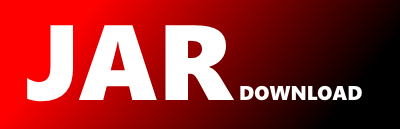
net.intelie.liverig.witsml.etp.processor.ETPMessageProcessor Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml.etp.processor;
import org.apache.avro.io.BinaryDecoder;
import org.apache.avro.io.DecoderFactory;
import org.apache.avro.specific.SpecificDatumReader;
import org.apache.avro.specific.SpecificRecord;
import org.jetbrains.annotations.NotNull;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.concurrent.CountDownLatch;
public abstract class ETPMessageProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(ETPMessageProcessor.class);
private Class getHeaderClass() {
Type type = getClass().getGenericSuperclass();
ParameterizedType paramType = (ParameterizedType) type;
return (Class) paramType.getActualTypeArguments()[0];
}
private Class getBodyClass() {
Type type = getClass().getGenericSuperclass();
ParameterizedType paramType = (ParameterizedType) type;
return (Class) paramType.getActualTypeArguments()[1];
}
public long getDefaultTimeout() {
return 10;
}
abstract BODY parse(@NotNull CountDownLatch messageLatch, @NotNull HEADER header, @NotNull BODY body);
public BODY process(@NotNull CountDownLatch messageLatch, @NotNull byte[] bytes) throws Exception {
HEADER header = getHeaderClass().newInstance();
BODY body = getBodyClass().newInstance();
try {
BinaryDecoder binaryDecoder = DecoderFactory.get().binaryDecoder(bytes, null);
// serialize header
SpecificDatumReader headerWriter = new SpecificDatumReader(header.getSchema());
headerWriter.read(header, binaryDecoder);
body = tryRead(body, binaryDecoder);
LOGGER.debug("Received message: \n{}\n{} ", header, body);
return parse(messageLatch, header, body);
} catch (Exception e) {
LOGGER.error("{} FAIL:\n{}\n{}", this.getClass().getSimpleName(), header, body);
}
return null;
}
protected BODY tryRead(@NotNull BODY body, @NotNull BinaryDecoder binaryDecoder) throws Exception {
// serialize body
SpecificDatumReader bodyWriter = new SpecificDatumReader(body.getSchema());
bodyWriter.read(body, binaryDecoder);
return body;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy