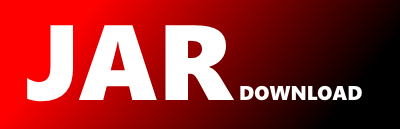
net.intelie.live.plugins.messenger.search.SearchableEventBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plugin-messenger Show documentation
Show all versions of plugin-messenger Show documentation
Intelie Live Messenger Plugin
The newest version!
package net.intelie.live.plugins.messenger.search;
import net.intelie.live.*;
import net.intelie.live.plugins.messenger.search.document.*;
import net.intelie.pipes.types.Type;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.LongPoint;
import org.apache.lucene.document.StringField;
import org.apache.lucene.document.TextField;
import org.apache.lucene.index.Term;
import org.jetbrains.annotations.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicLong;
import static net.intelie.live.plugins.messenger.search.SearchableFields.UID;
class SearchableEventBase implements AutoCloseable {
private static final Logger LOGGER = LoggerFactory.getLogger(SearchableEventBase.class);
private final Live live;
private final SettingsNode lastIndexedNodeInfo;
private volatile AtomicLong lastIndexedTimestamp = new AtomicLong();
private final AtomicLong nIndexedEvents = new AtomicLong();
private Live.Action runningQueryAction;
private final Live.Action providerListenerAction;
private final SearchableIndexWriter indexWriter;
private final SearchableEventHandler searchableHandler;
private final LoggedUser loggedUser;
SearchableEventBase(Live live, SearchableIndexWriter indexWriter, SearchableEventHandler searchableHandler) throws Exception {
this.live = live;
this.indexWriter = indexWriter;
this.searchableHandler = searchableHandler;
this.loggedUser = live.auth().getLoggedUser();
SettingsNode settings = live.settings().home().cd("search");
lastIndexedNodeInfo = settings.cd("lastIndexedTimestamp").cd(searchableHandler.searchableName());
String actionDescription = "Provider Listener for " + searchableHandler.searchableName() + " searchable";
providerListenerAction = this.live.describeAction(actionDescription,
this.live.engine().getMainStorage().onProvidersChange(new ProviderListenerBase()));
}
String searchableName() {
return searchableHandler.searchableName();
}
Set searchableFields(){
return searchableHandler.searchableFields();
}
@Override
public void close() throws Exception {
try {
providerListenerAction.close();
} finally {
stopIndexing();
}
}
synchronized void restartIndexing() throws Exception {
stopIndexing();
lastIndexedTimestamp = new AtomicLong();
nIndexedEvents.set(0L);
lastIndexedNodeInfo.set(0L);
startIndexing();
}
synchronized void stopIndexing() throws Exception{
if (this.runningQueryAction != null) {
this.runningQueryAction.close();
}
}
synchronized void startIndexing() throws Exception {
Long lastTimestamp = getLastIndexedTimestamp();
Query query = searchableHandler.createQuery(lastTimestamp);
runningQueryAction = live.engine().runQueries(query.listenWith(new QueryListener.Empty() {
@Override
public void onEvent(QueryEvent event, boolean history) throws Exception {
for (Map evt : event)
processEventToSearchable(evt);
}
}));
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy