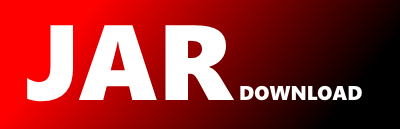
Energistics.Protocol.GrowingObject.GrowingObjectGetRange Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package Energistics.Protocol.GrowingObject;
import org.apache.avro.message.BinaryMessageDecoder;
import org.apache.avro.message.BinaryMessageEncoder;
import org.apache.avro.message.SchemaStore;
import org.apache.avro.specific.SpecificData;
@SuppressWarnings("all")
@org.apache.avro.specific.AvroGenerated
public class GrowingObjectGetRange extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = 8509116472303129354L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"GrowingObjectGetRange\",\"namespace\":\"Energistics.Protocol.GrowingObject\",\"fields\":[{\"name\":\"uri\",\"type\":\"string\"},{\"name\":\"startIndex\",\"type\":{\"type\":\"record\",\"name\":\"GrowingObjectIndex\",\"namespace\":\"Energistics.Datatypes.Object\",\"fields\":[{\"name\":\"item\",\"type\":[\"null\",\"long\",\"double\"]}],\"fullName\":\"Energistics.Datatypes.Object.GrowingObjectIndex\",\"depends\":[]}},{\"name\":\"endIndex\",\"type\":\"Energistics.Datatypes.Object.GrowingObjectIndex\"},{\"name\":\"uom\",\"type\":\"string\"},{\"name\":\"depthDatum\",\"type\":\"string\"}],\"messageType\":\"4\",\"protocol\":\"6\",\"senderRole\":\"customer\",\"protocolRoles\":\"store,customer\",\"fullName\":\"Energistics.Protocol.GrowingObject.GrowingObjectGetRange\",\"depends\":[\"Energistics.Datatypes.Object.GrowingObjectIndex\",\"Energistics.Datatypes.Object.GrowingObjectIndex\"]}");
public static org.apache.avro.Schema getClassSchema() {
return SCHEMA$;
}
private static SpecificData MODEL$ = new SpecificData();
private static final BinaryMessageEncoder ENCODER =
new BinaryMessageEncoder(MODEL$, SCHEMA$);
private static final BinaryMessageDecoder DECODER =
new BinaryMessageDecoder(MODEL$, SCHEMA$);
/**
* Return the BinaryMessageDecoder instance used by this class.
*/
public static BinaryMessageDecoder getDecoder() {
return DECODER;
}
/**
* Create a new BinaryMessageDecoder instance for this class that uses the specified {@link SchemaStore}.
*
* @param resolver a {@link SchemaStore} used to find schemas by fingerprint
*/
public static BinaryMessageDecoder createDecoder(SchemaStore resolver) {
return new BinaryMessageDecoder(MODEL$, SCHEMA$, resolver);
}
/**
* Serializes this GrowingObjectGetRange to a ByteBuffer.
*/
public java.nio.ByteBuffer toByteBuffer() throws java.io.IOException {
return ENCODER.encode(this);
}
/**
* Deserializes a GrowingObjectGetRange from a ByteBuffer.
*/
public static GrowingObjectGetRange fromByteBuffer(
java.nio.ByteBuffer b) throws java.io.IOException {
return DECODER.decode(b);
}
private java.lang.CharSequence uri;
private Energistics.Datatypes.Object.GrowingObjectIndex startIndex;
private Energistics.Datatypes.Object.GrowingObjectIndex endIndex;
private java.lang.CharSequence uom;
private java.lang.CharSequence depthDatum;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public GrowingObjectGetRange() {
}
/**
* All-args constructor.
*
* @param uri The new value for uri
* @param startIndex The new value for startIndex
* @param endIndex The new value for endIndex
* @param uom The new value for uom
* @param depthDatum The new value for depthDatum
*/
public GrowingObjectGetRange(java.lang.CharSequence uri, Energistics.Datatypes.Object.GrowingObjectIndex startIndex, Energistics.Datatypes.Object.GrowingObjectIndex endIndex, java.lang.CharSequence uom, java.lang.CharSequence depthDatum) {
this.uri = uri;
this.startIndex = startIndex;
this.endIndex = endIndex;
this.uom = uom;
this.depthDatum = depthDatum;
}
public org.apache.avro.Schema getSchema() {
return SCHEMA$;
}
// Used by DatumWriter. Applications should not call.
public java.lang.Object get(int field$) {
switch (field$) {
case 0:
return uri;
case 1:
return startIndex;
case 2:
return endIndex;
case 3:
return uom;
case 4:
return depthDatum;
default:
throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
// Used by DatumReader. Applications should not call.
@SuppressWarnings(value = "unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0:
uri = (java.lang.CharSequence) value$;
break;
case 1:
startIndex = (Energistics.Datatypes.Object.GrowingObjectIndex) value$;
break;
case 2:
endIndex = (Energistics.Datatypes.Object.GrowingObjectIndex) value$;
break;
case 3:
uom = (java.lang.CharSequence) value$;
break;
case 4:
depthDatum = (java.lang.CharSequence) value$;
break;
default:
throw new org.apache.avro.AvroRuntimeException("Bad index");
}
}
/**
* Gets the value of the 'uri' field.
*
* @return The value of the 'uri' field.
*/
public java.lang.CharSequence getUri() {
return uri;
}
/**
* Sets the value of the 'uri' field.
*
* @param value the value to set.
*/
public void setUri(java.lang.CharSequence value) {
this.uri = value;
}
/**
* Gets the value of the 'startIndex' field.
*
* @return The value of the 'startIndex' field.
*/
public Energistics.Datatypes.Object.GrowingObjectIndex getStartIndex() {
return startIndex;
}
/**
* Sets the value of the 'startIndex' field.
*
* @param value the value to set.
*/
public void setStartIndex(Energistics.Datatypes.Object.GrowingObjectIndex value) {
this.startIndex = value;
}
/**
* Gets the value of the 'endIndex' field.
*
* @return The value of the 'endIndex' field.
*/
public Energistics.Datatypes.Object.GrowingObjectIndex getEndIndex() {
return endIndex;
}
/**
* Sets the value of the 'endIndex' field.
*
* @param value the value to set.
*/
public void setEndIndex(Energistics.Datatypes.Object.GrowingObjectIndex value) {
this.endIndex = value;
}
/**
* Gets the value of the 'uom' field.
*
* @return The value of the 'uom' field.
*/
public java.lang.CharSequence getUom() {
return uom;
}
/**
* Sets the value of the 'uom' field.
*
* @param value the value to set.
*/
public void setUom(java.lang.CharSequence value) {
this.uom = value;
}
/**
* Gets the value of the 'depthDatum' field.
*
* @return The value of the 'depthDatum' field.
*/
public java.lang.CharSequence getDepthDatum() {
return depthDatum;
}
/**
* Sets the value of the 'depthDatum' field.
*
* @param value the value to set.
*/
public void setDepthDatum(java.lang.CharSequence value) {
this.depthDatum = value;
}
/**
* Creates a new GrowingObjectGetRange RecordBuilder.
*
* @return A new GrowingObjectGetRange RecordBuilder
*/
public static Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder newBuilder() {
return new Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder();
}
/**
* Creates a new GrowingObjectGetRange RecordBuilder by copying an existing Builder.
*
* @param other The existing builder to copy.
* @return A new GrowingObjectGetRange RecordBuilder
*/
public static Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder newBuilder(Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder other) {
return new Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder(other);
}
/**
* Creates a new GrowingObjectGetRange RecordBuilder by copying an existing GrowingObjectGetRange instance.
*
* @param other The existing instance to copy.
* @return A new GrowingObjectGetRange RecordBuilder
*/
public static Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder newBuilder(Energistics.Protocol.GrowingObject.GrowingObjectGetRange other) {
return new Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder(other);
}
/**
* RecordBuilder for GrowingObjectGetRange instances.
*/
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
private java.lang.CharSequence uri;
private Energistics.Datatypes.Object.GrowingObjectIndex startIndex;
private Energistics.Datatypes.Object.GrowingObjectIndex.Builder startIndexBuilder;
private Energistics.Datatypes.Object.GrowingObjectIndex endIndex;
private Energistics.Datatypes.Object.GrowingObjectIndex.Builder endIndexBuilder;
private java.lang.CharSequence uom;
private java.lang.CharSequence depthDatum;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder other) {
super(other);
if (isValidValue(fields()[0], other.uri)) {
this.uri = data().deepCopy(fields()[0].schema(), other.uri);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.startIndex)) {
this.startIndex = data().deepCopy(fields()[1].schema(), other.startIndex);
fieldSetFlags()[1] = true;
}
if (other.hasStartIndexBuilder()) {
this.startIndexBuilder = Energistics.Datatypes.Object.GrowingObjectIndex.newBuilder(other.getStartIndexBuilder());
}
if (isValidValue(fields()[2], other.endIndex)) {
this.endIndex = data().deepCopy(fields()[2].schema(), other.endIndex);
fieldSetFlags()[2] = true;
}
if (other.hasEndIndexBuilder()) {
this.endIndexBuilder = Energistics.Datatypes.Object.GrowingObjectIndex.newBuilder(other.getEndIndexBuilder());
}
if (isValidValue(fields()[3], other.uom)) {
this.uom = data().deepCopy(fields()[3].schema(), other.uom);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.depthDatum)) {
this.depthDatum = data().deepCopy(fields()[4].schema(), other.depthDatum);
fieldSetFlags()[4] = true;
}
}
/**
* Creates a Builder by copying an existing GrowingObjectGetRange instance
*
* @param other The existing instance to copy.
*/
private Builder(Energistics.Protocol.GrowingObject.GrowingObjectGetRange other) {
super(SCHEMA$);
if (isValidValue(fields()[0], other.uri)) {
this.uri = data().deepCopy(fields()[0].schema(), other.uri);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.startIndex)) {
this.startIndex = data().deepCopy(fields()[1].schema(), other.startIndex);
fieldSetFlags()[1] = true;
}
this.startIndexBuilder = null;
if (isValidValue(fields()[2], other.endIndex)) {
this.endIndex = data().deepCopy(fields()[2].schema(), other.endIndex);
fieldSetFlags()[2] = true;
}
this.endIndexBuilder = null;
if (isValidValue(fields()[3], other.uom)) {
this.uom = data().deepCopy(fields()[3].schema(), other.uom);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.depthDatum)) {
this.depthDatum = data().deepCopy(fields()[4].schema(), other.depthDatum);
fieldSetFlags()[4] = true;
}
}
/**
* Gets the value of the 'uri' field.
*
* @return The value.
*/
public java.lang.CharSequence getUri() {
return uri;
}
/**
* Sets the value of the 'uri' field.
*
* @param value The value of 'uri'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setUri(java.lang.CharSequence value) {
validate(fields()[0], value);
this.uri = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'uri' field has been set.
*
* @return True if the 'uri' field has been set, false otherwise.
*/
public boolean hasUri() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'uri' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder clearUri() {
uri = null;
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'startIndex' field.
*
* @return The value.
*/
public Energistics.Datatypes.Object.GrowingObjectIndex getStartIndex() {
return startIndex;
}
/**
* Sets the value of the 'startIndex' field.
*
* @param value The value of 'startIndex'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setStartIndex(Energistics.Datatypes.Object.GrowingObjectIndex value) {
validate(fields()[1], value);
this.startIndexBuilder = null;
this.startIndex = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'startIndex' field has been set.
*
* @return True if the 'startIndex' field has been set, false otherwise.
*/
public boolean hasStartIndex() {
return fieldSetFlags()[1];
}
/**
* Gets the Builder instance for the 'startIndex' field and creates one if it doesn't exist yet.
*
* @return This builder.
*/
public Energistics.Datatypes.Object.GrowingObjectIndex.Builder getStartIndexBuilder() {
if (startIndexBuilder == null) {
if (hasStartIndex()) {
setStartIndexBuilder(Energistics.Datatypes.Object.GrowingObjectIndex.newBuilder(startIndex));
} else {
setStartIndexBuilder(Energistics.Datatypes.Object.GrowingObjectIndex.newBuilder());
}
}
return startIndexBuilder;
}
/**
* Sets the Builder instance for the 'startIndex' field
*
* @param value The builder instance that must be set.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setStartIndexBuilder(Energistics.Datatypes.Object.GrowingObjectIndex.Builder value) {
clearStartIndex();
startIndexBuilder = value;
return this;
}
/**
* Checks whether the 'startIndex' field has an active Builder instance
*
* @return True if the 'startIndex' field has an active Builder instance
*/
public boolean hasStartIndexBuilder() {
return startIndexBuilder != null;
}
/**
* Clears the value of the 'startIndex' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder clearStartIndex() {
startIndex = null;
startIndexBuilder = null;
fieldSetFlags()[1] = false;
return this;
}
/**
* Gets the value of the 'endIndex' field.
*
* @return The value.
*/
public Energistics.Datatypes.Object.GrowingObjectIndex getEndIndex() {
return endIndex;
}
/**
* Sets the value of the 'endIndex' field.
*
* @param value The value of 'endIndex'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setEndIndex(Energistics.Datatypes.Object.GrowingObjectIndex value) {
validate(fields()[2], value);
this.endIndexBuilder = null;
this.endIndex = value;
fieldSetFlags()[2] = true;
return this;
}
/**
* Checks whether the 'endIndex' field has been set.
*
* @return True if the 'endIndex' field has been set, false otherwise.
*/
public boolean hasEndIndex() {
return fieldSetFlags()[2];
}
/**
* Gets the Builder instance for the 'endIndex' field and creates one if it doesn't exist yet.
*
* @return This builder.
*/
public Energistics.Datatypes.Object.GrowingObjectIndex.Builder getEndIndexBuilder() {
if (endIndexBuilder == null) {
if (hasEndIndex()) {
setEndIndexBuilder(Energistics.Datatypes.Object.GrowingObjectIndex.newBuilder(endIndex));
} else {
setEndIndexBuilder(Energistics.Datatypes.Object.GrowingObjectIndex.newBuilder());
}
}
return endIndexBuilder;
}
/**
* Sets the Builder instance for the 'endIndex' field
*
* @param value The builder instance that must be set.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setEndIndexBuilder(Energistics.Datatypes.Object.GrowingObjectIndex.Builder value) {
clearEndIndex();
endIndexBuilder = value;
return this;
}
/**
* Checks whether the 'endIndex' field has an active Builder instance
*
* @return True if the 'endIndex' field has an active Builder instance
*/
public boolean hasEndIndexBuilder() {
return endIndexBuilder != null;
}
/**
* Clears the value of the 'endIndex' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder clearEndIndex() {
endIndex = null;
endIndexBuilder = null;
fieldSetFlags()[2] = false;
return this;
}
/**
* Gets the value of the 'uom' field.
*
* @return The value.
*/
public java.lang.CharSequence getUom() {
return uom;
}
/**
* Sets the value of the 'uom' field.
*
* @param value The value of 'uom'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setUom(java.lang.CharSequence value) {
validate(fields()[3], value);
this.uom = value;
fieldSetFlags()[3] = true;
return this;
}
/**
* Checks whether the 'uom' field has been set.
*
* @return True if the 'uom' field has been set, false otherwise.
*/
public boolean hasUom() {
return fieldSetFlags()[3];
}
/**
* Clears the value of the 'uom' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder clearUom() {
uom = null;
fieldSetFlags()[3] = false;
return this;
}
/**
* Gets the value of the 'depthDatum' field.
*
* @return The value.
*/
public java.lang.CharSequence getDepthDatum() {
return depthDatum;
}
/**
* Sets the value of the 'depthDatum' field.
*
* @param value The value of 'depthDatum'.
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder setDepthDatum(java.lang.CharSequence value) {
validate(fields()[4], value);
this.depthDatum = value;
fieldSetFlags()[4] = true;
return this;
}
/**
* Checks whether the 'depthDatum' field has been set.
*
* @return True if the 'depthDatum' field has been set, false otherwise.
*/
public boolean hasDepthDatum() {
return fieldSetFlags()[4];
}
/**
* Clears the value of the 'depthDatum' field.
*
* @return This builder.
*/
public Energistics.Protocol.GrowingObject.GrowingObjectGetRange.Builder clearDepthDatum() {
depthDatum = null;
fieldSetFlags()[4] = false;
return this;
}
@Override
@SuppressWarnings("unchecked")
public GrowingObjectGetRange build() {
try {
GrowingObjectGetRange record = new GrowingObjectGetRange();
record.uri = fieldSetFlags()[0] ? this.uri : (java.lang.CharSequence) defaultValue(fields()[0]);
if (startIndexBuilder != null) {
record.startIndex = this.startIndexBuilder.build();
} else {
record.startIndex = fieldSetFlags()[1] ? this.startIndex : (Energistics.Datatypes.Object.GrowingObjectIndex) defaultValue(fields()[1]);
}
if (endIndexBuilder != null) {
record.endIndex = this.endIndexBuilder.build();
} else {
record.endIndex = fieldSetFlags()[2] ? this.endIndex : (Energistics.Datatypes.Object.GrowingObjectIndex) defaultValue(fields()[2]);
}
record.uom = fieldSetFlags()[3] ? this.uom : (java.lang.CharSequence) defaultValue(fields()[3]);
record.depthDatum = fieldSetFlags()[4] ? this.depthDatum : (java.lang.CharSequence) defaultValue(fields()[4]);
return record;
} catch (java.lang.Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumWriter
WRITER$ = (org.apache.avro.io.DatumWriter) MODEL$.createDatumWriter(SCHEMA$);
@Override
public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumReader
READER$ = (org.apache.avro.io.DatumReader)MODEL$.createDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy