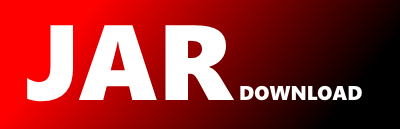
net.intelie.liverig.witsml.server.WITSMLServer Maven / Gradle / Ivy
The newest version!
package net.intelie.liverig.witsml.server;
import com.google.common.base.Joiner;
import com.google.common.base.Splitter;
import com.google.common.base.Strings;
import com.google.common.collect.Iterables;
import net.intelie.liverig.util.XMLOutputFactoryFactory;
import net.intelie.liverig.util.XMLStreamWriterAutoCloseable;
import net.intelie.liverig.witsml.StoreSoapPortInterface;
import net.intelie.liverig.witsml.WITSMLBaseMsg;
import net.intelie.liverig.witsml.WITSMLException;
import net.intelie.liverig.witsml.WITSMLResult;
import net.intelie.liverig.witsml.objects.ApiVers;
import net.intelie.liverig.witsml.objects.LogDateTimeIndex;
import net.intelie.liverig.witsml.objects.LogIndex;
import org.jetbrains.annotations.Nullable;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamWriter;
import java.io.StringWriter;
import java.util.*;
public class WITSMLServer implements StoreSoapPortInterface {
private static final Splitter OPTIONS_SPLITTER = Splitter.on(';').omitEmptyStrings().trimResults();
private static final Splitter SPLITTER = Splitter.on(',').trimResults();
private static final Joiner JOINER = Joiner.on(',');
private final Config config;
public WITSMLServer(Config config) {
this.config = config;
}
@Override
public String addToStore(String type, String xml, String options, String capabilitiesIn) throws WITSMLException {
throw new WITSMLException((short) -414);
}
@Override
public void deleteFromStore(String type, String query, String options, String capabilitiesIn) throws WITSMLException {
throw new WITSMLException((short) -414);
}
@Override
public String getBaseMsg(short result) {
return WITSMLBaseMsg.getBaseMsg(result);
}
@Override
public String getCap(String options) throws WITSMLException {
String dataVersion = option(options, "dataVersion");
ApiVers apiVers = apiVers(Strings.isNullOrEmpty(dataVersion) ? "1.3.1.1" : dataVersion);
if (apiVers == null) {
throw new WITSMLException((short) -423);
}
return config.getCap(apiVers).toString();
}
@Override
public WITSMLResult getFromStore(String type, String query, String options, String capabilitiesIn) throws WITSMLException {
if (Strings.isNullOrEmpty(type)) {
throw new WITSMLException((short) -407);
}
if (Strings.isNullOrEmpty(query)) {
throw new WITSMLException((short) -408);
}
QueryParser parser = new QueryParser();
parser.parse(query);
if (!(type + 's').equals(parser.collection())) {
throw new WITSMLException((short) -409);
}
ApiVers apiVers = apiVers(parser.version());
if (apiVers == null) {
throw new WITSMLException((short) -409);
}
String returnElements = option(options, "returnElements");
StringWriter sw = new StringWriter();
try (XMLStreamWriterAutoCloseable ac = XMLOutputFactoryFactory.createXMLStreamWriter(sw)) {
XMLStreamWriter writer = ac.get();
writer.setDefaultNamespace(apiVers.getSchemaNamespace());
writer.writeStartElement(apiVers.getSchemaNamespace(), parser.collection());
writer.writeDefaultNamespace(apiVers.getSchemaNamespace());
writer.writeAttribute("version", apiVers.getSchemaVersion());
for (QueryParser.Query entry : parser.queries()) {
getFromStore(writer, apiVers, entry, returnElements);
}
writer.writeEndDocument();
} catch (XMLStreamException e) {
throw new WITSMLException(WITSMLException.UNKNOWN_PROGRAM_ERROR, e);
}
return new WITSMLResult(sw.toString());
}
private void getFromStore(XMLStreamWriter writer, ApiVers apiVers, QueryParser.Query query,
@Nullable String returnElements) throws WITSMLException, XMLStreamException {
boolean id_only = apiVers == ApiVers.WITSML_141 && "id-only".equals(returnElements);
boolean header_only = apiVers == ApiVers.WITSML_141 && "header-only".equals(returnElements);
boolean data_only = apiVers == ApiVers.WITSML_141 && "data-only".equals(returnElements);
boolean all = "all".equals(returnElements);
boolean requested = !(id_only || header_only || data_only || all);
boolean header = !id_only && !data_only;
boolean data = data_only || all || (requested && query.getRequested().contains("logData"));
if ("well".equals(query.getObject())) {
for (Well well : getWells(query.getUid())) {
writer.writeStartElement(apiVers.getSchemaNamespace(), "well");
writer.writeAttribute("uid", Strings.nullToEmpty(well.getUid()));
writeSimpleElement(writer, apiVers.getSchemaNamespace(), "name", Strings.nullToEmpty(well.getName()));
writer.writeEndElement();
}
} else if ("wellbore".equals(query.getObject())) {
for (Wellbore wellbore : getWellbores(query.getUidWell(), query.getUid())) {
Well well = wellbore.getWell();
writer.writeStartElement(apiVers.getSchemaNamespace(), "wellbore");
writer.writeAttribute("uidWell", Strings.nullToEmpty(well.getUid()));
writer.writeAttribute("uid", Strings.nullToEmpty(wellbore.getUid()));
writeSimpleElement(writer, apiVers.getSchemaNamespace(), "nameWell", Strings.nullToEmpty(well.getName()));
writeSimpleElement(writer, apiVers.getSchemaNamespace(), "name", Strings.nullToEmpty(wellbore.getName()));
writer.writeEndElement();
}
} else if ("log".equals(query.getObject())) {
for (Log log : getLogs(query.getUidWell(), query.getUidWellbore(), query.getUid())) {
Wellbore wellbore = log.getWellbore();
Well well = wellbore.getWell();
writer.writeStartElement(apiVers.getSchemaNamespace(), "log");
writer.writeAttribute("uidWell", Strings.nullToEmpty(well.getUid()));
writer.writeAttribute("uidWellbore", Strings.nullToEmpty(wellbore.getUid()));
writer.writeAttribute("uid", Strings.nullToEmpty(log.getUid()));
writeSimpleElement(writer, apiVers.getSchemaNamespace(), "nameWell", Strings.nullToEmpty(well.getName()));
writeSimpleElement(writer, apiVers.getSchemaNamespace(), "nameWellbore", Strings.nullToEmpty(wellbore.getName()));
writeSimpleElement(writer, apiVers.getSchemaNamespace(), "name", Strings.nullToEmpty(log.getName()));
List mnemonics = new ArrayList<>(log.getCurves().keySet());
List units = null;
if (apiVers == ApiVers.WITSML_141) {
units = new ArrayList<>(mnemonics.size());
Map curves = log.getCurves();
for (String mnemonic : mnemonics) {
units.add(curves.get(mnemonic).getUnit());
}
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy