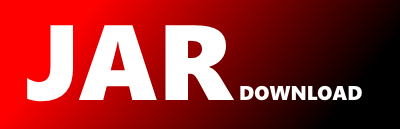
iot.jcypher.query.values.JcString Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jcypher Show documentation
Show all versions of jcypher Show documentation
Provides seamlessly integrated Java access to graph databases (Neo4J)
at different levels of abstraction.
/************************************************************************
* Copyright (c) 2014-2015 IoT-Solutions e.U.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
************************************************************************/
package iot.jcypher.query.values;
import iot.jcypher.domainquery.internal.QueryRecorder;
import iot.jcypher.query.values.functions.FUNCTION;
import iot.jcypher.query.values.operators.OPERATOR;
public class JcString extends JcPrimitive {
JcString() {
super();
}
/**
* JCYPHER
* create a string which is identified by a name
*
*/
public JcString(String name) {
this(name, null, null, null);
}
/**
* JCYPHER
* create a string which is identified by a name and initialized with a value
*
*/
public JcString(String name, String value) {
this(name, value, null, null);
}
JcString(Object val, ValueElement predecessor, IOperatorOrFunction opf) {
this(null, val, predecessor, opf);
}
JcString(String name, Object val, ValueElement predecessor, IOperatorOrFunction opf) {
super(name, val, predecessor, opf);
}
/**
* JCYPHER
* return the result of concatenating two strings, return a JcString
*
*/
public JcString concat(String concat) {
JcString ret = new JcString(concat, this, OPERATOR.String.CONCAT);
QueryRecorder.recordInvocationConditional(this, "concat", ret, QueryRecorder.literal(concat));
return ret;
}
/**
* JCYPHER
* return the result of concatenating two strings, return a JcString
*
*/
public JcString concat(JcString concat) {
JcString ret = new JcString(concat, this, OPERATOR.String.CONCAT);
QueryRecorder.recordInvocationConditional(this, "concat", ret, QueryRecorder.placeHolder(concat));
return ret;
}
/**
* JCYPHER
* return the result of removing leading and trailing white spaces form a string, return a JcString
*
*/
public JcString trim() {
JcString ret = new JcString(null, this,
new FunctionInstance(FUNCTION.String.TRIM, 1));
QueryRecorder.recordInvocationConditional(this, "trim", ret);
return ret;
}
/**
* JCYPHER
* return the result of removing leading white spaces form a string, return a JcString
*
*/
public JcString trimLeft() {
JcString ret = new JcString(null, this,
new FunctionInstance(FUNCTION.String.LTRIM, 1));
QueryRecorder.recordInvocationConditional(this, "trimLeft", ret);
return ret;
}
/**
* JCYPHER
* return the result of removing trailing white spaces form a string, return a JcString
*
*/
public JcString trimRight() {
JcString ret = new JcString(null, this,
new FunctionInstance(FUNCTION.String.RTRIM, 1));
QueryRecorder.recordInvocationConditional(this, "trimRight", ret);
return ret;
}
/**
* JCYPHER
* return the length of a string, return a JcNumber
*
*/
public JcNumber length() {
JcNumber ret = new JcNumber(null, this,
new FunctionInstance(FUNCTION.Collection.LENGTH, 1));
QueryRecorder.recordInvocationConditional(this, "length", ret);
return ret;
}
/**
* JCYPHER
* specify the part of a string which should be replaced
*
*/
public ReplaceWith replace(String what) {
ReplaceWith ret = new ReplaceWith(what, this, OPERATOR.Common.COMMA_SEPARATOR);
QueryRecorder.recordInvocationConditional(this, "replace", ret, QueryRecorder.literal(what));
return ret;
}
/**
* JCYPHER
* specify the part of a string which should be replaced
*
*/
public ReplaceWith replace(JcString what) {
ReplaceWith ret = new ReplaceWith(what, this, OPERATOR.Common.COMMA_SEPARATOR);
QueryRecorder.recordInvocationConditional(this, "replace", ret, QueryRecorder.placeHolder(what));
return ret;
}
/**
* JCYPHER
* specify the start offset of a substring to be extracted
*
*/
public SubString substring(int start) {
JcNumber sub = new JcNumber(start, this, OPERATOR.Common.COMMA_SEPARATOR);
SubString ret = new SubString(null, sub,
new FunctionInstance(FUNCTION.String.SUBSTRING, 2));
QueryRecorder.recordInvocationConditional(this, "substring", ret, QueryRecorder.literal(start));
return ret;
}
/**
* JCYPHER
* return a string containing the left n characters of the original string
*
*/
public JcString left(int n) {
JcNumber left = new JcNumber(n, this, OPERATOR.Common.COMMA_SEPARATOR);
SubString ret = new SubString(null, left,
new FunctionInstance(FUNCTION.String.LEFT, 2));
QueryRecorder.recordInvocationConditional(this, "left", ret, QueryRecorder.literal(n));
return ret;
}
/**
* JCYPHER
* return a string containing the right n characters of the original string
*
*/
public JcString right(int n) {
JcNumber right = new JcNumber(n, this, OPERATOR.Common.COMMA_SEPARATOR);
SubString ret = new SubString(null, right,
new FunctionInstance(FUNCTION.String.RIGHT, 2));
QueryRecorder.recordInvocationConditional(this, "right", ret, QueryRecorder.literal(n));
return ret;
}
/**
* JCYPHER
* return the original string in lowercase
*
*/
public JcString lower() {
JcString ret = new JcString(null, this,
new FunctionInstance(FUNCTION.String.LOWER, 1));
QueryRecorder.recordInvocationConditional(this, "lower", ret);
return ret;
}
/**
* JCYPHER
* return the original string in uppercase
*
*/
public JcString upper() {
JcString ret = new JcString(null, this,
new FunctionInstance(FUNCTION.String.UPPER, 1));
QueryRecorder.recordInvocationConditional(this, "upper", ret);
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy