();
if (defaultDir!=null)
path = defaultDir + path;
else
path = (new File(path)).getAbsolutePath();
}
if (!silentMode)
IJ.showStatus("Opening: " + path);
long start = System.currentTimeMillis();
ImagePlus imp = null;
if (path.endsWith(".txt"))
this.fileType = JAVA_OR_TEXT;
else
imp = openImage(path);
if (imp==null && isURL)
return;
if (imp!=null) {
WindowManager.checkForDuplicateName = true;
if (isRGB48)
openRGB48(imp);
else
imp.show(getLoadRate(start,imp));
} else {
switch (this.fileType) {
case LUT:
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.LutLoader", path);
if (imp.getWidth()!=0)
imp.show();
break;
case ROI:
IJ.runPlugIn("ij.plugin.RoiReader", path);
break;
case JAVA_OR_TEXT: case TEXT:
if (IJ.altKeyDown()) { // open in TextWindow if alt key down
new TextWindow(path,400,450);
IJ.setKeyUp(KeyEvent.VK_ALT);
break;
}
File file = new File(path);
int maxSize = 250000;
long size = file.length();
if (size>=28000) {
String osName = System.getProperty("os.name");
if (osName.equals("Windows 95") || osName.equals("Windows 98") || osName.equals("Windows Me"))
maxSize = 60000;
}
if (size64)
path = (new File(path)).getName();
if (path.length()<=64) {
if (IJ.redirectingErrorMessages())
msg += " \n "+path;
else
msg += " \n \n"+path;
}
}
if (openUsingPlugins)
msg += "\n \nNOTE: The \"OpenUsingPlugins\" option is set.";
IJ.wait(IJ.isMacro()?500:100); // work around for OS X thread deadlock problem
IJ.error("Opener", msg);
error = true;
break;
}
}
}
/** Displays a JFileChooser and then opens the tiff, dicom,
fits, pgm, jpeg, bmp, gif, lut, roi, or text files selected by
the user. Displays error messages if one or more of the selected
files is not in one of the supported formats. This is the method
that ImageJ's File/Open command uses to open files if
"Open/Save Using JFileChooser" is checked in EditOptions/Misc. */
public void openMultiple() {
Java2.setSystemLookAndFeel();
// run JFileChooser in a separate thread to avoid possible thread deadlocks
try {
EventQueue.invokeAndWait(new Runnable() {
public void run() {
JFileChooser fc = new JFileChooser();
fc.setMultiSelectionEnabled(true);
File dir = null;
String sdir = OpenDialog.getDefaultDirectory();
if (sdir!=null)
dir = new File(sdir);
if (dir!=null)
fc.setCurrentDirectory(dir);
if (IJ.debugMode) IJ.log("Opener.openMultiple: "+sdir+" "+dir);
int returnVal = fc.showOpenDialog(IJ.getInstance());
if (returnVal!=JFileChooser.APPROVE_OPTION)
return;
omFiles = fc.getSelectedFiles();
if (omFiles.length==0) { // getSelectedFiles does not work on some JVMs
omFiles = new File[1];
omFiles[0] = fc.getSelectedFile();
}
omDirectory = fc.getCurrentDirectory().getPath()+File.separator;
}
});
} catch (Exception e) {}
if (omDirectory==null) return;
OpenDialog.setDefaultDirectory(omDirectory);
for (int i=0; i0)
img = openURL(path);
else
img = openImage(getDir(path), getName(path));
return img;
}
/**
* Open the nth image of the specified tiff stack.
* @see ij.IJ#openImage(String,int)
*/
public ImagePlus openImage(String path, int n) {
if (path==null || path.equals(""))
path = getPath();
if (path==null) return null;
int type = getFileType(path);
if (type!=TIFF)
throw new IllegalArgumentException("TIFF file require");
return openTiff(path, n);
}
public static String getLoadRate(double time, ImagePlus imp) {
time = (System.currentTimeMillis()-time)/1000.0;
double mb = imp.getWidth()*imp.getHeight()*imp.getStackSize();
int bits = imp.getBitDepth();
if (bits==16)
mb *= 2;
else if (bits==24 || bits==32)
mb *=4;
mb /= 1024*1024;
double rate = mb/time;
int digits = rate<100.0?1:0;
return ""+IJ.d2s(time,2)+" seconds ("+IJ.d2s(mb/time,digits)+" MB/sec)";
}
private boolean isText(String path) {
if (path.endsWith(".txt") || path.endsWith(".ijm") || path.endsWith(".java")
|| path.endsWith(".js") || path.endsWith(".html") || path.endsWith(".htm")
|| path.endsWith(".bsh") || path.endsWith(".py") || path.endsWith("/"))
return true;
int lastSlash = path.lastIndexOf("/");
if (lastSlash==-1) lastSlash = 0;
int lastDot = path.lastIndexOf(".");
if (lastDot==-1 || lastDot6)
return true; // no extension
else
return false;
}
/** Opens the specified file and adds it to the File/Open Recent menu.
Returns true if the file was opened successfully. */
public boolean openAndAddToRecent(String path) {
open(path);
if (!error)
Menus.addOpenRecentItem(path);
return error;
}
/**
* Attempts to open the specified file as a tiff, bmp, dicom, fits,
* pgm, gif or jpeg image. Returns an ImagePlus object if successful.
* Modified by Gregory Jefferis to call HandleExtraFileTypes plugin if
* the file type is unrecognised.
* @see ij.IJ#openImage(String)
*/
public ImagePlus openImage(String directory, String name) {
ImagePlus imp;
FileOpener.setSilentMode(silentMode);
if (directory.length()>0 && !(directory.endsWith("/")||directory.endsWith("\\")))
directory += Prefs.separator;
OpenDialog.setLastDirectory(directory);
OpenDialog.setLastName(name);
String path = directory+name;
this.fileType = getFileType(path);
if (IJ.debugMode) IJ.log("openImage: \""+types[this.fileType]+"\", "+path);
switch (this.fileType) {
case TIFF:
imp = openTiff(directory, name);
return imp;
case DICOM: case TIFF_AND_DICOM:
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.DICOM", path);
if (imp.getWidth()!=0) return imp; else return null;
case FITS:
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.FITS_Reader", path);
if (imp.getWidth()!=0) return imp; else return null;
case PGM:
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.PGM_Reader", path);
if (imp.getWidth()!=0) {
if (imp.getStackSize()==3 && imp.getBitDepth()==16)
imp = new CompositeImage(imp, IJ.COMPOSITE);
return imp;
} else
return null;
case JPEG:
imp = openJpegOrGif(directory, name);
if (imp!=null&&imp.getWidth()!=0) return imp; else return null;
case GIF:
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.GIF_Reader", path);
if (imp!=null&&imp.getWidth()!=0) return imp; else return null;
case PNG:
imp = openUsingImageIO(directory+name);
if (imp!=null&&imp.getWidth()!=0) return imp; else return null;
case BMP:
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.BMP_Reader", path);
if (imp.getWidth()!=0) return imp; else return null;
case ZIP:
return openZip(path);
case AVI:
AVI_Reader reader = new AVI_Reader();
reader.setVirtual(true);
reader.displayDialog(!IJ.macroRunning());
reader.run(path);
return reader.getImagePlus();
case JAVA_OR_TEXT:
if (name.endsWith(".txt"))
return openTextImage(directory,name);
else
return null;
case UNKNOWN: case TEXT:
return openUsingHandleExtraFileTypes(path);
default:
return null;
}
}
// Call HandleExtraFileTypes plugin to see if it can handle unknown formats
// or files in TIFF format that the built in reader is unable to open.
private ImagePlus openUsingHandleExtraFileTypes(String path) {
int[] wrap = new int[] {this.fileType};
ImagePlus imp = openWithHandleExtraFileTypes(path, wrap);
if (imp!=null && imp.getNChannels()>1)
imp = new CompositeImage(imp, IJ.COLOR);
this.fileType = wrap[0];
if (imp==null && !silentMode && (this.fileType==UNKNOWN||this.fileType==TIFF))
IJ.error("Opener", "Unsupported format or file not found:\n"+path);
return imp;
}
String getPath() {
OpenDialog od = new OpenDialog("Open", "");
String dir = od.getDirectory();
String name = od.getFileName();
if (name==null)
return null;
else
return dir+name;
}
/** Opens the specified text file as a float image. */
public ImagePlus openTextImage(String dir, String name) {
String path = dir+name;
TextReader tr = new TextReader();
ImageProcessor ip = tr.open(path);
return ip!=null?new ImagePlus(name,ip):null;
}
/**
* Attempts to open the specified url as a tiff, zip compressed tiff,
* dicom, gif or jpeg. Tiff file names must end in ".tif", ZIP file names
* must end in ".zip" and dicom file names must end in ".dcm". Returns an
* ImagePlus object if successful.
* @see ij.IJ#openImage(String)
*/
public ImagePlus openURL(String url) {
url = updateUrl(url);
if (IJ.debugMode) IJ.log("OpenURL: "+url);
ImagePlus imp = openCachedImage(url);
if (imp!=null)
return imp;
try {
String name = "";
int index = url.lastIndexOf('/');
if (index==-1)
index = url.lastIndexOf('\\');
if (index>0)
name = url.substring(index+1);
else
throw new MalformedURLException("Invalid URL: "+url);
if (url.indexOf(" ")!=-1)
url = url.replaceAll(" ", "%20");
URL u = new URL(url);
IJ.showStatus(""+url);
String lurl = url.toLowerCase(Locale.US);
if (lurl.endsWith(".tif")) {
this.url = url;
imp = openTiff(u.openStream(), name);
} else if (lurl.endsWith(".zip"))
imp = openZipUsingUrl(u);
else if (lurl.endsWith(".jpg") || lurl.endsWith(".gif"))
imp = openJpegOrGifUsingURL(name, u);
else if (lurl.endsWith(".dcm") || lurl.endsWith(".ima")) {
imp = (ImagePlus)IJ.runPlugIn("ij.plugin.DICOM", url);
if (imp!=null && imp.getWidth()==0) imp = null;
} else if (lurl.endsWith(".png"))
imp = openPngUsingURL(name, u);
else {
URLConnection uc = u.openConnection();
String type = uc.getContentType();
if (type!=null && (type.equals("image/jpeg")||type.equals("image/gif")))
imp = openJpegOrGifUsingURL(name, u);
else if (type!=null && type.equals("image/png"))
imp = openPngUsingURL(name, u);
else
imp = openWithHandleExtraFileTypes(url, new int[]{0});
}
IJ.showStatus("");
return imp;
} catch (Exception e) {
String msg = e.getMessage();
if (msg==null || msg.equals(""))
msg = "" + e;
IJ.error("Open URL", msg);
return null;
}
}
/** Can't open imagej.nih.gov URLs due to encryption so redirect to imagej.net mirror. */
public static String updateUrl(String url) {
if (url==null || !url.contains("nih.gov"))
return url;
if (IJ.isJava18())
url = url.replace("http:", "https:");
else {
url = url.replace("imagej.nih.gov/ij", "imagej.net");
url = url.replace("rsb.info.nih.gov/ij", "imagej.net");
url = url.replace("rsbweb.nih.gov/ij", "imagej.net");
}
return url;
}
private ImagePlus openCachedImage(String url) {
if (url==null || !url.contains("/images"))
return null;
String ijDir = IJ.getDirectory("imagej");
if (ijDir==null)
return null;
int slash = url.lastIndexOf('/');
File file = new File(ijDir + "samples", url.substring(slash+1));
if (!file.exists())
return null;
if (url.endsWith(".gif")) // ij.plugin.GIF_Reader does not correctly handle inverting LUTs
return openJpegOrGif(file.getParent()+File.separator, file.getName());
return IJ.openImage(file.getPath());
}
/** Used by open() and IJ.open() to open text URLs. */
void openTextURL(String url) {
if (url.endsWith(".pdf")||url.endsWith(".zip"))
return;
String text = IJ.openUrlAsString(url);
if (text!=null && text.startsWith("0 && imp.getHeight()>0) {
fileTypes[0] = CUSTOM;
return imp;
} else {
if (imp.getWidth()==-1)
fileTypes[0] = CUSTOM; // plugin opened image so don't display error
return null;
}
}
/** Opens the ZIP compressed TIFF or DICOM at the specified URL. */
ImagePlus openZipUsingUrl(URL url) throws IOException {
URLConnection uc = url.openConnection();
InputStream in = uc.getInputStream();
ZipInputStream zis = new ZipInputStream(in);
ZipEntry entry = zis.getNextEntry();
if (entry==null) {
zis.close();
return null;
}
String name = entry.getName();
if (!(name.endsWith(".tif")||name.endsWith(".dcm")))
throw new IOException("This ZIP archive does not appear to contain a .tif or .dcm file\n"+name);
if (name.endsWith(".dcm"))
return openDicomStack(zis, entry);
else
return openTiff(zis, name);
}
ImagePlus openDicomStack(ZipInputStream zis, ZipEntry entry) throws IOException {
ImagePlus imp = null;
int count = 0;
ImageStack stack = null;
while (true) {
if (count>0) entry = zis.getNextEntry();
if (entry==null) break;
String name = entry.getName();
ImagePlus imp2 = null;
if (name.endsWith(".dcm")) {
ByteArrayOutputStream out = new ByteArrayOutputStream();
byte[] buf = new byte[4096];
int len, byteCount=0, progress=0;
while (true) {
len = zis.read(buf);
if (len<0) break;
out.write(buf, 0, len);
byteCount += len;
//IJ.showProgress((double)(byteCount%fileSize)/fileSize);
}
byte[] bytes = out.toByteArray();
out.close();
InputStream is = new ByteArrayInputStream(bytes);
DICOM dcm = new DICOM(is);
dcm.run(name);
imp2 = dcm;
is.close();
}
zis.closeEntry();
if (imp2==null) continue;
count++;
String label = imp2.getTitle();
String info = (String)imp2.getProperty("Info");
if (info!=null) label += "\n" + info;
if (count==1) {
imp = imp2;
imp.getStack().setSliceLabel(label, 1);
} else {
stack = imp.getStack();
stack.addSlice(label, imp2.getProcessor());
imp.setStack(stack);
}
}
zis.close();
IJ.showProgress(1.0);
if (count==0)
throw new IOException("This ZIP archive does not appear to contain any .dcm files");
return imp;
}
ImagePlus openJpegOrGifUsingURL(String title, URL url) {
if (url==null) return null;
Image img = Toolkit.getDefaultToolkit().createImage(url);
if (img!=null) {
ImagePlus imp = new ImagePlus(title, img);
return imp;
} else
return null;
}
ImagePlus openPngUsingURL(String title, URL url) {
if (url==null)
return null;
Image img = null;
try {
InputStream in = url.openStream();
img = ImageIO.read(in);
} catch (FileNotFoundException e) {
IJ.error("Open PNG Using URL", ""+e);
} catch (IOException e) {
IJ.handleException(e);
}
if (img!=null) {
ImagePlus imp = new ImagePlus(title, img);
return imp;
} else
return null;
}
ImagePlus openJpegOrGif(String dir, String name) {
ImagePlus imp = null;
Image img = Toolkit.getDefaultToolkit().createImage(dir+name);
if (img!=null) {
try {
imp = new ImagePlus(name, img);
} catch (Exception e) {
IJ.error("Opener", e.getMessage()+"\n(Note: ImageJ cannot open CMYK JPEGs)\n \n"+dir+name);
return null; // error loading image
}
if (imp.getType()==ImagePlus.COLOR_RGB)
convertGrayJpegTo8Bits(imp);
FileInfo fi = new FileInfo();
fi.fileFormat = fi.GIF_OR_JPG;
fi.fileName = name;
fi.directory = dir;
imp.setFileInfo(fi);
}
return imp;
}
ImagePlus openUsingImageIO(String path) {
ImagePlus imp = null;
BufferedImage img = null;
File f = new File(path);
try {
img = ImageIO.read(f);
} catch (Exception e) {
IJ.error("Open Using ImageIO", ""+e);
}
if (img==null)
return null;
if (img.getColorModel().hasAlpha()) {
int width = img.getWidth();
int height = img.getHeight();
BufferedImage bi = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics g = bi.getGraphics();
g.setColor(Color.white);
g.fillRect(0,0,width,height);
g.drawImage(img, 0, 0, null);
img = bi;
}
imp = new ImagePlus(f.getName(), img);
FileInfo fi = new FileInfo();
fi.fileFormat = fi.IMAGEIO;
fi.fileName = f.getName();
String parent = f.getParent();
if (parent!=null)
fi.directory = parent + File.separator;
imp.setFileInfo(fi);
return imp;
}
/** Converts the specified RGB image to 8-bits if the 3 channels are identical. */
public static void convertGrayJpegTo8Bits(ImagePlus imp) {
ImageProcessor ip = imp.getProcessor();
if (ip.getBitDepth()==24 && ip.isGrayscale()) {
IJ.showStatus("Converting to 8-bit grayscale");
new ImageConverter(imp).convertToGray8();
}
}
/** Are all the images in this file the same size and type? */
boolean allSameSizeAndType(FileInfo[] info) {
boolean sameSizeAndType = true;
boolean contiguous = true;
long startingOffset = info[0].getOffset();
int size = info[0].width*info[0].height*info[0].getBytesPerPixel();
for (int i=1; i1 && !allSameSizeAndType(info))
return null;
FileInfo fi = info[0];
if (fi.nImages>1)
return new FileOpener(fi).openImage(); // open contiguous images as stack
else {
ColorModel cm = createColorModel(fi);
ImageStack stack = new ImageStack(fi.width, fi.height, cm);
Object pixels = null;
long skip = fi.getOffset();
int imageSize = fi.width*fi.height*fi.getBytesPerPixel();
if (info[0].fileType==FileInfo.GRAY12_UNSIGNED) {
imageSize = (int)(fi.width*fi.height*1.5);
if ((imageSize&1)==1) imageSize++; // add 1 if odd
} if (info[0].fileType==FileInfo.BITMAP) {
int scan=(int)Math.ceil(fi.width/8.0);
imageSize = scan*fi.height;
}
long loc = 0L;
int nChannels = 1;
try {
InputStream is = createInputStream(fi);
ImageReader reader = new ImageReader(fi);
IJ.resetEscape();
for (int i=0; i1 && !(bpp==3||bpp==4||bpp==6)) {
nChannels = fi.samplesPerPixel;
channels = new Object[nChannels];
for (int c=0; c=FileInfo.LZW) skip = 0;
if (skip<0L) {
IJ.error("Opener", "Unexpected image offset");
break;
}
}
if (fi.fileType==FileInfo.RGB48) {
Object[] pixels2 = (Object[])pixels;
stack.addSlice(null, pixels2[0]);
stack.addSlice(null, pixels2[1]);
stack.addSlice(null, pixels2[2]);
isRGB48 = true;
} else if (nChannels>1) {
for (int c=0; c1 && (stackSize%nChannels)==0) {
imp.setDimensions(nChannels, stackSize/nChannels, 1);
imp = new CompositeImage(imp, IJ.COMPOSITE);
imp.setOpenAsHyperStack(true);
} else if (imp.getNChannels()>1)
imp = makeComposite(imp, fi);
IJ.showProgress(1.0);
return imp;
}
}
/** Attempts to open the specified file as a tiff.
Returns an ImagePlus object if successful. */
public ImagePlus openTiff(String directory, String name) {
TiffDecoder td = new TiffDecoder(directory, name);
if (IJ.debugMode) td.enableDebugging();
FileInfo[] info=null;
try {
info = td.getTiffInfo();
} catch (IOException e) {
this.fileType = TIFF;
return openUsingHandleExtraFileTypes(directory+name);
}
if (info==null)
return null;
return openTiff2(info);
}
/** Opens the nth image of the specified TIFF stack. */
public ImagePlus openTiff(String path, int n) {
TiffDecoder td = new TiffDecoder(getDir(path), getName(path));
if (IJ.debugMode) td.enableDebugging();
FileInfo[] info=null;
try {
info = td.getTiffInfo();
} catch (IOException e) {
String msg = e.getMessage();
if (msg==null||msg.equals("")) msg = ""+e;
IJ.error("Open TIFF", msg);
return null;
}
if (info==null) return null;
FileInfo fi = info[0];
if (info.length==1 && fi.nImages>1) {
if (n<1 || n>fi.nImages)
throw new IllegalArgumentException("N out of 1-"+fi.nImages+" range");
long size = fi.width*fi.height*fi.getBytesPerPixel();
fi.longOffset = fi.getOffset() + (n-1)*(size+fi.getGap());
fi.offset = 0;
fi.nImages = 1;
} else {
if (n<1 || n>info.length)
throw new IllegalArgumentException("N out of 1-"+info.length+" range");
fi.longOffset = info[n-1].getOffset();
fi.offset = 0;
fi.stripOffsets = info[n-1].stripOffsets;
fi.stripLengths = info[n-1].stripLengths;
}
FileOpener fo = new FileOpener(fi);
return fo.openImage();
}
/** Returns the FileInfo of the specified TIFF file. */
public static FileInfo[] getTiffFileInfo(String path) {
Opener o = new Opener();
TiffDecoder td = new TiffDecoder(o.getDir(path), o.getName(path));
if (IJ.debugMode) td.enableDebugging();
try {
return td.getTiffInfo();
} catch (IOException e) {
return null;
}
}
/** Attempts to open the specified inputStream as a
TIFF, returning an ImagePlus object if successful. */
public ImagePlus openTiff(InputStream in, String name) {
FileInfo[] info = null;
try {
TiffDecoder td = new TiffDecoder(in, name);
if (IJ.debugMode) td.enableDebugging();
info = td.getTiffInfo();
} catch (FileNotFoundException e) {
IJ.error("Open TIFF", "File not found: "+e.getMessage());
return null;
} catch (Exception e) {
IJ.error("Open TIFF", ""+e);
return null;
}
if (url!=null && info!=null && info.length==1 && info[0].inputStream!=null) {
try {
info[0].inputStream.close();
} catch (IOException e) {}
try {
info[0].inputStream = new URL(url).openStream();
} catch (Exception e) {
IJ.error("Open TIFF", ""+e);
return null;
}
}
return openTiff2(info);
}
/** Opens a single TIFF or DICOM contained in a ZIP archive,
or a ZIPed collection of ".roi" files created by the ROI manager. */
public ImagePlus openZip(String path) {
ImagePlus imp = null;
try {
ZipInputStream zis = new ZipInputStream(new FileInputStream(path));
ZipEntry entry = zis.getNextEntry();
if (entry==null) {
zis.close();
return null;
}
String name = entry.getName();
if (name.endsWith(".roi")) {
zis.close();
if (!silentMode)
if (IJ.isMacro() && Interpreter.isBatchMode() && RoiManager.getInstance()==null)
IJ.log("Use roiManager(\"Open\", path) instead of open(path)\nto open ROI sets in batch mode macros.");
else
IJ.runMacro("roiManager(\"Open\", getArgument());", path);
return null;
}
if (name.endsWith(".tif")) {
imp = openTiff(zis, name);
} else if (name.endsWith(".dcm")) {
DICOM dcm = new DICOM(zis);
dcm.run(name);
imp = dcm;
} else {
zis.close();
String msg = "This ZIP archive does not contain a TIFF or DICOM file, or ROIs:\n "+path;
if (silentMode)
IJ.log(msg);
else
IJ.error("Opener", msg);
return null;
}
zis.close();
} catch (Exception e) {
IJ.error("Opener", ""+e);
return null;
}
File f = new File(path);
FileInfo fi = imp.getOriginalFileInfo();
if (fi!=null) {
fi.fileFormat = FileInfo.ZIP_ARCHIVE;
fi.fileName = f.getName();
String parent = f.getParent();
if (parent!=null)
fi.directory = parent+File.separator;
}
return imp;
}
/** Deserialize a byte array that was serialized using the FileSaver.serialize(). */
public ImagePlus deserialize(byte[] bytes) {
ByteArrayInputStream stream = new ByteArrayInputStream(bytes);
TiffDecoder decoder = new TiffDecoder(stream, "Untitled");
if (IJ.debugMode)
decoder.enableDebugging();
FileInfo[] info = null;
try {
info = decoder.getTiffInfo();
} catch (IOException e) {
return null;
}
FileOpener opener = new FileOpener(info[0]);
ImagePlus imp = opener.openImage();
if (imp==null)
return null;
imp.setTitle(info[0].fileName);
imp = makeComposite(imp, info[0]);
return imp;
}
private ImagePlus makeComposite(ImagePlus imp, FileInfo fi) {
int c = imp.getNChannels();
boolean composite = c>1 && fi.description!=null && fi.description.indexOf("mode=")!=-1;
if (c>1 && (imp.getOpenAsHyperStack()||composite) && !imp.isComposite() && imp.getType()!=ImagePlus.COLOR_RGB) {
int mode = IJ.COLOR;
if (fi.description!=null) {
if (fi.description.indexOf("mode=composite")!=-1)
mode = IJ.COMPOSITE;
else if (fi.description.indexOf("mode=gray")!=-1)
mode = IJ.GRAYSCALE;
}
imp = new CompositeImage(imp, mode);
}
return imp;
}
public String getName(String path) {
int i = path.lastIndexOf('/');
if (i==-1)
i = path.lastIndexOf('\\');
if (i>0)
return path.substring(i+1);
else
return path;
}
public String getDir(String path) {
int i = path.lastIndexOf('/');
if (i==-1)
i = path.lastIndexOf('\\');
if (i>0)
return path.substring(0, i+1);
else
return "";
}
ImagePlus openTiff2(FileInfo[] info) {
if (info==null)
return null;
ImagePlus imp = null;
if (IJ.debugMode) // dump tiff tags
IJ.log(info[0].debugInfo);
if (info.length>1) { // try to open as stack
imp = openTiffStack(info);
if (imp!=null)
return imp;
}
FileOpener fo = new FileOpener(info[0]);
imp = fo.openImage();
if (imp==null)
return null;
int[] offsets = info[0].stripOffsets;
if (offsets!=null&&offsets.length>1 && offsets[offsets.length-1]126) {
isText = false;
break;
}
}
if (isText)
return TEXT;
// BMP ("BM")
if ((b0==66 && b1==77)||name.endsWith(".dib"))
return BMP;
// RAW
if (name.endsWith(".raw") && !Prefs.skipRawDialog)
return RAW;
return UNKNOWN;
}
/** Returns an IndexColorModel for the image specified by this FileInfo. */
ColorModel createColorModel(FileInfo fi) {
if (fi.lutSize>0)
return new IndexColorModel(8, fi.lutSize, fi.reds, fi.greens, fi.blues);
else
return LookUpTable.createGrayscaleColorModel(fi.whiteIsZero);
}
/** Returns an InputStream for the image described by this FileInfo. */
InputStream createInputStream(FileInfo fi) throws IOException, MalformedURLException {
if (fi.inputStream!=null)
return fi.inputStream;
else if (fi.url!=null && !fi.url.equals(""))
return new URL(fi.url+fi.fileName).openStream();
else {
File f = new File(fi.getFilePath());
if (f==null || f.isDirectory())
return null;
else {
InputStream is = new FileInputStream(f);
if (fi.compression>=FileInfo.LZW || (fi.stripOffsets!=null&&fi.stripOffsets.length>1))
is = new RandomAccessStream(is);
return is;
}
}
}
void openRGB48(ImagePlus imp) {
isRGB48 = false;
int stackSize = imp.getStackSize();
imp.setDimensions(3, stackSize/3, 1);
imp = new CompositeImage(imp, IJ.COMPOSITE);
imp.show();
}
/** The "Opening: path" status message is not displayed in silent mode. */
public void setSilentMode(boolean mode) {
silentMode = mode;
}
/** Open all images using HandleExtraFileTypes. Set from
a macro using setOption("openUsingPlugins", true). */
public static void setOpenUsingPlugins(boolean b) {
openUsingPlugins = b;
}
/** Returns the state of the openUsingPlugins flag. */
public static boolean getOpenUsingPlugins() {
return openUsingPlugins;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy