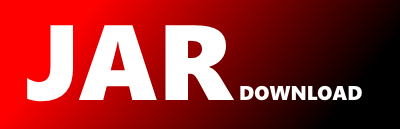
net.intelie.live.plugins.messenger.api.MessengerResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plugin-messenger Show documentation
Show all versions of plugin-messenger Show documentation
Intelie Live Messenger Plugin
The newest version!
package net.intelie.live.plugins.messenger.api;
import com.google.common.base.Strings;
import net.intelie.live.NeedsAuthority;
import net.intelie.live.QueryResponse;
import net.intelie.live.UseProxy;
import net.intelie.live.plugins.messenger.MessengerPermission;
import net.intelie.live.plugins.messenger.data.RoomData;
import net.intelie.live.plugins.messenger.data.UserData;
import net.intelie.live.plugins.messenger.data.UserMessage;
import net.intelie.live.plugins.messenger.data.UserRoomData;
import net.intelie.live.plugins.messenger.search.SearchService;
import net.intelie.live.util.WebUtils;
import org.jetbrains.annotations.NotNull;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.transaction.annotation.Transactional;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.*;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.Response;
import java.util.*;
import java.util.stream.Collectors;
import static net.intelie.live.Permission.ADMIN;
import static net.intelie.live.plugins.messenger.chat.MessengerConstants.ROOM_NAME_REQUIRED;
@UseProxy
@Path("/")
@Consumes("application/json")
@Produces("application/json")
public class MessengerResource {
private static final Logger LOGGER = LoggerFactory.getLogger(MessengerResource.class);
private final MessengerServiceImpl messengerService;
private final SearchService searchService;
public MessengerResource() {
this(null, null);
}
public MessengerResource(MessengerServiceImpl messengerService, SearchService searchService) {
this.messengerService = messengerService;
this.searchService = searchService;
}
@POST
@Path("room/{roomId}/message")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public UserMessage say(@PathParam("roomId") String roomId, UserMessage message) throws Exception {
if (!messengerService.hasPermission(roomId))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
return messengerService.sendMessage(roomId, message.getMessage());
}
@POST
@Path("room/{roomId}/message/{id}")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public UserMessage updateMessage(@PathParam("roomId") String roomId, @PathParam("id") String messageId, UserMessage message) throws Exception {
if (!messengerService.hasPermission(roomId))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
return messengerService.updateMessage(message);
}
@DELETE
@Path("room/{roomId}/message/{id}")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public UserMessage deleteMessageById(@PathParam("roomId") String roomId, @PathParam("id") String messageId) throws Exception {
if (!messengerService.hasPermission(roomId))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
return messengerService.deleteMessage(roomId, messageId);
}
@POST
@Transactional
@Path("room")
@NeedsAuthority(MessengerPermission.CREATE_ROOMS)
public RoomData createRoom(RoomData room) throws Exception {
if (Strings.isNullOrEmpty(room.getName()))
throw new Exception(ROOM_NAME_REQUIRED);
return messengerService.createRoom(room.getName());
}
@DELETE
@Transactional
@Path("room/{id}")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void deleteRoom(@PathParam("id") String id) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.deleteRoom(id);
}
@POST
@Transactional
@Path("room/{id}/name")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public RoomData renameRoom(@PathParam("id") String id, String newName) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
return messengerService.renameRoom(id, newName);
}
@POST
@Transactional
@Path("room/{id}/archive")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void archiveRoom(@PathParam("id") String id) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.archiveRoom(id);
}
@DELETE
@Transactional
@Path("room/{id}/archive")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void restoreRoom(@PathParam("id") String id) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.restoreRoom(id);
}
@POST
@Transactional
@Path("room/{id}/leave")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void leaveRoom(@PathParam("id") String id) throws Exception {
messengerService.leaveRoom(id);
}
@POST
@Transactional
@Path("room/{id}/mute")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void muteRoom(@PathParam("id") String id) throws Exception {
messengerService.setRoomIsMuted(id, true);
}
@DELETE
@Transactional
@Path("room/{id}/mute")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void unMuteRoom(@PathParam("id") String id) throws Exception {
messengerService.setRoomIsMuted(id, false);
}
@POST
@Transactional
@Path("room/{id}/favorite")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void favoriteRoom(@PathParam("id") String id) throws Exception {
messengerService.setRoomIsFavorite(id, true);
}
@DELETE
@Transactional
@Path("room/{id}/favorite")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void unFavoriteRoom(@PathParam("id") String id) throws Exception {
messengerService.setRoomIsFavorite(id, false);
}
@POST
@Transactional
@Path("room/{id}/user/{userId}")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void addUser(@PathParam("id") String id, @PathParam("userId") Integer userId) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.addUser(id, userId);
}
@DELETE
@Path("room/{id}/user/{userId}")
@Transactional
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void removeUser(@PathParam("id") String id, @PathParam("userId") Integer userId) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.removeUser(id, userId);
}
@POST
@Transactional
@Path("room/{id}/users")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void updateUsersAdmins(@PathParam("id") String id, UsersUpdatePackage updatePackage) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.updateRoomUsers(id, updatePackage.usersToAddOrUpdate(), updatePackage.usersToRemove());
}
@POST
@Transactional
@Path("room/{id}/admin/{userId}")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void makeRoomAdmin(@PathParam("id") String id, @PathParam("userId") Integer userId) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.makeRoomAdmin(id, userId);
}
@DELETE
@Path("room/{id}/admin/{userId}")
@Transactional
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public void removeRoomAdmin(@PathParam("id") String id, @PathParam("userId") Integer userId) throws Exception {
if (!messengerService.isRoomAdmin(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
messengerService.removeRoomAdmin(id, userId);
}
@GET
@Transactional
@Path("rooms")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public List listRooms() {
return messengerService.listRooms();
}
@GET
@Transactional(readOnly = true)
@Path("users/online")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public List onlineUsers() {
return messengerService.onlineUsers();
}
@POST
@Path("room/{id}/feed")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public List feed(@PathParam("id") String id, @Context HttpServletRequest context) throws Exception {
if (!messengerService.hasPermission(id))
throw new WebApplicationException(Response.Status.UNAUTHORIZED);
return messengerService.feed(id, WebUtils.makeHost(context));
}
@POST
@Path("feed")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public List feed(@Context HttpServletRequest context) throws Exception {
return messengerService.feed(WebUtils.makeHost(context));
}
@GET
@Path("room/{id}/feed/sync")
@NeedsAuthority({MessengerPermission.USE_MESSENGER, MessengerPermission.CREATE_ROOMS})
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy