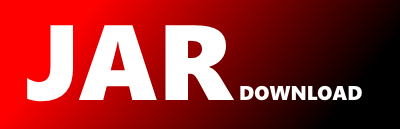
ij.gui.EllipseRoi Maven / Gradle / Ivy
package ij.gui;
import java.awt.*;
import java.awt.image.*;
import java.awt.event.*;
import ij.*;
import ij.plugin.frame.Recorder;
import ij.process.FloatPolygon;
import ij.measure.Calibration;
/** This class implements the ellipse selection tool. */
public class EllipseRoi extends PolygonRoi {
private static final int vertices = 72;
private static double defaultRatio = 0.6;
private double xstart, ystart;
private double aspectRatio = defaultRatio;
private int[] handle = {0, vertices/4, vertices/2, vertices/2+vertices/4};
public EllipseRoi(double x1, double y1, double x2, double y2, double aspectRatio) {
super(new float[vertices], new float[vertices], vertices, FREEROI);
if (aspectRatio<0.0) aspectRatio = 0.0;
if (aspectRatio>1.0) aspectRatio = 1.0;
this.aspectRatio = aspectRatio;
makeEllipse(x1, y1, x2, y2);
state = NORMAL;
bounds = null;
}
public EllipseRoi(int sx, int sy, ImagePlus imp) {
super(sx, sy, imp);
type = FREEROI;
xstart = offScreenXD(sx);
ystart = offScreenYD(sy);
setDrawOffset(false);
bounds = null;
}
public void draw(Graphics g) {
super.draw(g);
if (!overlay) {
for (int i=0; i1.0) aspectRatio = 1.0;
defaultRatio = aspectRatio;
}
public int isHandle(int sx, int sy) {
int size = getHandleSize()+5;
int halfSize = size/2;
int index = -1;
for (int i=0; i=sx2 && sx<=sx2+size && sy>=sy2 && sy<=sy2+size) {
index = i;
break;
}
}
return index;
}
/** Returns the perimeter of this ellipse. */
public double getLength() {
double length = 0.0;
double dx, dy;
double w2=1.0, h2=1.0;
if (imp!=null) {
Calibration cal = imp.getCalibration();
w2 = cal.pixelWidth*cal.pixelWidth;
h2 = cal.pixelHeight*cal.pixelHeight;
}
for (int i=0; i<(nPoints-1); i++) {
dx = xpf[i+1]-xpf[i];
dy = ypf[i+1]-ypf[i];
length += Math.sqrt(dx*dx*w2+dy*dy*h2);
}
dx = xpf[0]-xpf[nPoints-1];
dy = ypf[0]-ypf[nPoints-1];
length += Math.sqrt(dx*dx*w2+dy*dy*h2);
return length;
}
/** Returns x1, y1, x2, y2 and aspectRatio as a 5 element array. */
public double[] getParams() {
double[] params = new double[5];
params[0] = xpf[handle[2]]+x;
params[1] = ypf[handle[2]]+y;
params[2] = xpf[handle[0]]+x;
params[3] = ypf[handle[0]]+y;
params[4] = aspectRatio;
return params;
}
public double[] getFeretValues() {
double a[] = super.getFeretValues();
double pw=1.0, ph=1.0;
if (imp!=null) {
Calibration cal = imp.getCalibration();
pw = cal.pixelWidth;
ph = cal.pixelHeight;
}
if (pw != ph) //the following calculation holds only for pixel aspect ratio == 1 (otherwise different axes in distorted ellipse)
return a;
double[] p = getParams();
double dx = p[2] - p[0]; //this is always major axis; aspect ratio p[4] is limited to <= 1
double dy = p[3] - p[1];
double major = Math.sqrt(dx*dx + dy*dy);
double minor = major*p[4];
a[0] = major*pw; //Feret from convex hull should be accurate anyhow
a[2] = minor*pw; //here our own calculation is better
System.arraycopy(p, 0, a, 8, 4); //MaxFeret endpoints
double xCenter = 0.5*(p[2] + p[0]);
double yCenter = 0.5*(p[3] + p[1]);
double semiMinorX = dy * 0.5 * p[4];
double semiMinorY = dx * (-0.5) * p[4];
a[12] = xCenter + semiMinorX; a[14] = xCenter - semiMinorX;
a[13] = yCenter + semiMinorY; a[15] = yCenter - semiMinorY;
return a;
}
/** Always returns true. */
public boolean subPixelResolution() {
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy