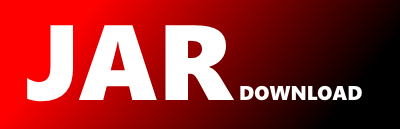
ij.process.FloatPolygon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ij Show documentation
Show all versions of ij Show documentation
ImageJ is an open source Java image processing program inspired by NIH Image for the Macintosh.
package ij.process;
import java.awt.Rectangle;
import java.awt.Polygon;
import java.awt.geom.Rectangle2D;
/** Used by the Roi classes to return float coordinate arrays and to
determine if a point is inside or outside of spline fitted selections. */
public class FloatPolygon {
private Rectangle bounds;
private float minX, minY, maxX, maxY;
/** The number of points. */
public int npoints;
/* The array of x coordinates. */
public float xpoints[];
/* The array of y coordinates. */
public float ypoints[];
/** Constructs an empty FloatPolygon. */
public FloatPolygon() {
npoints = 0;
xpoints = new float[10];
ypoints = new float[10];
}
/** Constructs a FloatPolygon from x and y arrays. */
public FloatPolygon(float xpoints[], float ypoints[]) {
if (xpoints.length!=ypoints.length)
throw new IllegalArgumentException("xpoints.length!=ypoints.length");
this.npoints = xpoints.length;
this.xpoints = xpoints;
this.ypoints = ypoints;
}
/** Constructs a FloatPolygon from x and y arrays. */
public FloatPolygon(float xpoints[], float ypoints[], int npoints) {
this.npoints = npoints;
this.xpoints = xpoints;
this.ypoints = ypoints;
}
/** Returns 'true' if the point (x,y) is inside this polygon. This is a Java
* version of the remarkably small C program by W. Randolph Franklin at
* http://www.ecse.rpi.edu/Homepages/wrf/Research/Short_Notes/pnpoly.html#The%20C%20Code
*
* In the absence of numerical errors, x coordinates exactly at a boundary are taken as if
* in the area immediately at the left of the boundary. For horizontal boundaries,
* y coordinates exactly at the border are taken as if in the area immediately above it
* (i.e., in decreasing y direction).
* The ImageJ convention of an offset of 0.5 pixels between pixel centers and outline
* coordinates is not taken into consideration; this method returns the purely
* geometrical relationship.
*/
public boolean contains(double x, double y) {
boolean inside = false;
for (int i=0, j=npoints-1; i=y)!=(ypoints[j]>=y)) &&
(x>((double)xpoints[j]-xpoints[i])*((double)y-ypoints[i])/((double)ypoints[j]-ypoints[i])+(double)xpoints[i]))
inside = !inside;
}
return inside;
}
/** A version of contains() that accepts float arguments. */
public boolean contains(float x, float y) {
return contains((double)x, (double)y);
}
public Rectangle getBounds() {
if (npoints==0)
return new Rectangle();
if (bounds==null)
calculateBounds(xpoints, ypoints, npoints);
return bounds.getBounds();
}
public Rectangle2D.Double getFloatBounds() {
if (npoints==0)
return new Rectangle2D.Double();
if (bounds==null)
calculateBounds(xpoints, ypoints, npoints);
return new Rectangle2D.Double(minX, minY, maxX-minX, maxY-minY);
}
void calculateBounds(float[] xpoints, float[] ypoints, int npoints) {
minX = Float.MAX_VALUE;
minY = Float.MAX_VALUE;
maxX = Float.MIN_VALUE;
maxY = Float.MIN_VALUE;
for (int i=0; i d2sqr;
}
if (determinate > 0 || collinearAndFurther) {
x2=x3; y2=y3; p2=p3; // p2 is not on the convex hull, p3 becomes the new candidate
}
p3 ++; if (p3==npoints) p3 = 0;
} while (p3 != p1); // all points have been checked whether they are the next one on the convex hull
xx[n2] = (float)x1; // save p1 as a point on the convex hull
yy[n2] = (float)y1;
n2++;
if (p2 == p1) break; // happens only if there was only one unique point
p1 = p2;
if (n2 > 1 && xpoints[p1]==xx[0] && ypoints[p1]==yy[0]) break; //all done but pstart was missed because of duplicate points
} while (p1!=pstart);
return new FloatPolygon(xx, yy, n2);
}
public synchronized void translate(double x, double y) {
float fx = (float)x;
float fy = (float)y;
for (int i=0; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy