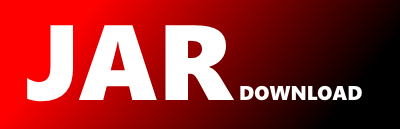
net.intelie.liverig.witsml.query.AbstractTrajectoryQuery131 Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml.query;
import com.google.common.base.Preconditions;
import com.google.common.base.Strings;
import net.intelie.liverig.witsml.WitsmlFilters;
import net.intelie.liverig.witsml.objects.LogDepthIndex;
import net.intelie.liverig.witsml.objects.LogIndex;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
import static net.intelie.liverig.witsml.query.QueryUtil.addFilter;
abstract class AbstractTrajectoryQuery131 extends AbstractQuery131 {
private final String uidWell;
private final String uidWellbore;
private final String uid;
private final WitsmlFilters filters;
private final LogIndex range;
private final boolean header;
private final boolean trajectoryStation;
AbstractTrajectoryQuery131(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, LogIndex range, boolean header, boolean trajectoryStation) {
Preconditions.checkArgument(header || range == null);
this.uidWell = uidWell;
this.uidWellbore = uidWellbore;
this.uid = uid;
this.filters = filters;
this.range = range;
this.header = header;
this.trajectoryStation = trajectoryStation;
}
boolean hasTrajectoryStation() {
return trajectoryStation;
}
@Override
public String type() {
return "trajectory";
}
@Override
void query(XMLStreamWriter writer) throws XMLStreamException {
writeRootElement(writer, "trajectorys");
writer.writeStartElement(WITSML_NS, "trajectory");
writer.writeAttribute("uidWell", uidWell);
writer.writeAttribute("uidWellbore", uidWellbore);
writer.writeAttribute("uid", Strings.nullToEmpty(uid));
writer.writeEmptyElement(WITSML_NS, "nameWell");
writer.writeEmptyElement(WITSML_NS, "nameWellbore");
writer.writeEmptyElement(WITSML_NS, "name");
addFilter(writer, WITSML_NS, "objectGrowing", filters.objectGrowing());
if (header) {
if (Strings.isNullOrEmpty(filters.objectGrowing()))
writer.writeEmptyElement(WITSML_NS, "objectGrowing");
writer.writeStartElement(WITSML_NS,"parentTrajectory");
writer.writeEmptyElement(WITSML_NS,"trajectoryReference");
writer.writeAttribute("uidRef", "");
writer.writeEmptyElement(WITSML_NS,"wellboreParent");
writer.writeAttribute("uidRef", "");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "dTimTrajStart");
writer.writeEmptyElement(WITSML_NS, "dTimTrajEnd");
LogIndex index = range != null ? range : new LogIndex();
LogDepthIndex depthIndex = range instanceof LogDepthIndex ? (LogDepthIndex) range : new LogDepthIndex();
writer.writeStartElement(WITSML_NS,"mdMn");
writer.writeAttribute("uom", Strings.nullToEmpty(index.getStartIndexUom()));
writer.writeAttribute("datum", Strings.nullToEmpty(depthIndex.getStartIndexDatum()));
writer.writeCharacters(index.getStartIndex() != null ? index.getStartIndex().toString() : "");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS,"mdMx");
writer.writeAttribute("uom", Strings.nullToEmpty(index.getEndIndexUom()));
writer.writeAttribute("datum", Strings.nullToEmpty(depthIndex.getEndIndexDatum()));
writer.writeCharacters(index.getEndIndex() != null ? index.getEndIndex().toString() : "");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS,"serviceCompany");
writer.writeEmptyElement(WITSML_NS,"magDeclUsed");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gridCorUsed");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"aziVertSect");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dispNsVertSectOrig");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dispEwVertSectOrig");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"definitive");
writer.writeEmptyElement(WITSML_NS,"memory");
writer.writeEmptyElement(WITSML_NS,"finalTraj");
writer.writeEmptyElement(WITSML_NS,"aziRef");
}
if (trajectoryStation) {
writer.writeStartElement(WITSML_NS,"trajectoryStation");
writer.writeAttribute("uid","");
writer.writeEmptyElement(WITSML_NS, "target");
writer.writeAttribute("uidRef", "");
writer.writeEmptyElement(WITSML_NS, "dTimStn");
writer.writeEmptyElement(WITSML_NS, "typeTrajStation");
writer.writeEmptyElement(WITSML_NS, "typeSurveyTool");
writer.writeEmptyElement(WITSML_NS,"md");
writer.writeAttribute("uom","");
writer.writeAttribute("datum","");
writer.writeEmptyElement(WITSML_NS,"tvd");
writer.writeAttribute("uom","");
writer.writeAttribute("datum","");
writer.writeEmptyElement(WITSML_NS,"incl");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"azi");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"mtf");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gtf");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dispNs");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dispEw");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"vertSect");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dls");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"rateTurn");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"rateBuild");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"mdDelta");
writer.writeAttribute("uom","");
writer.writeAttribute("datum","");
writer.writeEmptyElement(WITSML_NS,"tvdDelta");
writer.writeAttribute("uom","");
writer.writeAttribute("datum","");
writer.writeEmptyElement(WITSML_NS,"modelToolError");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gravTotalUncert");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dipAngleUncert");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magTotalUncert");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS, "gravAccelCorUsed");
writer.writeEmptyElement(WITSML_NS, "magXAxialCorUsed");
writer.writeEmptyElement(WITSML_NS, "sagCorUsed");
writer.writeEmptyElement(WITSML_NS, "magDrlstrCorUsed");
writer.writeEmptyElement(WITSML_NS,"gravTotalFieldReference");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magTotalFieldReference");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magDipAngleReference");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS, "magModelUsed");
writer.writeEmptyElement(WITSML_NS, "magModelValid");
writer.writeEmptyElement(WITSML_NS, "geoModelUsed");
writer.writeEmptyElement(WITSML_NS, "statusTrajStation");
writer.writeStartElement(WITSML_NS,"rawData");
writer.writeEmptyElement(WITSML_NS,"gravAxialRaw");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gravTran1Raw");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gravTran2Raw");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magAxialRaw");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magTran1Raw");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magTran2Raw");
writer.writeAttribute("uom","");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS,"corUsed");
writer.writeEmptyElement(WITSML_NS,"gravAxialAccelCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gravTran1AccelCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gravTran2AccelCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magAxialDrlstrCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magTran1DrlstrCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magTran2DrlstrCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"sagIncCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"sagAziCor");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"stnMagDeclUsed");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"stnGridCorUsed");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"dirSensorOffset");
writer.writeAttribute("uom","");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS,"valid");
writer.writeEmptyElement(WITSML_NS,"magTotalFieldCalc");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"magDipAngleCalc");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"gravTotalFieldCalc");
writer.writeAttribute("uom","");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS,"matrixCov");
writer.writeEmptyElement(WITSML_NS,"varianceNN");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"varianceNE");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"varianceNVert");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"varianceEE");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"varianceEVert");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"varianceVertVert");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"biasN");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"biasE");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"biasVert");
writer.writeAttribute("uom","");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS,"location");
writer.writeAttribute("uid","");
writer.writeEmptyElement(WITSML_NS,"wellCRS");
writer.writeAttribute("uidRef", "");
writer.writeEmptyElement(WITSML_NS,"latitude");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"longitude");
writer.writeAttribute("uom","");
writer.writeEmptyElement(WITSML_NS,"original");
writer.writeEmptyElement(WITSML_NS,"description");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "sourceStation");
writer.writeEmptyElement(WITSML_NS,"stationReference");
writer.writeEmptyElement(WITSML_NS,"trajectoryParent");
writer.writeAttribute("uidRef", "");
writer.writeEmptyElement(WITSML_NS,"wellboreParent");
writer.writeAttribute("uidRef", "");
writer.writeEndElement();
writeCommonData(writer);
writer.writeEndElement();
}
if (header) {
writeCommonData(writer);
writer.writeEmptyElement(WITSML_NS, "customData");
}
writer.writeEndElement();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy