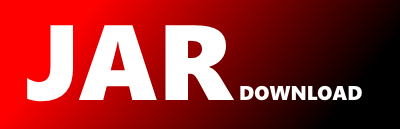
net.isger.brick.plugin.persist.Persists Maven / Gradle / Ivy
The newest version!
package net.isger.brick.plugin.persist;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import net.isger.util.Helpers;
import net.isger.util.Strings;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public final class Persists {
private static final Logger LOG;
private Map persists;
static {
LOG = LoggerFactory.getLogger(Persists.class);
}
public Persists() {
this(null);
}
@SuppressWarnings("unchecked")
public Persists(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy