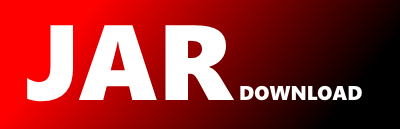
net.intelie.live.plugins.messenger.chat.RoomState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plugin-messenger Show documentation
Show all versions of plugin-messenger Show documentation
Intelie Live Messenger Plugin
The newest version!
package net.intelie.live.plugins.messenger.chat;
import net.intelie.live.plugins.feed.api.FeedDef.FeedItem;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
public class RoomState {
private String name;
private String type;
private Integer authorId;
private Long createdAt;
private Set users = new LinkedHashSet<>();
private Set feedItems;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAuthorId() {
return this.authorId;
}
public void setAuthorId(Integer authorId) {
this.authorId = authorId;
}
public Long getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Long createdAt) {
this.createdAt = createdAt;
}
public boolean addUser(Integer userId) {
if (this.users.stream().noneMatch(user -> userId.equals(user.getId()))) {
return this.users.add(new UserState(userId));
}
return false;
}
public boolean removeUser(Integer userId) {
return this.users.removeAll(this.users.stream().filter(user -> userId.equals(user.getId())).collect(Collectors.toList()));
}
public boolean makeAdmin(Integer userId, boolean opt) {
if (this.users.stream().anyMatch(user -> userId.equals(user.getId()))) {
for (UserState user : this.users) {
if (userId.equals(user.getId())) {
user.setAdmin(opt);
return true;
}
}
}
return false;
}
public boolean isUser(Integer userId) {
return this.users.stream().anyMatch(user -> userId.equals(user.getId()));
}
public boolean isAdmin(Integer userId) {
return this.users.stream().anyMatch(user -> userId.equals(user.getId()) && user.isAdmin());
}
public boolean isUniqueAdmin(Integer userId) {
List admins = this.users.stream().filter(UserState::isAdmin).collect(Collectors.toList());
return admins.size() == 1 && admins.stream().anyMatch(user -> userId.equals(user.getId()));
}
public boolean hasAdmin() {
return users.stream().anyMatch(UserState::isAdmin);
}
public Set getUsers() {
return this.users;
}
public Set getFeedItems() {
return feedItems;
}
public RoomState deepClone(){
RoomState state = new RoomState();
state.name = name;
state.type = type;
state.authorId = authorId;
state.createdAt = createdAt;
state.users = users.stream().map(UserState::deepClone).collect(Collectors.toSet());
return state;
}
public static class RoomFeedItem {
private String plugin;
private String type;
private String timestamp;
private String filter;
public String getPlugin() {
return plugin;
}
public void setPlugin(String plugin) {
this.plugin = plugin;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getTimestamp() {
return timestamp;
}
public void setTimestamp(String timestamp) {
this.timestamp = timestamp;
}
public String getFilter() {
return filter;
}
public void setFilter(String filter) {
this.filter = filter;
}
public FeedItem toFeedItem() {
FeedItem feedItem = new FeedItem();
feedItem.setType(getType());
feedItem.setTimestamp(getTimestamp());
feedItem.setFilter(getFilter());
return feedItem;
}
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy