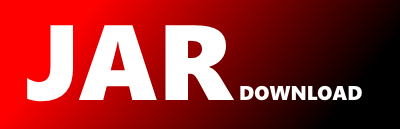
net.intelie.liverig.witsml.WITSMLClientFacade Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.base.Strings;
import net.intelie.liverig.parser.ParseException;
import net.intelie.liverig.witsml.objects.*;
import net.intelie.liverig.witsml.query.*;
import org.jetbrains.annotations.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.witsml.wsdl._120.StoreSoapPort;
import javax.xml.ws.WebServiceException;
import java.util.List;
import java.util.Map;
public class WITSMLClientFacade {
private static final Logger LOGGER = LoggerFactory.getLogger(WITSMLClientFacade.class);
public static final int DEFAULT_TIMEOUT = 15_000;
private final StoreSoapPortClient client;
private final QueryFactory queryFactory;
@Nullable
private String overwriteQueryOptions;
public WITSMLClientFacade(StoreSoapPort port, CapClient capClient) {
this.client = new StoreSoapPortClient(port, capClient.toString());
this.queryFactory = queryFactoryFor(capClient.getApiVers());
}
public WITSMLClientFacade(String address, String username, String password, CapClient capClient) {
this(new StoreSoapPortBuilder()
.endpointAddress(address)
.authentication(username, password)
.connectTimeout(DEFAULT_TIMEOUT)
.requestTimeout(DEFAULT_TIMEOUT)
.getPort(), capClient);
}
public StoreSoapPortClient getClient() {
return client;
}
public StoreSoapPort getPort() {
return client.getPort();
}
public QueryFactory getQueryFactory() {
return queryFactory;
}
public void setOverwriteQueryOptions(@Nullable String overwriteQueryOptions) {
this.overwriteQueryOptions = overwriteQueryOptions;
}
public List getCap() throws WITSMLException, ParseException {
CapabilityQuery query = queryFactory.getCapability();
return query.parse(getCapFromStore(query));
}
public List listWells(WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
WellListQuery query = queryFactory.listWells(filters);
return query.parse(getFromStore(query));
}
public List listWellbores(String uidWell)
throws WebServiceException, WITSMLException, ParseException {
WellboreListQuery query = queryFactory.listWellbores(uidWell);
return query.parse(getFromStore(query));
}
public List listLogs(String uidWell, String uidWellbore, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
return listLogs(uidWell, uidWellbore, filters, HeaderElements.ID_ONLY);
}
public List listLogs(String uidWell, String uidWellbore, WitsmlFilters filters, HeaderElements header)
throws WebServiceException, WITSMLException, ParseException {
LogListQuery query = queryFactory.listLogs(uidWell, uidWellbore, filters, header);
return query.parse(getFromStore(query));
}
public List listMudLogs(String uidWell, String uidWellbore, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
return listMudLogs(uidWell, uidWellbore, filters, HeaderElements.ID_ONLY);
}
public List listMudLogs(String uidWell, String uidWellbore, WitsmlFilters filters, HeaderElements header)
throws WebServiceException, WITSMLException, ParseException {
MudLogListQuery query = queryFactory.listMudLogs(uidWell, uidWellbore, filters, header);
return query.parse(getFromStore(query));
}
public List listTrajectories(String uidWell, String uidWellbore, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
return listTrajectories(uidWell, uidWellbore, filters, HeaderElements.ID_ONLY);
}
public List listTrajectories(String uidWell, String uidWellbore, WitsmlFilters filters, HeaderElements header)
throws WebServiceException, WITSMLException, ParseException {
TrajectoryListQuery query = queryFactory.listTrajectories(uidWell, uidWellbore, filters, header);
return query.parse(getFromStore(query));
}
public List listWellboreGeometries(String uidWell, String uidWellbore)
throws WebServiceException, WITSMLException, ParseException {
return listWellboreGeometries(uidWell, uidWellbore, HeaderElements.ID_ONLY);
}
public List listWellboreGeometries(String uidWell, String uidWellbore, HeaderElements header)
throws WebServiceException, WITSMLException, ParseException {
WellboreGeometryListQuery query = queryFactory.listWellboreGeometries(uidWell, uidWellbore, header);
return query.parse(getFromStore(query));
}
public List listTubulars(String uidWell, String uidWellbore, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
return listTubulars(uidWell, uidWellbore, filters, HeaderElements.ID_ONLY);
}
public List listTubulars(String uidWell, String uidWellbore, WitsmlFilters filters, HeaderElements header)
throws WebServiceException, WITSMLException, ParseException {
TubularListQuery query = queryFactory.listTubulars(uidWell, uidWellbore, filters, header);
return query.parse(getFromStore(query));
}
public List listMessages(String uidWell, String uidWellbore)
throws WebServiceException, WITSMLException, ParseException {
MessageListQuery query = queryFactory.listMessages(uidWell, uidWellbore);
return query.parse(getFromStore(query));
}
public AbstractLogHeader getLogHeader(String uidWell, String uidWellbore, String uid, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
return getLogHeader(uidWell, uidWellbore, uid, filters, false).getHeader();
}
public LogData getLogHeader(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
LogQuery query = queryFactory.getLogHeader(uidWell, uidWellbore, uid, filters, raw);
return query.parse(getFromStore(query));
}
public LogData getLogData(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogRange range)
throws WebServiceException, WITSMLException, ParseException {
return getLogData(uidWell, uidWellbore, uid, filters, range, false);
}
public LogData getLogData(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogRange range, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
LogQuery query = queryFactory.getLogData(uidWell, uidWellbore, uid, filters, range, raw);
return query.parse(getFromStore(query));
}
public RawData getLogDataOrRaw(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogRange range) {
LogQuery query = queryFactory.getLogData(uidWell, uidWellbore, uid, filters, range, true);
return getFromStoreOrRaw(query);
}
public MudLogHeader getMudLogHeader(String uidWell, String uidWellbore, String uid, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
return getMudLogHeader(uidWell, uidWellbore, uid, filters, false).getHeader();
}
public MudLogData getMudLogHeader(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
MudLogQuery query = queryFactory.getMudLogHeader(uidWell, uidWellbore, uid, filters, raw);
return query.parse(getFromStore(query));
}
public MudLogData getMudLogData(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex index)
throws WebServiceException, WITSMLException, ParseException {
return getMudLogData(uidWell, uidWellbore, uid, filters, index, false);
}
public MudLogData getMudLogData(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex index, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
MudLogQuery query = queryFactory.getMudLogData(uidWell, uidWellbore, uid, filters, index, raw);
return query.parse(getFromStore(query));
}
public RawData getMudLogDataOrRaw(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex index) {
MudLogQuery query = queryFactory.getMudLogData(uidWell, uidWellbore, uid, filters, index, true);
return getFromStoreOrRaw(query);
}
public TrajectoryData getTrajectoryHeader(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
TrajectoryQuery query = queryFactory.getTrajectoryHeader(uidWell, uidWellbore, uid, filters, raw);
return query.parse(getFromStore(query));
}
public TrajectoryData getTrajectoryData(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex index, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
TrajectoryQuery query = queryFactory.getTrajectoryData(uidWell, uidWellbore, uid, filters, index, raw);
return query.parse(getFromStore(query));
}
public RawData getTrajectoryDataOrRaw(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex index) {
TrajectoryQuery query = queryFactory.getTrajectoryData(uidWell, uidWellbore, uid, filters, index, true);
return getFromStoreOrRaw(query);
}
public WellboreGeometryData getWellboreGeometryHeader(String uidWell, String uidWellbore, String uid, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
WellboreGeometryQuery query = queryFactory.getWellboreGeometryHeader(uidWell, uidWellbore, uid, raw);
return query.parse(getFromStore(query));
}
public WellboreGeometryData getWellboreGeometryData(String uidWell, String uidWellbore, String uid, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
WellboreGeometryQuery query = queryFactory.getWellboreGeometryData(uidWell, uidWellbore, uid, raw);
return query.parse(getFromStore(query));
}
public RawData getWellboreGeometryDataOrRaw(String uidWell, String uidWellbore, String uid) {
WellboreGeometryQuery query = queryFactory.getWellboreGeometryData(uidWell, uidWellbore, uid, true);
return getFromStoreOrRaw(query);
}
public RawData getTubularDataOrRaw(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, boolean raw) {
TubularQuery query = queryFactory.getTubularData(uidWell, uidWellbore, uid, filters, raw);
return getFromStoreOrRaw(query);
}
public TubularHeader getTubularHeader(String uidWell, String uidWellbore, String uid, WitsmlFilters filters)
throws WebServiceException, WITSMLException, ParseException {
TubularQuery query = queryFactory.getTubularHeader(uidWell, uidWellbore, uid, filters, false);
return query.parse(getFromStore(query)).getHeader();
}
public TubularData getTubularData(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
TubularQuery query = queryFactory.getTubularData(uidWell, uidWellbore, uid, filters, raw);
return query.parse(getFromStore(query));
}
public MessageData getMessage(String uidWell, String uidWellbore, String uid)
throws WebServiceException, WITSMLException, ParseException {
return getMessage(uidWell, uidWellbore, uid, false);
}
public MessageData getMessage(String uidWell, String uidWellbore, String uid, boolean raw)
throws WebServiceException, WITSMLException, ParseException {
MessageQuery query = queryFactory.getMessage(uidWell, uidWellbore, uid, raw);
return query.parse(getFromStore(query));
}
public RawData getMessageOrRaw(String uidWell, String uidWellbore, String uid) {
MessageQuery query = queryFactory.getMessage(uidWell, uidWellbore, uid, true);
return getFromStoreOrRaw(query);
}
@VisibleForTesting
public RawData getFromStoreOrRaw(RawDataQuery query) {
WITSMLResult result;
try {
result = getFromStore(query);
} catch (WITSMLRawException e) {
return new RawData(e.getReturnValue(), e.getRaw(), e.getSuppMsg());
} catch (WITSMLException e) {
return new RawData(e.getReturnValue(), "", e.getMessage());
}
if (Strings.isNullOrEmpty(result.getXml()))
return result.toRawData();
try {
return query.parse(result);
} catch (ParseException e) {
LOGGER.info("Could not parse response", e);
return result.toRawData();
}
}
public void appendLogData(LogHeader header, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy