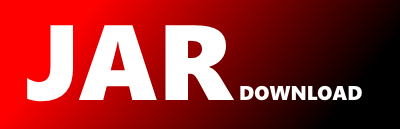
net.intelie.liverig.witsml.query.AbstractTubularQuery131 Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml.query;
import com.google.common.base.Strings;
import net.intelie.liverig.witsml.WitsmlFilters;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
abstract class AbstractTubularQuery131 extends AbstractQuery131 {
private final String uidWell;
private final String uidWellbore;
private final String uid;
private final WitsmlFilters filters;
private final boolean header;
private final boolean tubularComponent;
AbstractTubularQuery131(String uidWell, String uidWellbore, String uid, WitsmlFilters filters, boolean header, boolean tubularComponent) {
this.uidWell = uidWell;
this.uidWellbore = uidWellbore;
this.uid = uid;
this.filters = filters;
this.header = header;
this.tubularComponent = tubularComponent;
}
boolean hasTubularComponent() {
return tubularComponent;
}
@Override
public String type() {
return "tubular";
}
@Override
void query(XMLStreamWriter writer) throws XMLStreamException {
writeRootElement(writer, "tubulars");
writer.writeStartElement(WITSML_NS, "tubular");
writer.writeAttribute("uidWell", uidWell);
writer.writeAttribute("uidWellbore", uidWellbore);
writer.writeAttribute("uid", Strings.nullToEmpty(uid));
writer.writeEmptyElement(WITSML_NS, "nameWell");
writer.writeEmptyElement(WITSML_NS, "nameWellbore");
writer.writeEmptyElement(WITSML_NS, "name");
if (tubularComponent) {
writer.writeEmptyElement(WITSML_NS, "typeTubularAssy");
writer.writeEmptyElement(WITSML_NS, "valveFloat");
writer.writeEmptyElement(WITSML_NS, "sourceNuclear");
writer.writeEmptyElement(WITSML_NS, "diaHoleAssy");
writer.writeAttribute("uom", "");
writer.writeStartElement(WITSML_NS, "tubularComponent");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "typeTubularComp");
writer.writeEmptyElement(WITSML_NS, "sequence");
writer.writeEmptyElement(WITSML_NS, "description");
writer.writeEmptyElement(WITSML_NS, "id");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "od");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "odMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "len");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "lenJointAv");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "numJointStand");
writer.writeEmptyElement(WITSML_NS, "wtPerLen");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "grade");
writer.writeEmptyElement(WITSML_NS, "odDrift");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tensYield");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tqYield");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "stressFatig");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "lenFishneck");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "idFishneck");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "odFishneck");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "disp");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "presBurst");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "presCollapse");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "classService");
writer.writeEmptyElement(WITSML_NS, "wearWall");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "thickWall");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "configCon");
writer.writeEmptyElement(WITSML_NS, "bendStiffness");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "axialStiffness");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "torsionalStiffness");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "typeMaterial");
writer.writeEmptyElement(WITSML_NS, "doglegMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "vendor");
writer.writeEmptyElement(WITSML_NS, "model");
writer.writeStartElement(WITSML_NS, "nameTag");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "name");
writer.writeEmptyElement(WITSML_NS, "numberingScheme");
writer.writeEmptyElement(WITSML_NS, "technology");
writer.writeEmptyElement(WITSML_NS, "location");
writer.writeEmptyElement(WITSML_NS, "installationDate");
writer.writeEmptyElement(WITSML_NS, "installationCompany");
writer.writeEmptyElement(WITSML_NS, "mountingCode");
writer.writeEmptyElement(WITSML_NS, "comment");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "bitRecord");
writer.writeEmptyElement(WITSML_NS, "numBit");
writer.writeEmptyElement(WITSML_NS, "diaBit");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "diaPassThru");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "diaPilot");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "manufacturer");
writer.writeEmptyElement(WITSML_NS, "typeBit");
writer.writeEmptyElement(WITSML_NS, "cost");
writer.writeAttribute("currency", "");
writer.writeEmptyElement(WITSML_NS, "codeIADC");
writer.writeEmptyElement(WITSML_NS, "condInitInner");
writer.writeEmptyElement(WITSML_NS, "condInitOuter");
writer.writeEmptyElement(WITSML_NS, "condInitDull");
writer.writeEmptyElement(WITSML_NS, "condInitLocation");
writer.writeEmptyElement(WITSML_NS, "condInitBearing");
writer.writeEmptyElement(WITSML_NS, "condInitGauge");
writer.writeEmptyElement(WITSML_NS, "condInitOther");
writer.writeEmptyElement(WITSML_NS, "condInitReason");
writer.writeEmptyElement(WITSML_NS, "condFinalInner");
writer.writeEmptyElement(WITSML_NS, "condFinalOuter");
writer.writeEmptyElement(WITSML_NS, "condFinalDull");
writer.writeEmptyElement(WITSML_NS, "condFinalLocation");
writer.writeEmptyElement(WITSML_NS, "condFinalBearing");
writer.writeEmptyElement(WITSML_NS, "condFinalGauge");
writer.writeEmptyElement(WITSML_NS, "condFinalOther");
writer.writeEmptyElement(WITSML_NS, "condFinalReason");
writer.writeEmptyElement(WITSML_NS, "drive");
writer.writeEmptyElement(WITSML_NS, "bitClass");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "areaNozzleFlow");
writer.writeAttribute("uom", "");
writer.writeStartElement(WITSML_NS, "nozzle");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "index");
writer.writeEmptyElement(WITSML_NS, "diaNozzle");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "typeNozzle");
writer.writeEmptyElement(WITSML_NS, "len");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "orientation");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "connection");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "id");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "od");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "len");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "typeThread");
writer.writeEmptyElement(WITSML_NS, "sizeThread");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tensYield");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tqYield");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "position");
writer.writeEmptyElement(WITSML_NS, "criticalCrossSection");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "presLeak");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tqMakeup");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "jar");
writer.writeEmptyElement(WITSML_NS, "forUpSet");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "forDownSet");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "forUpTrip");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "forDownTrip");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "forPmpOpen");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "forSealFric");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "typeJar");
writer.writeEmptyElement(WITSML_NS, "jarAction");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "mwdTool");
writer.writeEmptyElement(WITSML_NS, "flowrateMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "flowrateMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "tempMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "idEquv");
writer.writeAttribute("uom", "");
writer.writeStartElement(WITSML_NS, "sensor");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "typeMeasurement");
writer.writeEmptyElement(WITSML_NS, "offsetBot");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "comments");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "motor");
writer.writeEmptyElement(WITSML_NS, "offsetTool");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "presLossFact");
writer.writeEmptyElement(WITSML_NS, "flowrateMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "flowrateMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "diaRotorNozzle");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "clearanceBearBox");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "lobesRotor");
writer.writeEmptyElement(WITSML_NS, "lobesStator");
writer.writeEmptyElement(WITSML_NS, "typeBearing");
writer.writeEmptyElement(WITSML_NS, "tempOpMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "rotorCatcher");
writer.writeEmptyElement(WITSML_NS, "dumpValve");
writer.writeEmptyElement(WITSML_NS, "diaNozzle");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "rotatable");
writer.writeEmptyElement(WITSML_NS, "bendSettingsMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "bendSettingsMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "stabilizer");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "lenBlade");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "lenBladeGauge");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "odBladeMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "odBladeMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "distBladeBot");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "shapeBlade");
writer.writeEmptyElement(WITSML_NS, "factFric");
writer.writeEmptyElement(WITSML_NS, "typeBlade");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "bend");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "angle");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "distBendBot");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "holeOpener");
writer.writeEmptyElement(WITSML_NS, "typeHoleOpener");
writer.writeEmptyElement(WITSML_NS, "numCutter");
writer.writeEmptyElement(WITSML_NS, "manufacturer");
writer.writeEmptyElement(WITSML_NS, "diaHoleOpener");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeStartElement(WITSML_NS, "rotarySteerableTool");
writer.writeEmptyElement(WITSML_NS, "deflectionMethod");
writer.writeEmptyElement(WITSML_NS, "bendAngle");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "bendOffset");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "holeSizeMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "holeSizeMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "wobMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "operatingSpeed");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "speedMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "flowRateMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "flowRateMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "downLinkFlowRateMn");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "downLinkFlowRateMx");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "pressLossFact");
writer.writeEmptyElement(WITSML_NS, "padCount");
writer.writeEmptyElement(WITSML_NS, "padLen");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "padWidth");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "padOffset");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "openPadOd");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "closePadOd");
writer.writeAttribute("uom", "");
writer.writeStartElement(WITSML_NS, "sensor");
writer.writeAttribute("uid", "");
writer.writeEmptyElement(WITSML_NS, "typeMeasurement");
writer.writeEmptyElement(WITSML_NS, "offsetBot");
writer.writeAttribute("uom", "");
writer.writeEmptyElement(WITSML_NS, "comments");
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
writer.writeEmptyElement(WITSML_NS, "customData");
writer.writeEndElement();
}
if (header) {
writeCommonData(writer);
writer.writeEmptyElement(WITSML_NS, "customData");
}
writer.writeEndElement();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy