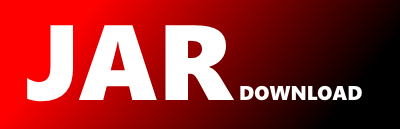
net.intelie.liverig.witsml.etp.processor.GetObjectMessageProcessor Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml.etp.processor;
import Energistics.Datatypes.MessageHeader;
import Energistics.Protocol.Store.Object;
import org.apache.commons.compress.utils.IOUtils;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.ByteArrayInputStream;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.util.concurrent.CountDownLatch;
import java.util.zip.GZIPInputStream;
public class GetObjectMessageProcessor extends ETPMessageProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(GetObjectMessageProcessor.class);
@Override
public Object parse(@NotNull CountDownLatch messageLatch, @NotNull MessageHeader header, @Nullable Object body) {
if (body != null && body.getDataObject() != null) {
LOGGER.debug("Returning body: {} {} {}", body.getDataObject().getResource().getUri(), body.getDataObject().getContentEncoding(), body.getDataObject().getResource().getContentType());
if (body.getDataObject().getContentEncoding().toString().equals("gzip")) {
String unzipedXml = decompress(body.getDataObject().getData().array());
body.getDataObject().setData(ByteBuffer.wrap(unzipedXml.getBytes(StandardCharsets.UTF_8)));
body.getDataObject().setContentEncoding(body.getDataObject().getResource().getContentType());
}
}
messageLatch.countDown();
return body;
}
private String decompress(final byte[] compressed) {
try {
ByteArrayInputStream bis = new ByteArrayInputStream(compressed);
GZIPInputStream gis = new GZIPInputStream(bis);
byte[] bytes = IOUtils.toByteArray(gis);
return new String(bytes, StandardCharsets.UTF_8);
} catch (Exception e) {
LOGGER.error("Fail to decompress", e);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy