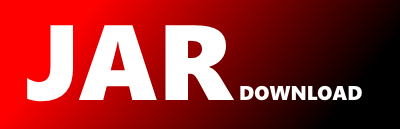
Energistics.Protocol.GrowingObject.GrowingObjectDelete Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package Energistics.Protocol.GrowingObject;
import org.apache.avro.message.BinaryMessageDecoder;
import org.apache.avro.message.BinaryMessageEncoder;
import org.apache.avro.message.SchemaStore;
import org.apache.avro.specific.SpecificData;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class GrowingObjectDelete extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = -6809161825221115075L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"GrowingObjectDelete\",\"namespace\":\"Energistics.Protocol.GrowingObject\",\"fields\":[{\"name\":\"uri\",\"type\":\"string\"},{\"name\":\"uid\",\"type\":\"string\"}],\"messageType\":\"1\",\"protocol\":\"6\",\"senderRole\":\"customer\",\"protocolRoles\":\"store,customer\",\"fullName\":\"Energistics.Protocol.GrowingObject.GrowingObjectDelete\",\"depends\":[]}");
public static org.apache.avro.Schema getClassSchema() {
return SCHEMA$;
}
private static SpecificData MODEL$ = new SpecificData();
private static final BinaryMessageEncoder ENCODER =
new BinaryMessageEncoder(MODEL$, SCHEMA$);
private static final BinaryMessageDecoder DECODER =
new BinaryMessageDecoder(MODEL$, SCHEMA$);
/**
* Return the BinaryMessageDecoder instance used by this class.
*/
public static BinaryMessageDecoder getDecoder() {
return DECODER;
}
/**
* Create a new BinaryMessageDecoder instance for this class that uses the specified {@link SchemaStore}.
*
* @param resolver a {@link SchemaStore} used to find schemas by fingerprint
*/
public static BinaryMessageDecoder createDecoder(SchemaStore resolver) {
return new BinaryMessageDecoder(MODEL$, SCHEMA$, resolver);
}
/**
* Serializes this GrowingObjectDelete to a ByteBuffer.
*/
public java.nio.ByteBuffer toByteBuffer() throws java.io.IOException {
return ENCODER.encode(this);
}
/**
* Deserializes a GrowingObjectDelete from a ByteBuffer.
*/
public static GrowingObjectDelete fromByteBuffer(
java.nio.ByteBuffer b) throws java.io.IOException {
return DECODER.decode(b);
}
private java.lang.CharSequence uri;
private java.lang.CharSequence uid;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public GrowingObjectDelete() {
}
/**
* All-args constructor.
*
* @param uri The new value for uri
* @param uid The new value for uid
*/
public GrowingObjectDelete(java.lang.CharSequence uri, java.lang.CharSequence uid) {
this.uri = uri;
this.uid = uid;
}
public org.apache.avro.Schema getSchema() {
return SCHEMA$;
}
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0:
return uri;
case 1:
return uid;
default:
throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value = "unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0:
uri = (java.lang.CharSequence) value$;
break;
case 1:
uid = (java.lang.CharSequence) value$;
break;
default:
throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'uri' field.
*
* @return The value of the 'uri' field.
*/
public java.lang.CharSequence getUri() {
return uri;
}
/**
* Sets the value of the 'uri' field.
*
* @param value the value to set.
*/
public void setUri(java.lang.CharSequence value) {
this.uri = value;
}
/**
* Gets the value of the 'uid' field.
*
* @return The value of the 'uid' field.
*/
public java.lang.CharSequence getUid() {
return uid;
}
/**
* Sets the value of the 'uid' field.
*
* @param value the value to set.
*/
public void setUid(java.lang.CharSequence value) {
this.uid = value;
}
/**
* Creates a new GrowingObjectDelete RecordBuilder.
*
* @return A new GrowingObjectDelete RecordBuilder
*/
public static Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder newBuilder() {
return new Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder();
}
/**
* Creates a new GrowingObjectDelete RecordBuilder by copying an existing Builder.
*
* @param other The existing builder to copy.
* @return A new GrowingObjectDelete RecordBuilder
*/
public static Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder newBuilder(Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder other) {
return new Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder(other);
}
/**
* Creates a new GrowingObjectDelete RecordBuilder by copying an existing GrowingObjectDelete instance.
*
* @param other The existing instance to copy.
* @return A new GrowingObjectDelete RecordBuilder
*/
public static Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder newBuilder(Energistics.Protocol.GrowingObject.GrowingObjectDelete other) {
return new Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder(other);
}
/**
* RecordBuilder for GrowingObjectDelete instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.CharSequence uri;
private java.lang.CharSequence uid;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder other) {
super(other);
if (isValidValue(fields()[0], other.uri)) {
this.uri = data().deepCopy(fields()[0].schema(), other.uri);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.uid)) {
this.uid = data().deepCopy(fields()[1].schema(), other.uid);
fieldSetFlags()[1] = true;
}
}
/**
* Creates a Builder by copying an existing GrowingObjectDelete instance
* @param other The existing instance to copy.
*/
private Builder(Energistics.Protocol.GrowingObject.GrowingObjectDelete other) {
super(SCHEMA$);
if (isValidValue(fields()[0], other.uri)) {
this.uri = data().deepCopy(fields()[0].schema(), other.uri);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.uid)) {
this.uid = data().deepCopy(fields()[1].schema(), other.uid);
fieldSetFlags()[1] = true;
}
}
/**
* Gets the value of the 'uri' field.
*
* @return The value.
*/
public java.lang.CharSequence getUri() {
return uri;
}
/**
* Sets the value of the 'uri' field.
*
* @param value The value of 'uri'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder setUri(java.lang.CharSequence value) {
validate(fields()[0], value);
this.uri = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'uri' field has been set.
*
* @return True if the 'uri' field has been set, false otherwise.
*/
public boolean hasUri() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'uri' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder clearUri() {
uri = null;
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'uid' field.
*
* @return The value.
*/
public java.lang.CharSequence getUid() {
return uid;
}
/**
* Sets the value of the 'uid' field.
*
* @param value The value of 'uid'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder setUid(java.lang.CharSequence value) {
validate(fields()[1], value);
this.uid = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'uid' field has been set.
*
* @return True if the 'uid' field has been set, false otherwise.
*/
public boolean hasUid() {
return fieldSetFlags()[1];
}
/**
* Clears the value of the 'uid' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectDelete.Builder clearUid() {
uid = null;
fieldSetFlags()[1] = false;
return this;
}
@Override
@SuppressWarnings("unchecked")
public GrowingObjectDelete build() {
try {
GrowingObjectDelete record = new GrowingObjectDelete();
record.uri = fieldSetFlags()[0] ? this.uri : (java.lang.CharSequence) defaultValue(fields()[0]);
record.uid = fieldSetFlags()[1] ? this.uid : (java.lang.CharSequence) defaultValue(fields()[1]);
return record;
} catch (java.lang.Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumWriter
WRITER$ = (org.apache.avro.io.DatumWriter) MODEL$.createDatumWriter(SCHEMA$);
@Override
public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumReader
READER$ = (org.apache.avro.io.DatumReader) MODEL$.createDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy