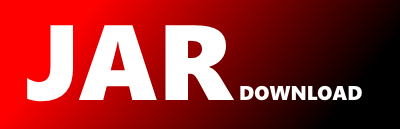
ij.plugin.Grid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ij Show documentation
Show all versions of ij Show documentation
ImageJ is an open source Java image processing program inspired by NIH Image for the Macintosh.
package ij.plugin;
import ij.*;
import ij.process.*;
import ij.gui.*;
import ij.measure.*;
import ij.util.Tools;
import java.awt.*;
import java.awt.geom.*;
import java.util.*;
/** This class implements the Analyze/Tools/Grid command. */
public class Grid implements PlugIn, DialogListener {
private static final String OPTIONS = "grid.options";
private static final String GRID = "|GRID|";
private static double crossSize = 0.1;
private static String[] colors = {"Red","Green","Blue","Magenta","Cyan","Yellow","Orange","Black","White"};
private final static int LINES=0, HLINES=1, CROSSES=2, POINTS=3, CIRCLES=4, NONE=4;
private static String[] types = {"Lines","Horizontal Lines", "Crosses", "Points", "Circles", "None"};
private Random random = new Random(System.currentTimeMillis());
private ImagePlus imp;
private double tileWidth, tileHeight;
private int xstart, ystart;
private int linesV, linesH;
private double pixelWidth=1.0, pixelHeight=1.0;
private String units = "pixels";
private boolean isMacro;
private Roi gridOnEntry;
private String type = types[LINES];
private double areaPerPoint;
private static double saveAreaPerPoint;
private String color = "Cyan";
private boolean bold;
private boolean randomOffset;
private boolean centered;
private Checkbox centerCheckbox, randomCheckbox;
public void run(String arg) {
imp = IJ.getImage();
Overlay overlay = imp.getOverlay();
int index = overlay!=null?overlay.getIndex(GRID):-1;
if (index>=0)
gridOnEntry = overlay.get(index);
if (showDialog() && !isMacro)
saveSettings();
}
// http://stackoverflow.com/questions/30654203/how-to-create-a-circle-using-generalpath-and-apache-poi
private void drawCircles(double size) {
double R = size*tileWidth;
if (R<1) R =1;
if (bold && type.equals(types[POINTS])) R*=1.5;
double kappa = 0.5522847498f;
GeneralPath path = new GeneralPath();
for(int h=0; h1) {
overlay.remove(GRID);
imp.draw();
} else
imp.setOverlay(null);
}
} else {
Roi roi = new ShapeRoi(shape);
roi.setStrokeColor(Colors.getColor(color,Color.cyan));
if (bold && linesV*linesH<5000) {
ImageCanvas ic = imp.getCanvas();
double mag = ic!=null?ic.getMagnification():1.0;
double width = 2.0;
if (mag<1.0)
width = width/mag;
roi.setStrokeWidth(width);
}
IJ.showStatus(linesV*linesH+" nodes");
Overlay overlay = imp.getOverlay();
if (overlay!=null)
overlay.remove(GRID);
else
overlay = new Overlay();
overlay.add(roi, GRID);
imp.setOverlay(overlay);
}
}
private boolean showDialog() {
isMacro = Macro.getOptions()!=null;
if (!isMacro)
getSettings();
int width = imp.getWidth();
int height = imp.getHeight();
Calibration cal = imp.getCalibration();
int places;
if (cal.scaled()) {
pixelWidth = cal.pixelWidth;
pixelHeight = cal.pixelHeight;
units = cal.getUnits();
places = 2;
} else {
pixelWidth = 1.0;
pixelHeight = 1.0;
units = "pixels";
places = 0;
}
if (areaPerPoint==0.0)
areaPerPoint = (width*cal.pixelWidth*height*cal.pixelHeight)/81.0; // default to 9x9 grid
GenericDialog gd = new GenericDialog("Grid...");
gd.addChoice("Grid type:", types, type);
gd.addNumericField("Area per point:", areaPerPoint, places, 6, units+"^2");
gd.addChoice("Color:", colors, color);
gd.addCheckbox("Bold", bold);
gd.addCheckbox("Center grid on image", centered);
gd.addCheckbox("Random offset", randomOffset);
gd.addDialogListener(this);
if (!isMacro) {
Vector v = gd.getCheckboxes();
centerCheckbox = (Checkbox)v.elementAt(1);
randomCheckbox = (Checkbox)v.elementAt(2);
}
dialogItemChanged(gd, null);
gd.showDialog();
if (gd.wasCanceled()) {
Overlay overlay = imp.getOverlay();
if (overlay!=null && gridOnEntry!=null) {
overlay.remove(GRID);
overlay.add(gridOnEntry);
imp.draw();
} else
drawGrid(null);
return false;
} else
return true;
}
public boolean dialogItemChanged(GenericDialog gd, AWTEvent e) {
int width = imp.getWidth();
int height = imp.getHeight();
type = gd.getNextChoice();
areaPerPoint = gd.getNextNumber();
color = gd.getNextChoice();
bold = gd.getNextBoolean();
centered = gd.getNextBoolean();
randomOffset = gd.getNextBoolean();
if (randomOffset) {
centered = false;
if (centerCheckbox!=null)
centerCheckbox.setState(false);
}
double minArea= (width*height)/50000.0;
if (type.equals(types[CROSSES])&&minArea<50.0)
minArea = 50.0;
else if (minArea<16)
minArea = 16.0;
if (areaPerPoint/(pixelWidth*pixelHeight)=3) {
type = options[0];
if ("None".equals(type))
type = types[LINES];
areaPerPoint = saveAreaPerPoint;
color = options[1];
bold = options[2].contains("bold");
centered = options[2].contains("centered");
randomOffset = options[2].contains("random");
if (centered)
randomOffset = false;
}
}
private void saveSettings() {
String options = type+","+color+",";
String options2 = (bold?"bold ":"")+(centered?"centered ":"")+(randomOffset?"random ":"");
if (options2.length()==0)
options2 = "-";
Prefs.set(OPTIONS, options+options2);
saveAreaPerPoint = areaPerPoint;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy