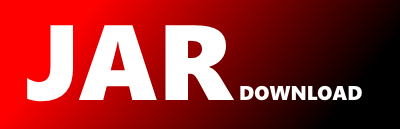
net.intelie.liverig.witsml.StoreSoapPortClient Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml;
import org.witsml.wsdl._120.StoreSoapPort;
import javax.xml.ws.Holder;
import javax.xml.ws.WebServiceException;
/** Wrapper around StoreSoapPort with an easier to use API. */
public class StoreSoapPortClient {
/** The real StoreSoapPort. */
private final StoreSoapPort port;
/** The capClient XML element to be sent as the CapabilitiesIn parameter. */
private final String capClient;
public StoreSoapPortClient(StoreSoapPort port, String capClient) {
this.port = port;
this.capClient = capClient;
}
public StoreSoapPort getPort() {
return port;
}
public String addToStore(String type, String xml, String options) throws WebServiceException, WITSMLException {
Holder suppMsgOut = new Holder<>();
check(port.wmlsAddToStore(type, xml, options, capClient, suppMsgOut), suppMsgOut);
return suppMsgOut.value;
}
public void deleteFromStore(String type, String query, String options) throws WebServiceException, WITSMLException {
Holder suppMsgOut = new Holder<>();
check(port.wmlsDeleteFromStore(type, query, options, capClient, suppMsgOut), suppMsgOut);
}
public String getBaseMsg(short result) throws WebServiceException {
return port.wmlsGetBaseMsg(result);
}
public String getCap(String options) throws WebServiceException, WITSMLException {
Holder capabilitiesOut = new Holder<>();
Holder suppMsgOut = new Holder<>();
check(port.wmlsGetCap(options, capabilitiesOut, suppMsgOut), capabilitiesOut, suppMsgOut);
return capabilitiesOut.value;
}
public WITSMLResult getFromStore(String type, String query, String options) throws WebServiceException, WITSMLException {
Holder xmlOut = new Holder<>();
Holder suppMsgOut = new Holder<>();
short result = check(port.wmlsGetFromStore(type, query, options, capClient, xmlOut, suppMsgOut), xmlOut, suppMsgOut);
return new WITSMLResult(result, xmlOut.value);
}
public String getVersion() throws WebServiceException {
return port.wmlsGetVersion();
}
public void updateInStore(String type, String xml, String options) throws WebServiceException, WITSMLException {
Holder suppMsgOut = new Holder<>();
check(port.wmlsUpdateInStore(type, xml, options, capClient, suppMsgOut), suppMsgOut);
}
/** Convert an error return into an exception. */
private static short check(Short result, Holder suppMsgOut) throws WITSMLException {
// NOV is broken and uses WMLS_GetFromStoreResult and similar instead of Result, which leads to null here
if (result != null && result < 0)
throw new WITSMLRawException(result, suppMsgOut.value);
return result != null ? result : 0;
}
/** Convert an error return into an exception. */
private static short check(Short result, Holder holder, Holder suppMsgOut) throws WITSMLException {
// NOV is broken and uses WMLS_GetFromStoreResult and similar instead of Result, which leads to null here
if (result != null && result < 0)
throw new WITSMLRawException(result, suppMsgOut.value, holder.value);
return result != null ? result : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy