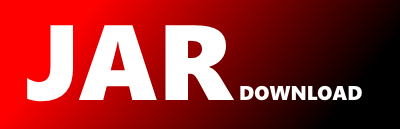
net.intelie.liverig.witsml.query.AbstractMudLogQuery131 Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml.query;
import com.google.common.base.Preconditions;
import com.google.common.base.Strings;
import net.intelie.liverig.witsml.WitsmlFilters;
import net.intelie.liverig.witsml.objects.LogDepthIndex;
import net.intelie.liverig.witsml.objects.LogIndex;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
import static net.intelie.liverig.witsml.query.QueryUtil.addFilter;
abstract class AbstractMudLogQuery131 extends AbstractQuery131 {
private final String uidWell;
private final String uidWellbore;
private final String uid;
private final WitsmlFilters filters;
private final LogIndex range;
private final boolean header;
private final boolean geologyInterval;
AbstractMudLogQuery131(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex range, boolean header, boolean geologyInterval) {
Preconditions.checkArgument(header || range == null);
this.uidWell = uidWell;
this.uidWellbore = uidWellbore;
this.uid = uid;
this.filters = filters;
this.range = range;
this.header = header;
this.geologyInterval = geologyInterval;
}
boolean hasGeologyInterval() {
return geologyInterval;
}
@Override
public String type() {
return "mudLog";
}
@Override
void query(XMLStreamWriter writer) throws XMLStreamException {
writeRootElement(writer, "mudLogs");
writer.writeStartElement(WITSML_NS, "mudLog");
writer.writeAttribute("uidWell", uidWell);
writer.writeAttribute("uidWellbore", uidWellbore);
writer.writeAttribute("uid", Strings.nullToEmpty(uid));
writer.writeEmptyElement(WITSML_NS, "nameWell");
writer.writeEmptyElement(WITSML_NS, "nameWellbore");
writer.writeEmptyElement(WITSML_NS, "name");
addFilter(writer, WITSML_NS, "objectGrowing", filters.objectGrowing());
if (header) {
if (Strings.isNullOrEmpty(filters.objectGrowing()))
writer.writeEmptyElement(WITSML_NS, "objectGrowing");
writer.writeEmptyElement(WITSML_NS, "dTim");
writer.writeEmptyElement(WITSML_NS, "mudLogCompany");
writer.writeEmptyElement(WITSML_NS, "mudLogEngineers");
LogIndex index = range != null ? range : new LogIndex();
LogDepthIndex depthIndex = range instanceof LogDepthIndex ? (LogDepthIndex) range : new LogDepthIndex();
writer.writeStartElement(WITSML_NS, "startMd");
writer.writeAttribute("uom", Strings.nullToEmpty(index.getStartIndexUom()));
writer.writeAttribute("datum", Strings.nullToEmpty(depthIndex.getStartIndexDatum()));
writer.writeCharacters(index.getStartIndex() != null ? index.getStartIndex().toString() : "");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "endMd");
writer.writeAttribute("uom", Strings.nullToEmpty(index.getEndIndexUom()));
writer.writeAttribute("datum", Strings.nullToEmpty(depthIndex.getEndIndexDatum()));
writer.writeCharacters(index.getEndIndex() != null ? index.getEndIndex().toString() : "");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "relatedLog");
}
if (geologyInterval) {
writer.writeStartElement(WITSML_NS, "parameter");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "type");
writer.writeEmptyElement(WITSML_NS, "dTime");
writer.writeEmptyElement(WITSML_NS, "mdTop");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "mdBottom");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "text");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "geologyInterval");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "typeLithology");
writer.writeEmptyElement(WITSML_NS, "mdTop");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "mdBottom");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "dTim");
writer.writeEmptyElement(WITSML_NS, "tvdTop");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tvdBase");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ropAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ropMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ropMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "wobAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tqAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "currentAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "rpmAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "wtMudAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ecdTdAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "dxcAv");
writer.writeStartElement(WITSML_NS, "lithology");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "type");
writer.writeEmptyElement(WITSML_NS, "codeLith");
writer.writeEmptyElement(WITSML_NS, "lithPc");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "description");
writer.writeEmptyElement(WITSML_NS, "lithClass");
writer.writeEmptyElement(WITSML_NS, "grainType");
writer.writeEmptyElement(WITSML_NS, "dunhamClass");
writer.writeEmptyElement(WITSML_NS, "color");
writer.writeEmptyElement(WITSML_NS, "texture");
writer.writeEmptyElement(WITSML_NS, "hardness");
writer.writeEmptyElement(WITSML_NS, "sizeGrain");
writer.writeEmptyElement(WITSML_NS, "roundness");
writer.writeEmptyElement(WITSML_NS, "sorting");
writer.writeEmptyElement(WITSML_NS, "matrixCement");
writer.writeEmptyElement(WITSML_NS, "porosityVisible");
writer.writeEmptyElement(WITSML_NS, "permeability");
writer.writeEmptyElement(WITSML_NS, "densShale");
writer.writeAttribute("uom", "");
writer.writeStartElement(WITSML_NS, "qualifier");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "type");
writer.writeEmptyElement(WITSML_NS, "mdTop");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "mdBottom");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "abundance");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "abundanceCode");
writer.writeEmptyElement(WITSML_NS, "description");
writer.writeEndElement();
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "show");
writer.writeEmptyElement(WITSML_NS, "showRat");
writer.writeEmptyElement(WITSML_NS, "stainColor");
writer.writeEmptyElement(WITSML_NS, "stainDistr");
writer.writeEmptyElement(WITSML_NS, "stainPc");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "natFlorColor");
writer.writeEmptyElement(WITSML_NS, "natFlorPc");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "natFlorLevel");
writer.writeEmptyElement(WITSML_NS, "natFlorDesc");
writer.writeEmptyElement(WITSML_NS, "cutColor");
writer.writeEmptyElement(WITSML_NS, "cutSpeed");
writer.writeEmptyElement(WITSML_NS, "cutStrength");
writer.writeEmptyElement(WITSML_NS, "cutForm");
writer.writeEmptyElement(WITSML_NS, "cutLevel");
writer.writeEmptyElement(WITSML_NS, "cutFlorColor");
writer.writeEmptyElement(WITSML_NS, "cutFlorSpeed");
writer.writeEmptyElement(WITSML_NS, "cutFlorStrength");
writer.writeEmptyElement(WITSML_NS, "cutFlorForm");
writer.writeEmptyElement(WITSML_NS, "cutFlorLevel");
writer.writeEmptyElement(WITSML_NS, "residueColor");
writer.writeEmptyElement(WITSML_NS, "showDesc");
writer.writeEmptyElement(WITSML_NS, "impregnatedLitho");
writer.writeEmptyElement(WITSML_NS, "odor");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "chromatograph");
writer.writeEmptyElement(WITSML_NS, "dTim");
writer.writeEmptyElement(WITSML_NS, "mdTop");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "mdBottom");
writer.writeAttribute("uom", "");
writer.writeAttribute("datum", "");
writer.writeEmptyElement(WITSML_NS, "wtMudIn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "wtMudOut");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "chromType");
writer.writeEmptyElement(WITSML_NS, "eTimChromCycle");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "chromIntRpt");
writer.writeEmptyElement(WITSML_NS, "methAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "methMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "methMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ethAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ethMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ethMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "propAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "propMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "propMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ibutAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ibutMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ibutMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nbutAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nbutMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nbutMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ipentAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ipentMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ipentMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "npentAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "npentMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "npentMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "epentAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "epentMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "epentMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ihexAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ihexMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "ihexMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nhexAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nhexMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nhexMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "co2Av");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "co2Mn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "co2Mx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "h2sAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "h2sMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "h2sMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "acetylene");
writer.writeAttribute("uom", "");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "mudGas");
writer.writeEmptyElement(WITSML_NS, "gasAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "gasPeak");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "gasPeakType");
writer.writeEmptyElement(WITSML_NS, "gasBackgnd");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "gasConAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "gasConMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "gasTrip");
writer.writeAttribute("uom", "");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "densBulk");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "densShale");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "calcite");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "dolomite");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "cec");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "qft");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "calcStab");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "nameFormation");
writer.writeEmptyElement(WITSML_NS, "lithostratigraphic");
writer.writeEmptyElement(WITSML_NS, "chronostratigraphic");
writer.writeEmptyElement(WITSML_NS, "sizeMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "sizeMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "lenPlug");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "description");
writer.writeEmptyElement(WITSML_NS, "cuttingFluid");
writer.writeEmptyElement(WITSML_NS, "cleaningMethod");
writer.writeEmptyElement(WITSML_NS, "dryingMethod");
writer.writeEmptyElement(WITSML_NS, "comments");
writer.writeEndElement();
}
if (header) {
writeCommonData(writer);
writer.writeEmptyElement(WITSML_NS, "customData");
}
writer.writeEndElement();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy