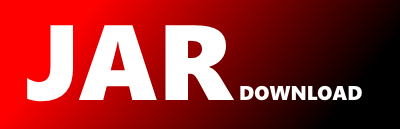
net.intelie.liverig.witsml.ObjectInfoExtractor Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml;
import net.intelie.liverig.util.XMLInputFactoryFactory;
import net.intelie.liverig.util.XMLStreamReaderAutoCloseable;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.xml.XMLConstants;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamReader;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.List;
import static javax.xml.stream.XMLStreamConstants.END_ELEMENT;
import static javax.xml.stream.XMLStreamConstants.START_ELEMENT;
public class ObjectInfoExtractor {
public static ObjectInfo tryExtract(String data) throws XMLStreamException {
try (XMLStreamReaderAutoCloseable reader = XMLInputFactoryFactory.createXMLStreamReader(new StringReader(data))) {
return tryExtract(reader.get());
}
}
private static ObjectInfo tryExtract(XMLStreamReader reader) throws XMLStreamException {
String collection = null;
String version = null;
List uids = new ArrayList<>();
int level = 0;
while (reader.hasNext()) {
reader.next();
switch (reader.getEventType()) {
case START_ELEMENT:
level++;
if (level == 1) {
collection = reader.getLocalName();
version = reader.getAttributeValue(XMLConstants.NULL_NS_URI, "version");
} else if (level == 2 && (reader.getLocalName() + "s").equals(collection)) {
String uid = reader.getAttributeValue(XMLConstants.NULL_NS_URI, "uid");
if (uid != null && !uid.isEmpty())
uids.add(uid);
}
break;
case END_ELEMENT:
level--;
break;
default:
break;
}
}
return new ObjectInfo(collection, version, uids);
}
public static class ObjectInfo {
private final String collection;
private final String version;
private final List uids;
ObjectInfo(@Nullable String collection, @Nullable String version, @NotNull List uids) {
this.collection = collection;
this.version = version;
this.uids = uids;
}
@Nullable
public String getCollection() {
return collection;
}
@Nullable
public String getVersion() {
return version;
}
@NotNull
public List getUids() {
return uids;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy