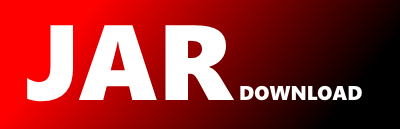
ij.plugin.frame.ColorPicker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ij Show documentation
Show all versions of ij Show documentation
ImageJ is an open source Java image processing program inspired by NIH Image for the Macintosh.
package ij.plugin.frame;
import ij.*;
import ij.plugin.*;
import java.awt.*;
import java.awt.event.*;
import java.util.Vector;
import javax.swing.BoxLayout;
import ij.process.*;
import ij.gui.*;
/** Implements the Image/Color/Color Picker command. */
public class ColorPicker extends PlugInDialog {
public static int ybase = 2;
private int colorWidth = 22;
private int colorHeight = 16;
private int columns = 5;
private int rows = 20;
private static final String LOC_KEY = "cp.loc";
private static ColorPicker instance;
private ColorGenerator cg;
private Canvas colorCanvas;
TextField colorField;
public ColorPicker() {
super("CP");
if (instance!=null) {
instance.toFront();
return;
}
double scale = Prefs.getGuiScale();
instance = this;
WindowManager.addWindow(this);
int width = (int)(columns*colorWidth*scale);
int height = (int)((rows*colorHeight+ybase)*scale);
addKeyListener(IJ.getInstance());
setLayout(new BorderLayout());
cg = new ColorGenerator(width, height, new int[width*height]);
cg.drawColors(colorWidth, colorHeight, columns, rows);
colorCanvas = new ColorCanvas(width, height, this, cg, scale);
Panel panel = new Panel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS));
panel.add(colorCanvas);
String hexColor = Colors.colorToString(Toolbar.getForegroundColor());
colorField = new TextField(hexColor+" ",7);
colorField.setEditable(false);
colorField.select(hexColor.length(),hexColor.length());
GUI.scale(colorField);
panel.add(colorField);
add(panel);
setResizable(false);
pack();
Point loc = Prefs.getLocation(LOC_KEY);
if (loc!=null)
setLocation(loc);
else
GUI.centerOnImageJScreen(this);
show();
}
public void close() {
super.close();
instance = null;
Prefs.saveLocation(LOC_KEY, getLocation());
IJ.notifyEventListeners(IJEventListener.COLOR_PICKER_CLOSED);
}
public static void update() {
ColorPicker cp = instance;
if (cp!=null && cp.colorCanvas!=null) {
cp.cg.refreshBackground(false);
cp.cg.refreshForeground(false);
cp.colorCanvas.repaint();
cp.colorField.setText(Colors.colorToString(Toolbar.getForegroundColor()));
}
}
}
class ColorGenerator extends ColorProcessor {
private int ybase = ColorPicker.ybase;
private int w, h;
private int[] colors = {0xff0000, 0x00ff00, 0x0000ff, 0xffffff, 0x00ffff, 0xff00ff, 0xffff00, 0x000000};
public ColorGenerator(int width, int height, int[] pixels) {
super(width, height, pixels);
setAntialiasedText(true);
}
void drawColors(int colorWidth, int colorHeight, int columns, int rows) {
w = colorWidth;
h = colorHeight;
setColor(0xffffff);
setRoi(0, ybase, 110, 320);
fill();
drawRamp();
resetBW();
flipper();
//drawLine(0, 256, 110, 256);
refreshBackground(false);
refreshForeground(false);
Color c;
float hue, saturation=1f, brightness=1f;
double w=colorWidth, h=colorHeight;
for (int x=2; x<10; x++) {
for (int y=0; y<32; y++) {
hue = (float)(y/(2*h)-.15);
if (x<6) {
saturation = 1f;
brightness = (float)(x*4/w);
} else {
saturation = 1f - ((float)((5-x)*-4/w));
brightness = 1f;
}
c = Color.getHSBColor(hue, saturation, brightness);
setRoi(x*(int)(w/2), ybase+y*(int)(h/2), (int)w/2, (int)h/2);
setColor(c);
fill();
}
}
drawSpectrum(h);
resetRoi();
}
void drawColor(int x, int y, Color c) {
setRoi(x*w, y*h, w, h);
setColor(c);
fill();
}
public void refreshBackground(boolean backgroundInFront) {
//Boundary for Background Selection
setColor(0x444444);
drawRect((w*2)-12, ybase+276, (w*2)+4, (h*2)+4);
setColor(0x999999);
drawRect((w*2)-11, ybase+277, (w*2)+2, (h*2)+2);
setRoi((w*2)-10, ybase+278, w*2, h*2);//Paints the Background Color
Color bg = Toolbar.getBackgroundColor();
setColor(bg);
fill();
if (backgroundInFront)
drawLabel("B", bg, w*4-18, ybase+278+h*2);
}
public void refreshForeground(boolean backgroundInFront) {
//Boundary for Foreground Selection
setColor(0x444444);
drawRect(8, ybase+266, (w*2)+4, (h*2)+4);
setColor(0x999999);
drawRect(9, ybase+267, (w*2)+2, (h*2)+2);
setRoi(10, ybase+268, w*2, h*2); //Paints the Foreground Color
Color fg = Toolbar.getForegroundColor();
setColor(fg);
fill();
if (backgroundInFront)
drawLabel("F", fg, 12, ybase+268+14);
}
private void drawLabel(String label, Color c, int x, int y) {
int intensity = (c.getRed()+c.getGreen()+c.getBlue())/3;
c = intensity<128?Color.white:Color.black;
setColor(c);
drawString(label, x, y);
}
void drawSpectrum(double h) {
Color c;
for ( int x=5; x<7; x++) {
for ( int y=0; y<32; y++) {
float hue = (float)(y/(2*h)-.15);
c = Color.getHSBColor(hue, 1f, 1f);
setRoi(x*(int)(w/2), ybase+y*(int)(h/2), (int)w/2, (int)h/2);
setColor(c);
fill();
}
}
setRoi(55, ybase+32, 22, 16); //Solid red
setColor(0xff0000);
fill();
setRoi(55, ybase+120, 22, 16); //Solid green
setColor(0x00ff00);
fill();
setRoi(55, ybase+208, 22, 16); //Solid blue
setColor(0x0000ff);
fill();
setRoi(55, ybase+80, 22, 8); //Solid yellow
setColor(0xffff00);
fill();
setRoi(55, ybase+168, 22, 8); //Solid cyan
setColor(0x00ffff);
fill();
setRoi(55, ybase+248, 22, 8); //Solid magenta
setColor(0xff00ff);
fill();
}
void drawRamp() {
int r,g,b;
for (int x=0; x>16;
int g = (p&0xff00)>>8;
int b = p&0xff;
Color c = new Color(r, g, b);
if (setBackground) {
Toolbar.setBackgroundColor(c);
if (Recorder.record)
Recorder.setBackgroundColor(c);
} else {
Toolbar.setForegroundColor(c);
if (Recorder.record)
Recorder.setForegroundColor(c);
}
}
void editColor() {
Color c = background?Toolbar.getBackgroundColor():Toolbar.getForegroundColor();
ColorChooser cc = new ColorChooser((background?"Background":"Foreground")+" Color", c, false);
c = cc.getColor();
if (background)
Toolbar.setBackgroundColor(c);
else
Toolbar.setForegroundColor(c);
}
public void refreshColors() {
ip.refreshBackground(false);
ip.refreshForeground(false);
repaint();
}
private void showStatus(String msg, int rgb) {
if (msg.length()>1)
IJ.showStatus(msg);
else {
int r = (rgb&0xff0000)>>16;
int g = (rgb&0xff00)>>8;
int b = rgb&0xff;
String hex = Colors.colorToString(new Color(r,g,b));
IJ.showStatus("red="+pad(r)+", green="+pad(g)+", blue="+pad(b)+" ("+hex+") "+msg);
}
}
public void mouseExited(MouseEvent e) {
IJ.showStatus("");
setCursor(defaultCursor);
}
public void mouseEntered(MouseEvent e) {
setCursor(crosshairCursor);
}
public void mouseReleased(MouseEvent e) {}
public void mouseClicked(MouseEvent e) {}
public void mouseDragged(MouseEvent e) {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy